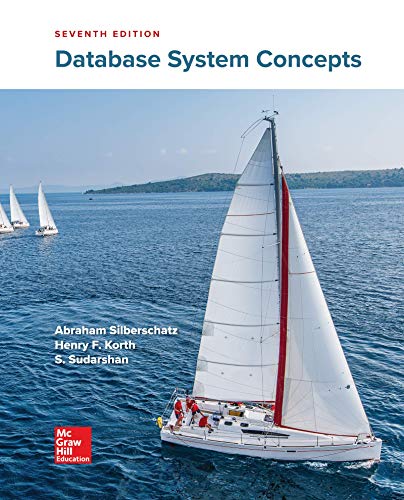
Change this code in C to Create functions and function prototypes to replace each of the
calculator actions. Prototype each function above main and write the
function (definition) below main.
- The input by the user and output statement of each calculation should
stay in main and not be moved to a function except the output for
function “iseven” which should be done in the function.
- Each function will accept the value(s) supplied by the user as parameters
and return the result in a return statement (not a printf statement
except for “iseven”).
- The result returned by each function should be outputted by a printf
statement in main.
- Create a function named display that contains: menu display
statements, the user prompt to select an option, user input. This
function should have no parameters and should return a character as
the option chosen by the user.
----------------------------------------------------------------------------------------------------------
#include<math.h>
#include<stdio.h>
int main(void)
{
char menuoption;
int intx, inty, answeri, count, n, fact;
double doublex, doubley, answerd;
//prompt user
do{
//menu
printf("\nOperation Menu Option\n");
printf("Add A\n");
printf("Subtract S\n");
printf("Multiply M\n");
printf("Divide D\n");
printf("Remainder R\n");
printf("Squared V\n");
printf("Square Root T\n");
printf("Factorial F\n");
printf("Is even E\n");
printf("Greater integer G\n");
printf("Less integer L\n");
printf("Exit program X\n\n");
printf("Please enter a menu option from the table above: ");
scanf("%c", &menuoption);
switch (menuoption)
{
//addition
case'a': case'A':
printf("Please enter the first number: ");
scanf("%lf", &doublex);
printf("Please enter the second number: ");
scanf("%lf", &doubley);
answerd= doublex + doubley;
printf("Answer = %.2lf", answerd);
break;
//subtraction
case 's': case'S':
printf("Please enter the first number:");
scanf("%lf", &doublex);
printf("Please enter the second number:");
scanf("%lf", &doubley);
answerd= doublex-doubley;
printf("Answer = %.2lf", answerd);
break;
//multiplication
case 'm': case'M':
printf("Please enter the first number:");
scanf("%lf", &doublex);
printf("Please enter the second number:");
scanf("%lf", &doubley);
answerd= doublex * doubley;
printf("Answer = %.2f\n", answerd);
break;
//division
case 'd': case'D':
printf("Please enter the first number:");
scanf("%lf", &doublex);
printf("Please enter the second number:");
scanf("%lf", &doubley);
if (doubley == 0) //checking if denominator
is zero
printf("invalid, cannot divide by zero\n");
else
{
answerd= doublex / doubley;
printf("Answer = %.2f\n", answerd);
}
break;
//remainder
case 'r': case'R':
printf("Please enter the first number:");
scanf("%d", &intx);
printf("Please enter the second number:");
scanf("%d", &inty);
answeri= intx % inty;
printf("Answer = %d\n", answeri);
break;
//squared
case 'v': case'V':
printf("Please enter the number to be squared:");
scanf("%lf", &doublex);
answerd= doublex*doublex;
printf("Answer = %.2lf", answerd);
break;
//square root
case 't': case'T':
printf("Please enter the number to take the square root of:");
scanf("%lf", &doublex);
if (doublex < 0) // checking
is number for square root is negative
printf("invalid, cannot square root negative number\n");
else
{
answerd= sqrt(doublex);
printf("Answer = %.2f\n", answerd);
}
break;
//factorial
case 'f': case'F':
fact = 1;
printf("Please enter the number to take the factorial:");
scanf("%d", &n);
if (n<0)
printf("Cannot take the factorial of a negative number.");
else if (n>0)
{
for (count= n ; count>= 1; count--)
{
fact = fact * count;
}
printf("Factorial = %d", fact );
}
else
printf("The factorial of 0 is 1.");
break;
//even
case 'e': case'E':
printf("Please enter the first number:");
scanf("%d", &intx);
answeri= intx % 2;
if (answeri==0)
printf("The number is even.\n");
else
printf("The number is not even.\n");
break;
//greater integer
case 'g': case'G':
printf("Please enter the first number:");
scanf("%lf", &doublex);
answeri= ceil(doublex);
printf("Answer = %d", answeri);
break;
//lesser integer
case 'l': case'L':
printf("Please enter the first number:");
scanf("%d", &doublex);
answeri= floor(doublex) ;
printf("Answer = %d", answeri);
break;
//exit program
case 'x': case'X':
return 0;
break;
//improper input
default:
printf("Input not recognized.\n");
}
} while (1);
return 0;
}

Trending nowThis is a popular solution!
