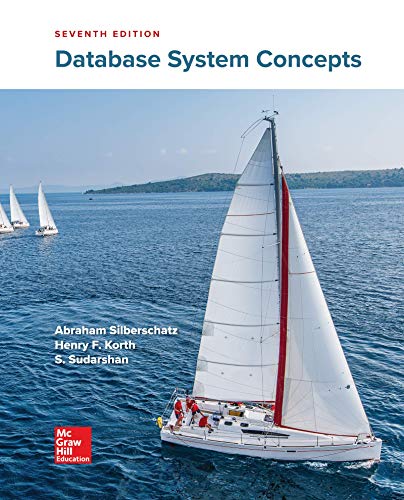
initial c++ file/starter code:
#include <
#include <iostream>
#include <algorithm>
using namespace std;
// The puzzle will always have exactly 20 columns
const int numCols = 20;
// Searches the entire puzzle, but may use helper functions to implement logic
void searchPuzzle(const char puzzle[][numCols], const string wordBank[],
vector <string> &discovered, int numRows, int numWords);
// Printer function that outputs a vector
void printVector(const vector <string> &v);
// Example of one potential helper function.
// bool searchPuzzleToTheRight(const char puzzle[][numCols], const string &word,
// int rowStart, int colStart)
int main()
{
int numRows, numWords;
// grab the array row dimension and amount of words
cin >> numRows >> numWords;
// declare a 2D array
char puzzle[numRows][numCols];
// TODO: fill the 2D array via input
// read the puzzle in from the input file using cin
// create a 1D array for wods
string wordBank[numWords];
// TODO: fill the wordBank through input using cin
// set up discovery vector
vector <string> discovered;
// Search for Words
searchPuzzle(puzzle, wordBank, discovered, numRows, numWords);
// Sort the results
sort(discovered.begin(), discovered.end());
// Print vector of discovered words
printVector(discovered);
return 0;
}
// TODO: implement searchPuzzle and any helper functions you want
void searchPuzzle(const char puzzle[][numCols], const string wordBank[],
vector <string> &discovered, int numRows, int numWords)
{
}
// TODO: implement printVector
void printVector(const vector <string> &v)
{
}
example input file:
3
2
H E L L O J K L I Y Q S R P Z I M K O P
W R L D Q J K L I Y Q S R P Z I M K O P
B Y Z A N T I N E Q W E R T Y U I O P Z
HELLO
WORLD
star wars file:
25
15
L P O A G Y R K T A T O O I N E D O X L
J G G T J U V R E B A S T H G I L J Y N
F P A X B E L L D N Y U E Z B I E S N H
L E I A H K S U S S A M C V B H N T W S
U I Z U L F F K D O T E J O Z F M D T Z
B M G L G Z E E H R N I N C W X X O T R
I S N T X T K S Z T O E R E H U R D I I
M V P N B W A H L A K I W K X M R C F J
P Z H K L Z J T G T P N D P T P V X Y H
U N W W J Y J R L W U I O R R Y O X P P
G A A I L I B A D V S Y O I U Q H O D F
M O D W Z Z Q D K N Q O N A N F P T H R
D V Z G Y W Z Y Q P P C H G V M T J P P
L Q H V E Z V T G E E M O O F S L G Y L
C Z L U P S A M R S G V X V M I L T F R
U A K B O Z R T S T C Z T P D T A X K A
U A W A V V X I E I D L C Y O O B C N N
S Y I W X C N D V N C H H C D R J A Y A
G J E D I I E Q N S S N G H O I E W I K
G F E G H R J E G L L P Q O Y Q O D E I
A V V N N P D T W R D V C M A G S J A N
A D N I Q Y Y G O W Y E D Y Z A O O P V
R J O K N J M Z L V T U V W H F O F D V
V C T Z H U O B I W A N Z H I P Y B Z M
L M X Y N I T G C Q E L V U Q B P L D L
AMIDALA ANAKIN DARTH
DROID JEDI KENOBI
LEIA LIGHTSABER LUKE
OBIWAN PRINCESS STORMTROOPER
TATOOINE TWILEK VADER
toy story file:
17
38
S T I N K Y P E T E Y O C K D Y N E Y C
B B Y A J S S D R Y M B B H S Q Y R N F
W J S D L J U F P A L O O T U E V R N U
K T B I O C P H Z D O L R P S C E T E E
E I N H K O R F J K H E O L E X K P L R
B K A Y D Z W A W U T S L M B E T L E K
Y Q C D B K Z O E C U U H A E F P T E R
B O Y D N A R U H Y B A R E A S S D H S
T I J A A M E O B N T B I L R U H U T P
Q L G V S I P T P L I H I Y B I B H Q U
E E I B X A B A M E H E G S S Z F D D C
H M I I A I R T H T N T H I G U E F O R
Q D R S X B I O E S L O S A L R A F L E
I T J M S H Y P G Q O C N D B G E O L T
R P N I U E W W R F T N H A M M E Y Y T
E E M I L Y J J A N S H N E K E N K M U
G Y Z E E H W M S A O F H C T I W T P B
ALIENS
ANDY
BARBIE
BIGBABY
BOOKWORM
BOPEEP
BULLSEYE
BUSTER
BUTTERCUP
BUZZ
CHUCKLES
DAISY
DAPHNE
DOLLY
DUCKY
EMILY
GERI
HAMM
JESSIE
KEN
LENNY
LIGHTYEAR
LOTSO
MOLLY
POTATO
REX
RIP
SARGE
SHERIFF
SLINKY
STINKYPETE
STRETCH
TRIXIE
TWITCH
WARDOG
WHEEZY
WOODY
ZURG
c++ code
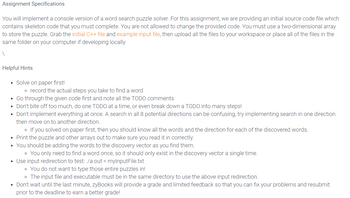

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

