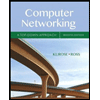
C++
This is the given code:
#include <
#include <iostream>
#include <algorithm>
using namespace std;
// The puzzle will always have exactly 20 columns
const int numCols = 20;
// Searches the entire puzzle, but may use helper functions to implement logic
void searchPuzzle(const char puzzle[][numCols], const string wordBank[],
vector <string> &discovered, int numRows, int numWords);
// Printer function that outputs a vector
void printVector(const vector <string> &v);
// Example of one potential helper function.
// bool searchPuzzleToTheRight(const char puzzle[][numCols], const string &word,
// int rowStart, int colStart)
int main()
{
int numRows, numWords;
// grab the array row dimension and amount of words
cin >> numRows >> numWords;
// declare a 2D array
char puzzle[numRows][numCols];
// TODO: fill the 2D array via input
// read the puzzle in from the input file using cin
// create a 1D array for wods
string wordBank[numWords];
// TODO: fill the wordBank through input using cin
// set up discovery vector
vector <string> discovered;
// Search for Words
searchPuzzle(puzzle, wordBank, discovered, numRows, numWords);
// Sort the results
sort(discovered.begin(), discovered.end());
// Print vector of discovered words
printVector(discovered);
return 0;
}
// TODO: implement searchPuzzle and any helper functions you want
void searchPuzzle(const char puzzle[][numCols], const string wordBank[],
vector <string> &discovered, int numRows, int numWords)
{
}
// TODO: implement printVector
void printVector(const vector <string> &v)
{
}

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps

- // MichiganCities.cpp - This program prints a message for invalid cities in Michigan. // Input: Interactive // Output: Error message or nothing #include <iostream> #include <string> using namespace std; int main() { // Declare variables string inCity; // name of city to look up in array const int NUM_CITIES = 10; // Initialized array of cities string citiesInMichigan[] = {"Acme", "Albion", "Detroit", "Watervliet", "Coloma", "Saginaw", "Richland", "Glenn", "Midland", "Brooklyn"}; bool foundIt = false; // Flag variable int x; // Loop control variable // Get user input cout << "Enter name of city: "; cin >> inCity; // Write your loop here // Write your test statement here to see if there is // a match. Set the flag to true if city is found. // Test to see if city was not found to determine if // "Not a city in Michigan" message should be printed.…arrow_forwardRemove Char This function will be given a list of strings and a character. You must remove all occurrences of the character from each string in the list. The function should return the list of strings with the character removed. Signature: public static ArrayList<String> removeChar(String pattern, ArrayList<String> list) Example:list: ['adndj', 'adjdlaa', 'aa', 'djoe']pattern: a Output: ['dndj', 'djdl', '', 'djoe']arrow_forwardstatic List<String> findWinners(String pattern, int minLength, boolean even, Stream<String> stream) This function will receive a stream of strings and it will return a sorted list of strings that meet all of the following criteria: They must contain the pattern The strings length must be equal to or greater than the min length number The strings length must be an even or odd number This Function must take no longer 10000 milliseconds to return. pattern - A pattern that all the strings in the list returned must containminLength - The length that all the strings in the list returned must be equal to or greater thaneven - True if even false if odd. Denotes a condition that all the strings in the list returned must have a length that is even or odd.stream - A stream of strings to be filteredreturn - A list of strings that meets the criteria outlined above sorted by their length from small to largearrow_forward
- struct remove_from_front_of_vector { // Function takes no parameters, removes the book at the front of a vector, // and returns nothing. void operator()(const Book& unused) { (/// TO-DO (12) ||||| // // // Write the lines of code to remove the book at the front of "my_vector". // // Remember, attempting to remove an element from an empty data structure is // a logic error. Include code to avoid that. //// END-TO-DO (12) /||, } std::vector& my_vector; };arrow_forwardQ# Describe what a data structure is for a char**? (e.g. char** argv) Group of answer choices A. It is a pointer to a single c-string. B. It is an array of c-strings C. It is the syntax for dereferencing a c-string and returning the value.arrow_forward#include <iostream>#include <cstdlib>#include <time.h>#include <chrono> using namespace std::chrono;using namespace std; void randomVector(int vector[], int size){ for (int i = 0; i < size; i++) { //ToDo: Add Comment vector[i] = rand() % 100; }} int main(){ unsigned long size = 100000000; srand(time(0)); int *v1, *v2, *v3; //ToDo: Add Comment auto start = high_resolution_clock::now(); //ToDo: Add Comment v1 = (int *) malloc(size * sizeof(int *)); v2 = (int *) malloc(size * sizeof(int *)); v3 = (int *) malloc(size * sizeof(int *)); randomVector(v1, size); randomVector(v2, size); //ToDo: Add Comment for (int i = 0; i < size; i++) { v3[i] = v1[i] + v2[i]; } auto stop = high_resolution_clock::now(); //ToDo: Add Comment auto duration = duration_cast<microseconds>(stop - start); cout << "Time taken by function: " << duration.count()…arrow_forward
