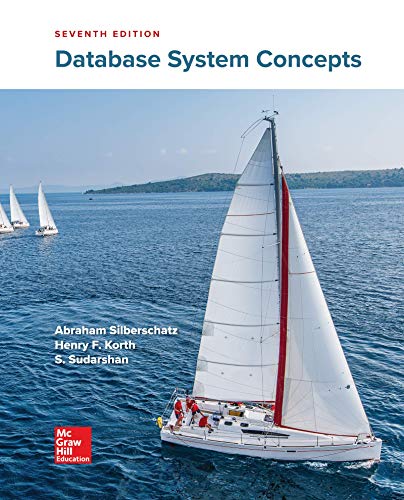
1. Display Function
- Implement a function to display the contents of the patient_list array.
- Add code to call this function. Note that there are multiple places where this function needs to be called. Look for the // TODO comments to find the correct locations.
- Compile and test your program.
- Make sure that your output is formatted similarly to the sample output shown at the end of this document. The sorting part does not need to work yet.
Take a screenshot of a sample output and upload the picture as part of your assignment submission.
2. Sorting the array by Age
- Implement the code to sort the contents of the patient_list array based on the value stored in the age field. To do this you will need to implement code that relies on the qsort function from the C Standard library
(see http://www.cplusplus.com/reference/cstdlib/qsort/). As shown in the reference, this code requires two parts: - A function that compares two patient elements, based on the value stored in the age field.
- A call to the qsort function, which includes the array to be sorted, the number of elements in the array, the size of each array element and the function used to compare the array elements.
- Add code to call the qsort function, using the age comparison function that you implemented. This code should be placed just under the appropriate // TODO comment in main().
- Compile and test your program.
- Make sure that your output is formatted similarly to the sample output shown at the end of this document. The first sorted list should be correct now.
Take a screenshot of a sample output and upload the picture as part of your assignment submission.
Sorting the array by Balance Due
- Implement the code to sort the contents of the patient_list array based on the value stored in the balance field. To do this you will need to implement code that relies on the qsort function from the C Standard library
(see http://www.cplusplus.com/reference/cstdlib/qsort/). As shown in the reference, this code requires two parts: - A function that compares two patient elements, based on the value stored in the balance field.
- A call to the qsort function, which includes the array to be sorted, the number of elements in the array, the size of each array element and the function used to compare the array elements.
- Add code to call the qsort function, using the balance comparison function that you implemented. This code should be placed just under the appropriate // TODO comment in main().
- Compile and test your program.
- Make sure that your output is formatted similarly to the sample output shown at the end of this document. The second sorted list should be correct now.
Take a screenshot of a sample output and upload the picture as part of your assignment submission.
Sorting the array by Patient Name
- Implement the code to sort the contents of the patient_list array based on the value stored in the name field. To do this you will need to implement code that relies on the qsort function from the C Standard library
(see http://www.cplusplus.com/reference/cstdlib/qsort/). As shown in the reference, this code requires two parts: - A function that compares two patient elements, based on the value stored in the name field. Because name data is stored in an array of characters, you cannot use the relational operators (<, >, <=, … ) to do the comparison, instead you should use a function from the C Standard Library to determine the order of the names, see (http://www.cplusplus.com/reference/cstring/strncmp/).
- A call to the qsort function, which includes the array to be sorted, the number of elements in the array, the size of each array element and the function used to compare the array elements.
- Add code to call the qsort function, using the name comparison function that you implemented. This code should be placed just under the appropriate // TODO comment in main().
- Compile and test your program.
- Make sure that your output is formatted similarly to the sample output shown at the end of this document. The third and final sorted list should be correct now.
Take a screenshot of a sample output and upload the picture as part of your assignment submission.
Sample (partial) Output for Completed Solution:
Note: this sample output only shows the existing list of patients ; your output should additionally show the entry with your details.
OUTPUT IN IMAGES
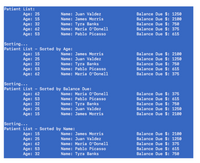

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 2 images

- Matlab Questionarrow_forwarda. Create a 1D array of integers to store 50 integers. b. Store values from 0 to 49 into the array you just created. c. Create a new String Object using no-arg constructor. d. Using for loop append the array elements one by one to the String (one per loop iteration) e. Record and display a run-time it took to append all integers to the String (record run-time of 1.d.)). E.g. "It took nanoseconds to append 50 integers to the Stringarrow_forwardPYTHON Complete the function below, which takes two arguments: data: a list of tweets search_words: a list of search phrases The function should, for each tweet in data, check whether that tweet uses any of the words in the list search_words. If it does, we keep the tweet. If it does not, we ignore the tweet. data = ['ZOOM earnings for Q1 are up 5%', 'Subscriptions at ZOOM have risen to all-time highs, boosting sales', "Got a new Mazda, ZOOM ZOOM Y'ALL!", 'I hate getting up at 8am FOR A STUPID ZOOM MEETING', 'ZOOM execs hint at a decline in earnings following a capital expansion program'] Hint: Consider the example_function below. It takes a list of numbers in numbers and keeps only those that appear in search_numbers. def example_function(numbers, search_numbers): keep = [] for number in numbers: if number in search_numbers(): keep.append(number) return keep def search_words(data, search_words):arrow_forward
- Section A. Declare and initialize an array myChars containing the following characters: B, C, D, A, M, S and F.Remember to choose the correct data type for the array elements.Section B. Using a WHILE loop, output the elements of the array myChars so that they are displayed on thesame line and separated by a space. Use .length to get the length of the array.Section C. Change the last value of the array myChars to the letter X. Use .length in your code.Section D. Using a FOR loop, output the elements of the myChars array so that they are displayed on the sameline and separated by a dash (an extra dash at the end is okay). Use .length in your code.Section E. Declare an array of int of capacity 10 named thisArray.Section F. Use a FOR loop to populate the array thisArray with the following numbers 5, 10, 15, 20, 25. Thinkof a way to insert these numbers without actually writing “5” or “10” and so on, but by using the variable i ofyour FOR loop. NOTE that this is a partially-filled array and…arrow_forward6. In NumPy, write a program to show the syntax to print the data type of an array. Take the sample array as [1, 2, 3, 4, 5]arrow_forwardrun the program and please attach the screenshot of the outputarrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
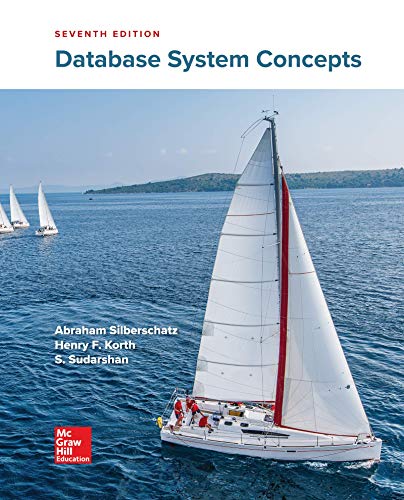
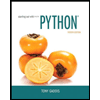
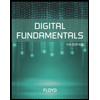
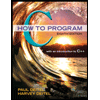
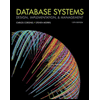
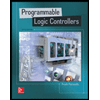