- #include <iostream>#include <cstdlib>#include <time.h>#include <chrono> using namespace std::chrono;using namespace std; void randomVector(int vector[], int size){ for (int i = 0; i < size; i++) { //ToDo: Add Comment vector[i] = rand() % 100; }} int main(){ unsigned long size = 100000000; srand(time(0)); int *v1, *v2, *v3; //ToDo: Add Comment auto start = high_resolution_clock::now(); //ToDo: Add Comment v1 = (int *) malloc(size * sizeof(int *)); v2 = (int *) malloc(size * sizeof(int *)); v3 = (int *) malloc(size * sizeof(int *)); randomVector(v1, size); randomVector(v2, size); //ToDo: Add Comment for (int i = 0; i < size; i++) { v3[i] = v1[i] + v2[i]; } auto stop = high_resolution_clock::now(); //ToDo: Add Comment auto duration = duration_cast<microseconds>(stop - start); cout << "Time taken by function: " << duration.count()…arrow_forwardIn C++ commas must be there. main.cpp #include <vector>#include <string>#include <iostream> using namespace std; // TODO: Write method to create and output all permutations of the list of names.void PrintAllPermutations(const vector<string> &permList, const vector<string> &nameList) { } int main() { vector<string> nameList; vector<string> permList; string name; // TODO: Read in a list of names; stop when -1 is read. Then call recursive method. return 0;}arrow_forward// MichiganCities.cpp - This program prints a message for invalid cities in Michigan. // Input: Interactive // Output: Error message or nothing #include <iostream> #include <string> using namespace std; int main() { // Declare variables string inCity; // name of city to look up in array const int NUM_CITIES = 10; // Initialized array of cities string citiesInMichigan[] = {"Acme", "Albion", "Detroit", "Watervliet", "Coloma", "Saginaw", "Richland", "Glenn", "Midland", "Brooklyn"}; bool foundIt = false; // Flag variable int x; // Loop control variable // Get user input cout << "Enter name of city: "; cin >> inCity; // Write your loop here // Write your test statement here to see if there is // a match. Set the flag to true if city is found. // Test to see if city was not found to determine if // "Not a city in Michigan" message should be printed.…arrow_forward
- Assign pizzasInStore's first element's numCalories with the value in pizzasInStore's second element's numCalories. #include <stdio.h>#include <string.h> typedef struct Pizza_struct { char pizzaName[75]; int numCalories;} Pizza; int main(void) { Pizza pizzasInStore[2]; scanf("%s", pizzasInStore[0].pizzaName); scanf("%d", &pizzasInStore[0].numCalories); scanf("%s", pizzasInStore[1].pizzaName); scanf("%d", &pizzasInStore[1].numCalories); /* Your code goes here */ printf("A %s slice contains %d calories.\n", pizzasInStore[0].pizzaName, pizzasInStore[0].numCalories); printf("A %s slice contains %d calories.\n", pizzasInStore[1].pizzaName, pizzasInStore[1].numCalories); return 0;} I tried 10 time but I cant solve it so please help mearrow_forwardstatic List<String> findWinners(String pattern, int minLength, boolean even, Stream<String> stream) This function will receive a stream of strings and it will return a sorted list of strings that meet all of the following criteria: They must contain the pattern The strings length must be equal to or greater than the min length number The strings length must be an even or odd number This Function must take no longer 10000 milliseconds to return. pattern - A pattern that all the strings in the list returned must containminLength - The length that all the strings in the list returned must be equal to or greater thaneven - True if even false if odd. Denotes a condition that all the strings in the list returned must have a length that is even or odd.stream - A stream of strings to be filteredreturn - A list of strings that meets the criteria outlined above sorted by their length from small to largearrow_forwardmain.cc file #include <iostream>#include <memory> #include "customer.h" int main() { // Creates a line of customers with Adele at the front. // LinkedList diagram: // Adele -> Kehlani -> Giveon -> Drake -> Ruel std::shared_ptr<Customer> ruel = std::make_shared<Customer>("Ruel", 5, nullptr); std::shared_ptr<Customer> drake = std::make_shared<Customer>("Drake", 8, ruel); std::shared_ptr<Customer> giveon = std::make_shared<Customer>("Giveon", 2, drake); std::shared_ptr<Customer> kehlani = std::make_shared<Customer>("Kehlani", 15, giveon); std::shared_ptr<Customer> adele = std::make_shared<Customer>("Adele", 4, kehlani); std::cout << "Total customers waiting: "; // =================== YOUR CODE HERE =================== // 1. Print out the total number of customers waiting // in line by invoking TotalCustomersInLine. //…arrow_forward