- 23. In the C++ language write a program to find the product of all the elements present in the array of integers given below. int numArr[5] = {16,4, 8, 7, 12};arrow_forward### Import section# 3 necessary statements for numerical plotting in Jupyterimport numpy as npimport matplotlib.pyplot as plt %matplotlib inline# Factorial comes from 'math' packagefrom math import factorial ### Initialization section#array of derivatives at 0 compute by handderiv = np.array([1, 0, -1, 0, 1, 0, -1, 0])# degree of Taylor polynomialN=len(deriv)-1 # Max abs. value of N+1 deriv on interval (Compute using calculus)# (used in the remainder formula)# @@@ The N+1 derivative will be proportional to cosine, and |cos| is always # @@@ less than 1maxAbsNplus1deriv=1 #array with h valuesh = np.arange(-3,3.005,0.01) # Give the expansion point for the Taylor seriesexpansion=0 # Create an array to contain Taylor seriestaylor = np.zeros(len(h)) ### Calculation section#calculate Taylor series for all h at once using a loopfor n in range(N+1): taylor = taylor + (h**n)/factorial(n)*deriv[n] #actual function values actualFn = np.cos(expansion+h) #error actual and taylor…arrow_forwardUse stack concepts Q #2 Ô https://www.loc-cs.org/~chu/DataStructu Essay Questions (20% each)- continue [0] [1] [2] [3] myList "Bashful" "Awful" Jumpy "Happy The above "myList" is an ArrayList in Java program. 2. If I want to remove "Awful" from myList, which of the following actions should I take first? a. Move "Happy" to the previous element first. b. Move "Jumpy" to the previous element first. Describe the reason of your choice. Next Pagearrow_forward
- initial c++ file/starter code: #include <vector>#include <iostream>#include <algorithm> using namespace std; // The puzzle will always have exactly 20 columnsconst int numCols = 20; // Searches the entire puzzle, but may use helper functions to implement logicvoid searchPuzzle(const char puzzle[][numCols], const string wordBank[],vector <string> &discovered, int numRows, int numWords); // Printer function that outputs a vectorvoid printVector(const vector <string> &v);// Example of one potential helper function.// bool searchPuzzleToTheRight(const char puzzle[][numCols], const string &word,// int rowStart, int colStart) int main(){int numRows, numWords; // grab the array row dimension and amount of wordscin >> numRows >> numWords;// declare a 2D arraychar puzzle[numRows][numCols];// TODO: fill the 2D array via input// read the puzzle in from the input file using cin // create a 1D array for wodsstring wordBank[numWords];// TODO:…arrow_forward#include <iostream>#include <list>#include <string>#include <cstdlib>using namespace std;int main(){int myints[] = {};list<int> l, l1 (myints, myints + sizeof(myints) / sizeof(int));list<int>::iterator it;int choice, item;while (1){cout<<"\n---------------------"<<endl;cout<<"***List Implementation***"<<endl;cout<<"\n---------------------"<<endl;cout<<"1.Insert Element at the Front"<<endl;cout<<"2.Insert Element at the End"<<endl;cout<<"3.Front Element of List"<<endl;cout<<"4.Last Element of the List"<<endl;cout<<"5.Size of the List"<<endl;cout<<"6.Display Forward List"<<endl;cout<<"7.Exit"<<endl;cout<<"Enter your Choice: ";cin>>choice;switch(choice){case 1:cout<<"Enter value to be inserted at the front: ";cin>>item;l.push_front(item);break;case 2:cout<<"Enter value to be inserted at the end:…arrow_forward#include <iostream>#include <vector>using namespace std; void PrintVectors(vector<int> numsList) { unsigned int i; for (i = 0; i < numsList.size(); ++i) { cout << numsList.at(i) << " "; } cout << endl;} int main() { vector<int> numsList; int userInput; int i; for (i = 0; i < 3; ++i) { cin >> userInput; numsList.push_back(userInput); } numsList.erase(numsList.begin()+1); numsList.insert(numsList.begin()+1, 102); numsList.insert(numsList.begin()+1, 100); PrintVectors(numsList); return 0;} Not all tests passed clearTesting with inputs: 33 200 10 Output differs. See highlights below. Special character legend Your output 33 100 102 10 Expected output 100 33 102 10 clearTesting with inputs: 6 7 8 Output differs. See highlights below. Special character legend Your output 6 100 102 8 Expected output 100 6 102 8 Not sure what I did wrong but the the 33…arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
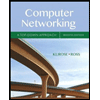
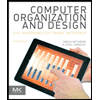
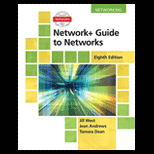
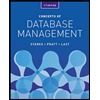
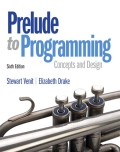
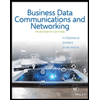