Step by stepSolved in 5 steps with 10 images

- Call by value in programming means that a copy of variable value is pased from function calling part to function definition part. Call by reference means that an address of variables are passed from calling part to function definition. arrow_forward Step 2 Call by reference is used to avoid extra memory usage because in call by reference same variables addresses are passed. Any changes made will reflect back. So it doesn't require extra variable to hold data. Call by value in programming is used where memory storage is not concern. Scenario in which actual value must not be disturbed then in these type of cases, call by value is used. We can't use only one because user requirement changes. Sometimes user tella actual data should be preserved and he/she want any data processing, in such scenario programmers use call by value. Some users only want calculated result. In such cases, programmers use call by reference to preserve memory usage. So it is totally dependent on user…arrow_forwardQUESTION 3 Which of the following statements is TRUE about function? A function is activated through its function prototype. Every function must return a type and contains a list of parameters. If a function is declared as an integer it will return a decimal value. A group of statements placed together to perform a task.arrow_forwardQuestion 3 Formal parameters of a function are available outside the function. True False Question 4arrow_forward
- Write a statement that declares a prototype for a function divide that takes four arguments and returns no value. The first two arguments are of type int. The last two arguments arguments are pointers to int that are set by the function to the quotient and remainder of dividing the first argument by the second argument. The function does not return a value.arrow_forwardWhat are the assertions that describe how a function works?arrow_forwardAll lines of code in your program can be reached and executed when I run your program define two functions and they have a "meaningful purpose" to your program. Each function must have more than three statements or lines of code. At least one function defines a parameter and you use this parameter in a meaningful way At least one function returns a value back to the code that is calling it and it is used in a meaningful wayarrow_forward
- Write the function prototype of the void function named G() that takes an int reference parameter and two char parameters respectively Your answerarrow_forwardWhat does it mean to provide parameters to a function in the right order when it accepts more than one?arrow_forwardO d. The actual parameters are defined in the header of a function; for example, in the following function the actual parameters are x and y: def f(x,y): The formal parameters are those provided to a function at the time the function is called; for example, 5 and 15 are the formal parameters of the function f: k f(5,15) Ое. The formal parameters are defined in the header of a function; for example, in the following function the formal parameters are 5 and 15: def f(5,15): The actual parameters are those provided to a function at the time the function is called; for example, x and y are the actual parameters of the function f: k f(x,y) %Darrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
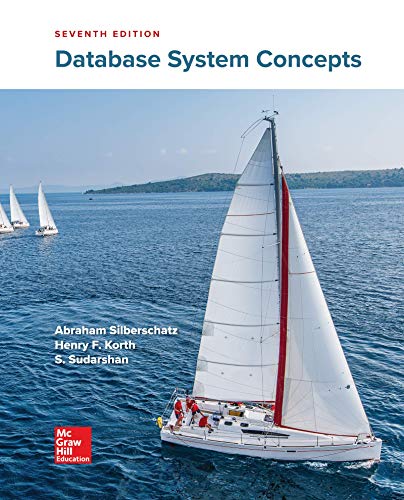
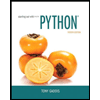
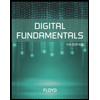
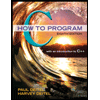
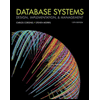
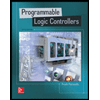