Java Bubble Sort but make it read the data 64, 34, 25, 12, 22, 11, 90 from a file not an array // Java program for implementation of Bubble Sort class BubbleSort { void bubbleSort(int arr[]) { int n = arr.length; for (int i = 0; i < n - 1; i++) for (int j = 0; j < n - i - 1; j++) if (arr[j] > arr[j + 1]) { // swap arr[j+1] and arr[j] int temp = arr[j]; arr[j] = arr[j + 1]; arr[j + 1] = temp; } } /* Prints the array */ void printArray(int arr[]) { int n = arr.length; for (int i = 0; i < n; ++i) System.out.print(arr[i] + " "); System.out.println(); } // Driver method to test above public static void main(String args[]) { BubbleSort ob = new BubbleSort(); int arr[] = { 64, 34, 25, 12, 22, 11, 90 }; // make it read from a file instead of having this ob.bubbleSort(arr); System.out.println("Sorted array"); ob.printArray(arr); } } /* This code is contributed by Rajat Mishra */
Java Bubble Sort but make it read the data 64, 34, 25, 12, 22, 11, 90 from a file not an array // Java program for implementation of Bubble Sort class BubbleSort { void bubbleSort(int arr[]) { int n = arr.length; for (int i = 0; i < n - 1; i++) for (int j = 0; j < n - i - 1; j++) if (arr[j] > arr[j + 1]) { // swap arr[j+1] and arr[j] int temp = arr[j]; arr[j] = arr[j + 1]; arr[j + 1] = temp; } } /* Prints the array */ void printArray(int arr[]) { int n = arr.length; for (int i = 0; i < n; ++i) System.out.print(arr[i] + " "); System.out.println(); } // Driver method to test above public static void main(String args[]) { BubbleSort ob = new BubbleSort(); int arr[] = { 64, 34, 25, 12, 22, 11, 90 }; // make it read from a file instead of having this ob.bubbleSort(arr); System.out.println("Sorted array"); ob.printArray(arr); } } /* This code is contributed by Rajat Mishra */
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN:9781337102087
Author:D. S. Malik
Publisher:D. S. Malik
Chapter18: Stacks And Queues
Section: Chapter Questions
Problem 16PE:
The implementation of a queue in an array, as given in this chapter, uses the variable count to...
Related questions
Question
100%
Java Bubble Sort but make it read the data 64, 34, 25, 12, 22, 11, 90 from a file not an array
// Java program for implementation of Bubble Sort
class BubbleSort {
void bubbleSort(int arr[])
{
int n = arr.length;
for (int i = 0; i < n - 1; i++)
for (int j = 0; j < n - i - 1; j++)
if (arr[j] > arr[j + 1]) {
// swap arr[j+1] and arr[j]
int temp = arr[j];
arr[j] = arr[j + 1];
arr[j + 1] = temp;
}
}
/* Prints the array */
void printArray(int arr[])
{
int n = arr.length;
for (int i = 0; i < n; ++i)
System.out.print(arr[i] + " ");
System.out.println();
}
// Driver method to test above
public static void main(String args[])
{
BubbleSort ob = new BubbleSort();
int arr[] = { 64, 34, 25, 12, 22, 11, 90 }; // make it read from a file instead of having this
ob.bubbleSort(arr);
System.out.println("Sorted array");
ob.printArray(arr);
}
}
/* This code is contributed by Rajat Mishra */
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 5 steps with 3 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
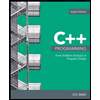
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
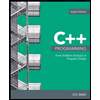
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning