Java Code: Below is Parser.java and there are errors. getType() and getStart() is undefined for the type Optional and there is an error in addNode(). Make sure to get rid of all the errors in the code. Attached is images of the errors. Parser.java import java.text.ParseException; import java.util.LinkedList; import java.util.List; import java.util.Optional; import javax.swing.ActionMap; public class Parser { private TokenHandler tokenHandler; private LinkedList tokens; public Parser(LinkedList tokens) { this.tokenHandler = new TokenHandler(tokens); this.tokens = tokens; } public boolean AcceptSeparators() { boolean foundSeparator = false; while (tokenHandler.MoreTokens()) { Optional currentToken = tokenHandler.getCurrentToken(); if (currentToken.getType() == Token.TokenType.NEWLINE || currentToken.getType() == Token.TokenType.SEMICOLON) { tokenHandler.consumeMatchedToken(); foundSeparator = true; } else { break; } } return foundSeparator; } public ProgramNode Parse() throws ParseException { ProgramNode programNode = new ProgramNode(null, 0); while (tokenHandler.MoreTokens()) { if (!ParseFunction(programNode) && !parseAction(programNode)) { throw new ParseException("Unexpected token: " + tokenHandler.getCurrentToken(), tokenHandler.getCurrentToken().getStart()); } } return programNode; } private boolean ParseFunction(ProgramNode programNode) { Optional functionNameToken = tokenHandler.getCurrentToken(); if (tokenHandler.MatchAndRemove(Token.TokenType.IDENTIFIER) != null) { FunctionDefinitionNode functionNode = new FunctionDefinitionNode(functionNameToken.getType()); programNode.addNode(functionNode); if (tokenHandler.MatchAndRemove(Token.TokenType.LPAREN) != null) { if (tokenHandler.MatchAndRemove(Token.TokenType.RPAREN) != null) { AcceptSeparators(); return true; } } } return false; } private boolean parseAction(ProgramNode programNode) { Optional functionNameToken = tokenHandler.getCurrentToken(); if (tokenHandler.MatchAndRemove(Token.TokenType.IDENTIFIER) != null) { ActionMap actionMap = new ActionMap(tokenHandler.getLastMatchedToken().getType()); programNode.addNode(actionMap); AcceptSeparators(); return true; } return false; } }
Java Code: Below is Parser.java and there are errors. getType() and getStart() is undefined for the type Optional<Token> and there is an error in addNode(). Make sure to get rid of all the errors in the code. Attached is images of the errors.
Parser.java
import java.text.ParseException;
import java.util.LinkedList;
import java.util.List;
import java.util.Optional;
import javax.swing.ActionMap;
public class Parser {
private TokenHandler tokenHandler;
private LinkedList<Token> tokens;
public Parser(LinkedList<Token> tokens) {
this.tokenHandler = new TokenHandler(tokens);
this.tokens = tokens;
}
public boolean AcceptSeparators() {
boolean foundSeparator = false;
while (tokenHandler.MoreTokens()) {
Optional<Token> currentToken = tokenHandler.getCurrentToken();
if (currentToken.getType() == Token.TokenType.NEWLINE || currentToken.getType() == Token.TokenType.SEMICOLON) {
tokenHandler.consumeMatchedToken();
foundSeparator = true;
} else {
break;
}
}
return foundSeparator;
}
public ProgramNode Parse() throws ParseException {
ProgramNode programNode = new ProgramNode(null, 0);
while (tokenHandler.MoreTokens()) {
if (!ParseFunction(programNode) && !parseAction(programNode)) {
throw new ParseException("Unexpected token: " + tokenHandler.getCurrentToken(), tokenHandler.getCurrentToken().getStart());
}
}
return programNode;
}
private boolean ParseFunction(ProgramNode programNode) {
Optional<Token> functionNameToken = tokenHandler.getCurrentToken();
if (tokenHandler.MatchAndRemove(Token.TokenType.IDENTIFIER) != null) {
FunctionDefinitionNode functionNode = new FunctionDefinitionNode(functionNameToken.getType());
programNode.addNode(functionNode);
if (tokenHandler.MatchAndRemove(Token.TokenType.LPAREN) != null) {
if (tokenHandler.MatchAndRemove(Token.TokenType.RPAREN) != null) {
AcceptSeparators();
return true;
}
}
}
return false;
}
private boolean parseAction(ProgramNode programNode) {
Optional<Token> functionNameToken = tokenHandler.getCurrentToken();
if (tokenHandler.MatchAndRemove(Token.TokenType.IDENTIFIER) != null) {
ActionMap actionMap = new ActionMap(tokenHandler.getLastMatchedToken().getType());
programNode.addNode(actionMap);
AcceptSeparators();
return true;
}
return false;
}
}
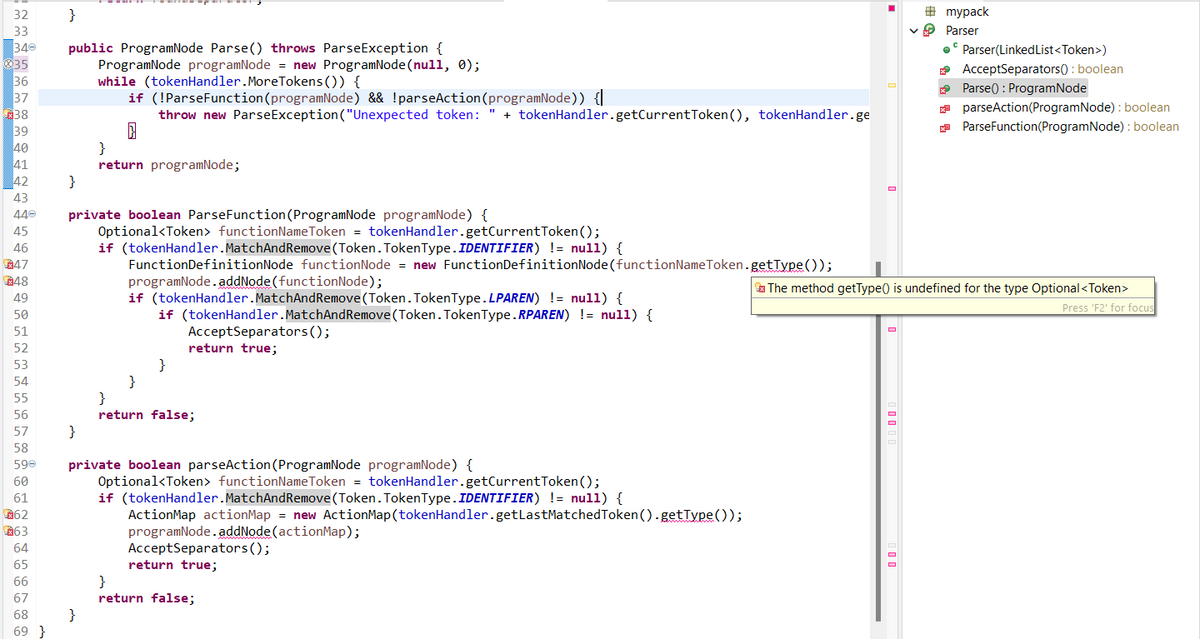
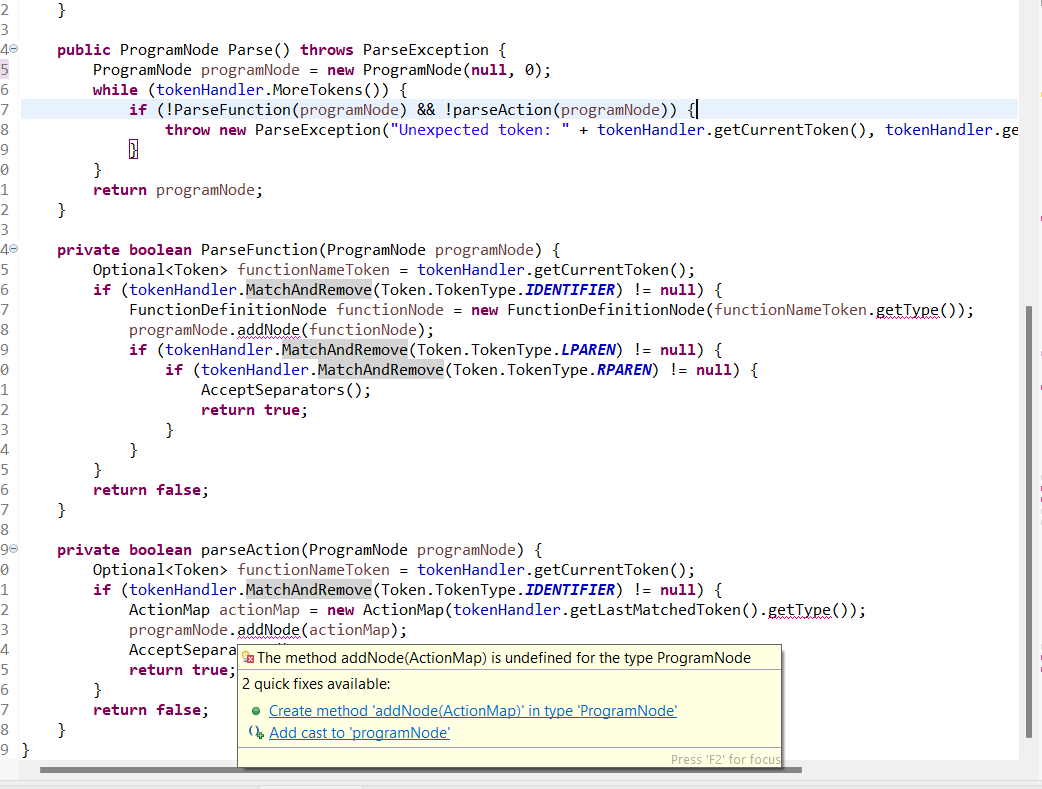

Step by step
Solved in 3 steps

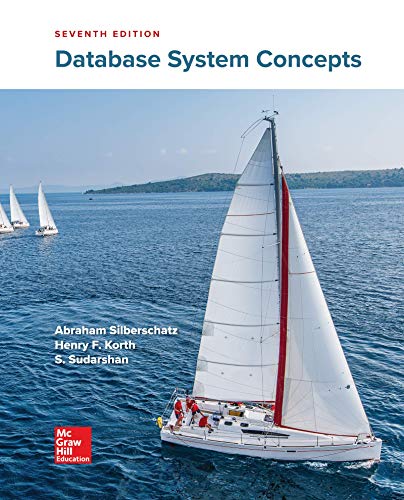
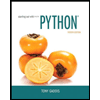
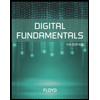
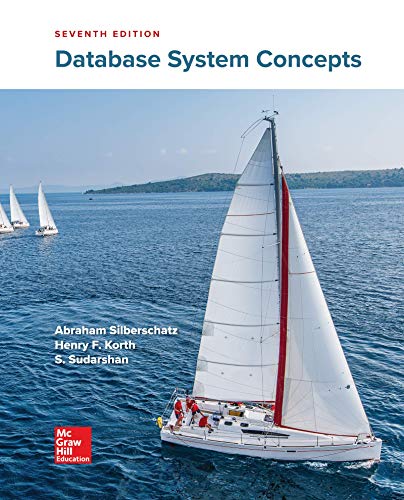
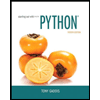
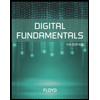
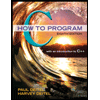
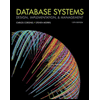
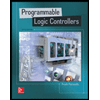