Write a Java TCP socket program consisting of one client C and one local server S (localhost). Code for the client and server should be separate and contained in the respective files F1 and F2 above. Modify and use the code in the files posted on Blackboard: TCPWebClient18.java and TCPKRClient.java for the client C and TCPKRServer.java for the server S. Since we are testing behavior with no threads, the code should not have threads. Do not use System.exit() in your code. Submit your socket code in the files F1 and F2 (named as above). All the code needed should be in these two files. Include a comment at the top of each file that says how you compiled and ran the code. Capture screenshots with client and server screens during an example run and put them in the file F3. The client C asks the user to enter a web server W’s name as a string www.name.suf (for example, www.ieee.org) prints the message “CLIENT START=” followed by C’s local system time CT1 makes a TCP socket connection to the local server S listening on port 11211 sends W’s name to S and waits to receive W’s page from S receives and displays the text of W’s page sent to it by S prints the message “CLIENT END=” followed by C’s local system time CT2 prints the message “CLIENT DELAY=” followed by the value of CT=CT2-CT1 in milliseconds prints the message “ROUNDTRIP TIME=” followed by the value of RT=CT-ST in milliseconds, where ST is the value of server delay sent to it by S. The local server S (localhost) listens for and accepts the connection from C on port 11211 receives and prints the message “WEB SERVER=” followed by W’s name sent by C prints the message “IP ADDRESS=” followed by W’s IP address prints the message “SERVER START=” followed by S’s local system time ST1 uses the class HttpURLConnection to connect to the web server W on port 443 receives W’s page from the web server W prints the message “SERVER END=” followed by S’s local system time ST2 prints the message “SERVER DELAY=” followed by the value of ST=ST2-ST1 in milliseconds sends to C the page received from the web server W sends to C the value of ST Run Wireshark (WS) and use the Firefox web browser to make a request to https://www.ieee.org. Stop the WS capture and save it in a .pcap file named F4 that WS itself can open. Find the frames in the WS capture that are needed to answer the questions below (click on parts of packets in the frames to get more information if needed). Submit a file F5 (named as above) that has all your answers. 2.1 Of the WS frames corresponding to the packets sent by the web server at http://www.ieee.org, choose any (ONE) WS frame that WS has labeled with Protocol=TCP that has Length=1514. 2.1.1 Indicate the WS frame number, and the source IP address and destination port number in this frame. Explain the significance of this specific IP address and this specific port number. 2.1.2 Indicate in order each protocol that appears in this frame and indicate the size of each of these protocol headers. 2.1.3 What is the value of the Total Length field in the IP header and what is the value of the size of the TCP segment data given by WS? Explain carefully the relation between these two values. 2.1.4 Using the actual hex values of the relevant bytes in this frame, explain the relation between the Sequence Number and Sequence Number (raw) in the TCP header.
- Write a Java TCP socket
program consisting of one client C and one local server S (localhost). Code for the client and server should be separate and contained in the respective files F1 and F2 above. Modify and use the code in the files posted on Blackboard: TCPWebClient18.java and TCPKRClient.java for the client C and TCPKRServer.java for the server S. Since we are testing behavior with no threads, the code should not have threads.
Do not use System.exit() in your code. Submit your socket code in the files F1 and F2 (named as above). All the code needed should be in these two files. Include a comment at the top of each file that says how you compiled and ran the code. Capture screenshots with client and server screens during an example run and put them in the file F3.
The client C
- asks the user to enter a web server W’s name as a string www.name.suf (for example, www.ieee.org)
- prints the message “CLIENT START=” followed by C’s local system time CT1
- makes a TCP socket connection to the local server S listening on port 11211
- sends W’s name to S and waits to receive W’s page from S
- receives and displays the text of W’s page sent to it by S
- prints the message “CLIENT END=” followed by C’s local system time CT2
- prints the message “CLIENT DELAY=” followed by the value of CT=CT2-CT1 in milliseconds
- prints the message “ROUNDTRIP TIME=” followed by the value of RT=CT-ST in milliseconds, where ST is the value of server delay sent to it by S.
The local server S (localhost)
- listens for and accepts the connection from C on port 11211
- receives and prints the message “WEB SERVER=” followed by W’s name sent by C
- prints the message “IP ADDRESS=” followed by W’s IP address
- prints the message “SERVER START=” followed by S’s local system time ST1
- uses the class HttpURLConnection to connect to the web server W on port 443
- receives W’s page from the web server W
- prints the message “SERVER END=” followed by S’s local system time ST2
- prints the message “SERVER DELAY=” followed by the value of ST=ST2-ST1 in milliseconds
- sends to C the page received from the web server W
- sends to C the value of ST
- Run Wireshark (WS) and use the Firefox web browser to make a request to https://www.ieee.org. Stop the WS capture and save it in a .pcap file named F4 that WS itself can open. Find the frames in the WS capture that are needed to answer the questions below (click on parts of packets in the frames to get more information if needed). Submit a file F5 (named as above) that has all your answers.
2.1 Of the WS frames corresponding to the packets sent by the web server at http://www.ieee.org, choose any (ONE) WS frame that WS has labeled with Protocol=TCP that has Length=1514.
2.1.1 Indicate the WS frame number, and the source IP address and destination port number in this frame. Explain the significance of this specific IP address and this specific port number.
2.1.2 Indicate in order each protocol that appears in this frame and indicate the size of each of these protocol headers.
2.1.3 What is the value of the Total Length field in the IP header and what is the value of the size of the TCP segment data given by WS? Explain carefully the relation between these two values.
2.1.4 Using the actual hex values of the relevant bytes in this frame, explain the relation between the Sequence Number and Sequence Number (raw) in the TCP header.

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

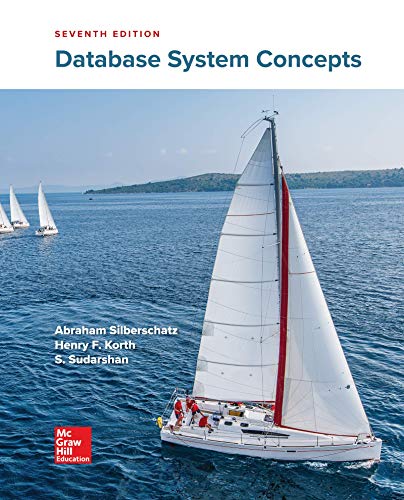
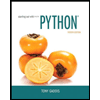
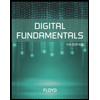
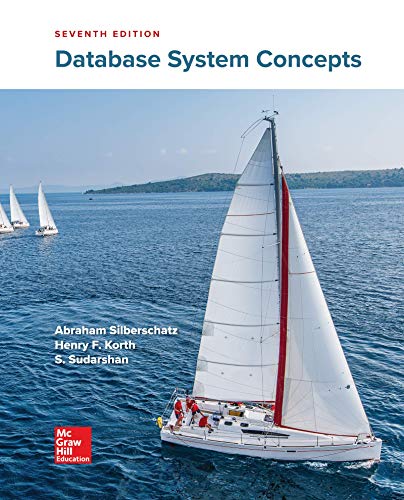
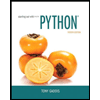
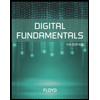
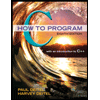
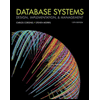
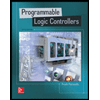