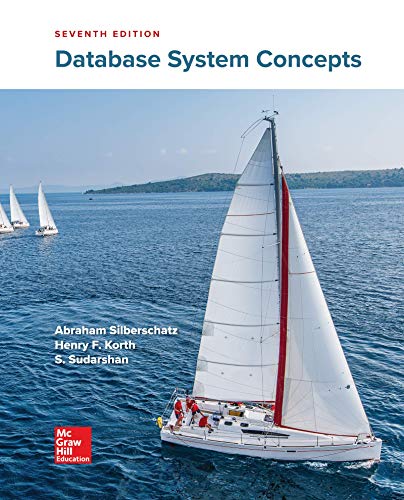
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Question
thumb_up100%
Java Shell Sort but make it read the data 12, 34, 54, 2, 3 from a file not an array
// Java implementation of ShellSort
class ShellSort
{
/* An utility function to print array of size n*/
static void printArray(int arr[])
{
int n = arr.length;
for (int i=0; i<n; ++i)
System.out.print(arr[i] + " ");
System.out.println();
}
/* function to sort arr using shellSort */
int sort(int arr[])
{
int n = arr.length;
// Start with a big gap, then reduce the gap
for (int gap = n/2; gap > 0; gap /= 2)
{
// Do a gapped insertion sort for this gap size.
// The first gap elements a[0..gap-1] are already
// in gapped order keep adding one more element
// until the entire array is gap sorted
for (int i = gap; i < n; i += 1)
{
// add a[i] to the elements that have been gap
// sorted save a[i] in temp and make a hole at
// position i
int temp = arr[i];
// shift earlier gap-sorted elements up until
// the correct location for a[i] is found
int j;
for (j = i; j >= gap && arr[j - gap] > temp; j -= gap)
arr[j] = arr[j - gap];
// put temp (the original a[i]) in its correct
// location
arr[j] = temp;
}
}
return 0;
}
// Driver method
public static void main(String args[])
{
int arr[] = {12, 34, 54, 2, 3}; // make it read from a file instead of having this
System.out.println("Array before sorting");
printArray(arr);
ShellSort ob = new ShellSort();
ob.sort(arr);
System.out.println("Array after sorting");
printArray(arr);
}
}
/*This code is contributed by Rajat Mishra */
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution
Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Select the for-loop which iterates through all even index values of an array.A. for(int idx = 0; idx < length; idx++)B. for(int idx = 0; idx < length; idx%2)C. for(int idx = 0; idx < length; idx+2)D. for(int idx = 0; idx < length; idx=idx+2)arrow_forwardI need this program in java please. #Given Arrayarray=[1,1,1,2,2,2,2,2,4,5,5,6,6,6] #Insert valuevalue=3 index=0for x in array: if value<x: #inserting to the array in the position index array.insert(index,value) print ("Output :",array) break #Incrementing position by 1 index=index+1arrow_forwardWrite the function lastOf which searches the array a for the last occurance of any value contained in the array b. Returns the index if found. If not found, return -1. arrays.cpp 1 #include 2 3 int lastof(const int a[), int alen, const int b[], int blen) 4 { int res = 0; for (int i = 0; i =0 ; j--) if (b[j] == a[i]) { if(res[1, 9, 4, 5, 1, 5, 1, 12, 8, 11, 2, 11, 7, 8] b1->[7, 5, 9] last0f(al, 14, b1, 3): -1 Expected: 12 al->[1, 9, 4, 5, 1, 5, 1, 12, 8, 11, 2, 11, 7, 8] b2->[7, 7] lastof (al, 14, b2, 2): -1 Expected: 12 a2->[3, 2, 1, 14, 10, 6, 13, 1ө, 11, 13, 14] b2->[7, 7] lastof (a2, 11, b2, 2): -1 Expected: -1 а2->[3, 2, 1, 14, 10, 6, 13, 1ө, 11, 13, 14] b1->[7, 5, 9] last0f (a2, 11, b1, 3): -1 Expected: -1 а2->[3, 2, 1, 14, 10, 6, 13, 10, 11, 13, 14] b3->[11, 2, 1, 15] lastof (a2, 11, b3, 4): -1 Expected: 8 Score 2/5arrow_forward
- Java program In main method: Create 2-D Array, called m: the row size of array m is 15 ask user to input column size of array m fill all array elements as double numbers in the range of (7.0, 17.0) by using random object create 2-d array, called double [][]md = {{1,1,1},{2,0,1},{4,7,4}}; pass the above array m and md to call the following two methods MaxCol(m); MaxCol(md); System.out.println (“the average of array m is: ” + returnLast3RowAvg(m)); System.out.println (“the average of array md is: ” + returnLast3RowAvg(md)); Print out the largest column sum, and the average of the last 3 rows of array m and md. In MaxCol(double[][]array) method, find and print the largest column sum in the array. In returnLast3RowAvg(double[][]array) method, find the average of the elements in the last 3 rows in the array and return this average value.arrow_forwardpublic static void reverse(ArrayList<Integer> alist){}public static ArrayList<Integer> reverseList(ArrayList<Integer> alist){} How to use these two headers method and a main method to create the output of [1,2,3] [3,2,1]arrow_forwardFill in the blanks of initialization of std::array array of int type, an_int_array with content {1, 2, 3). stà Then fill in the blanks in such a way that the following for loop simply prints 1, 2, 3, to standard output. for(int i = 0; i an_int_array {1, 2, 3); • you must assume using namespace std; has not being used assume the regular for loop pattern in the question abovearrow_forward
- int arr[] = {-2, -4, 8, 4, 3, 3, -9};In Java with this given this array, how would this version of Divide and Conqueror be implemented in this case, specifically?arrow_forwardGiven the Array declaration below, determine the output: int T[8] = {2, 3, 7, 9, -1, 2, 4, 5}; int x = 5; cout << T[x-2] / T[12-2*x]; int y = -3; cout << pow(T[y+4],T[3-y]);arrow_forwardjhfgjgjgjarrow_forward
arrow_back_ios
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
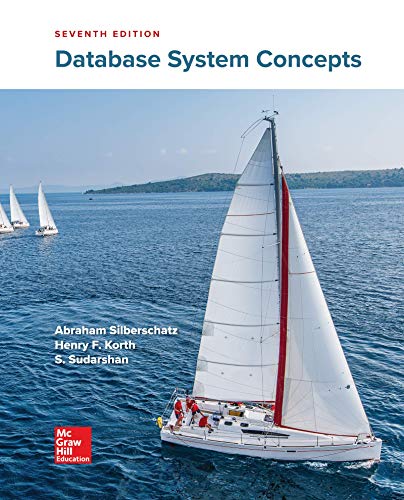
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
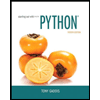
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
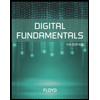
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
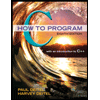
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
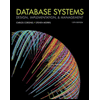
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
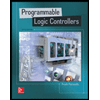
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education