- struct remove_from_front_of_vector { // Function takes no parameters, removes the book at the front of a vector, // and returns nothing. void operator()(const Book& unused) { (/// TO-DO (12) ||||| // // // Write the lines of code to remove the book at the front of "my_vector". // // Remember, attempting to remove an element from an empty data structure is // a logic error. Include code to avoid that. //// END-TO-DO (12) /||, } std::vector& my_vector; };arrow_forwardQ# Describe what a data structure is for a char**? (e.g. char** argv) Group of answer choices A. It is a pointer to a single c-string. B. It is an array of c-strings C. It is the syntax for dereferencing a c-string and returning the value.arrow_forward/* yahtC */ #include <stdio.h> #include <string.h> #include <stdlib.h> #include <time.h> void seed(int argc, char ** argv){ srand(time(NULL)); // Initialize random seed if (argc>1){ int i; if (1 == sscanf(argv[1],"%d",&i)){ srand(i); } } } void instructions(){ printf("\n\n\n\n" "\t**********************************************************************\n" "\t* Welcome to YahtC *\n" "\t**********************************************************************\n" "\tYahtC is a dice game (very) loosely modeled on Yahtzee\n" "\tBUT YahtC is not Yahtzee.\n\n" "\tRules:\n" "\t5 dice are rolled\n" "\tThe user selects which dice to roll again.\n" "\tThe user may choose to roll none or all 5 or any combination.\n" "\tAnd then the user selects which dice to roll, yet again.\n" "\tAfter this second reroll the turn is scored.\n\n" "\tScoring is as follows:\n" "\t\t*\t50 points \t5 of a kind scores 50 points.\n" "\t\t*\t45 points \tNo pairs (all unique) scores 45…arrow_forward
- POEM List[POEM_LINE] PRONUNCIATION_DICT Dict[str, PHONEMES] Do not add statements that call print, input, or open, or use an import statement. Do not use any break or continue statements.arrow_forwardhere is a and b part plz solve c part #include <iostream>#include <string.h>#include <stdlib.h>#include <sstream>#include <cmath>using namespace std; int main(){ /*Variable declarations*/int higestDegree,higestDegree1;int diffDegree,smallDegree;std::stringstream polynomial,addpolynomial;/*prompt for degree*/std::cout<<"Please enter higest degree of polynomial"<<std::endl;std::cin>>higestDegree; std::cout<<"Please Enter highest degree of 2nd polynomial"<<std::endl;std::cin>>higestDegree1;int *cofficient=new int[higestDegree+1];//cofficient array creation for 1st polynomailint *cofficient1=new int[higestDegree1+1];//cofficient array creation for 2st polynomail /*taking cofficient for 1st polynomial*/std::cout<<"Please enter cofficient of each term from higest degree to lowest for 1st polynomial"<<std::endl;for(int i=0;i<=higestDegree;i++){std::cin>>cofficient[i];}/*taking cofficient for 2st…arrow_forward#include <iostream>#include <list>#include <string>#include <cstdlib>using namespace std;int main(){int myints[] = {};list<int> l, l1 (myints, myints + sizeof(myints) / sizeof(int));list<int>::iterator it;int choice, item;while (1){cout<<"\n---------------------"<<endl;cout<<"***List Implementation***"<<endl;cout<<"\n---------------------"<<endl;cout<<"1.Insert Element at the Front"<<endl;cout<<"2.Insert Element at the End"<<endl;cout<<"3.Front Element of List"<<endl;cout<<"4.Last Element of the List"<<endl;cout<<"5.Size of the List"<<endl;cout<<"6.Display Forward List"<<endl;cout<<"7.Exit"<<endl;cout<<"Enter your Choice: ";cin>>choice;switch(choice){case 1:cout<<"Enter value to be inserted at the front: ";cin>>item;l.push_front(item);break;case 2:cout<<"Enter value to be inserted at the end:…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
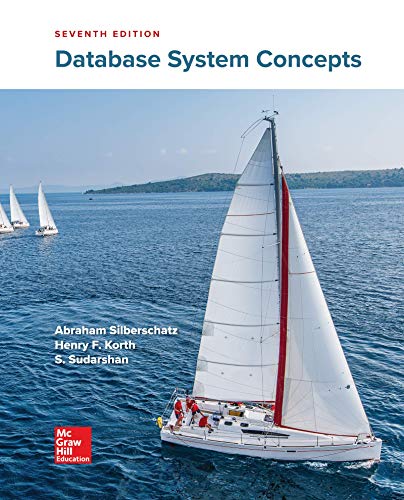
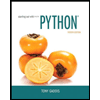
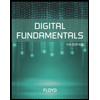
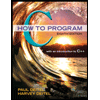
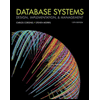
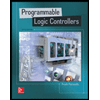