my code is getting errors and i cant figiure out why , can you check my code and help me fix the errors . written in c code. It needs to print out a maze and as you hit enter you go through the maze. #include <stdio.h>#include <stdlib.h> #define XSTART 2#define YSTART 0 #define DOWN 0#define RIGHT 1 #define UP 2#define LEFT 3 void mazeTraverse(char maze [12][12],int xCor,int yCor,int dir);int checkForEdge(int x , int y);int correctMoves (char maze[][12],int r , int c );void displayMaze (char maze [][12]); int main (void){ char maze [12] [12] ={{'#','#','#','#','#','#','#','#','#','#','#','#'}, {'#','.','.','.','#','.','.','.','.','.','.','#'}, {'.','.','#','.','#','.','#','#','#','#','.','#'}, {'#','#','#','.','#','.','.','.','.','#','.','#'}, {'#','.','.','.','.','#','#','#','.','#','.','.'}, {'#','#','#','#','.','#','.','#','.','#','.','#'}, {'#','.','.','#','.','#','.','#','.','#','.','#'}, {'#','#','.','#','.','#','.','#','.','#','.','#'}, {'#','.','.','.','.','.','.','.','.','#','.','#'}, {'#','#','#','#','#','#','.','#','#','#','.','#'}, {'#','.','.','.','.','.','.','#','.','.','.','#'}, {'#','#','#','#','#','#','#','#','#','#','#','#'} }; mazeTraverse(maze,XSTART,YSTART,RIGHT); return 0;} void mazeTraverse(char maze[12] [12] , int xCor ,int yCor , int dir) { static int flag =0; maze[xCor][yCor]='X'; displayMaze(maze); if (checkForEdge(xCor,yCor) && xCor != XSTART && yCor != YSTART) { printf("\n successfully exited the maze\n\n"); return; } else if (xCor == XSTART && yCor == YSTART && flag == 1) { printf("\nArrived back to starting position.\n\n"); return ; } else { int counter; int nextMove; flag = 1; for ( nextMove = dir , counter=0; counter < 4 ; ++counter,++nextMove, nextMove %= 4) { switch (nextMove) { case DOWN: if(correctMove (maze, xCor + 1, yCor )) { mazeTraverse(maze,xCor + 1,yCor,LEFT); return; } break; case RIGHT : if ( correctMove(maze,xCor,yCor + 1)) { mazeTraverse(maze, xCor,yCor + 1 , DOWN); return ; } break; case UP: if (correctMove(maze, xCor - 1,yCor)) { mazeTraverse(maze, xCor - 1 , yCor,RIGHT); return; } break; case LEFT : if(correctMove(maze, xCor,yCor - 1)) { mazeTraverse( maze , xCor,yCor -1, UP); return; } break; } } } int correctMove(char maze [][12],int r , int c ){ return(r>=0 && r <= 11 && c>= 0 && c <= 11 && maze[r][c] != '#' );} int checkForEdge(int x , int y ){ if ((x == 0 || x == 11)&& (y >= 0 && y <= 11)) { return 1; } else if ((y == 0 || y == 11) && (x >= 0 && x <= 11) ) { return 1; } else { return 0; }} void displayMaze(char maze [] [12]){ int x ; int y ; for (x = 0; x < 12 ; x++ ) { for (y = 0; y < 12 ; y++) { printf ( " %c ", maze[x] [y]); } printf("\n"); } printf("\n hit the return key to get next move"); getchar();}}
my code is getting errors and i cant figiure out why , can you check my code and help me fix the errors . written in c code. It needs to print out a maze and as you hit enter you go through the maze. #include <stdio.h>#include <stdlib.h> #define XSTART 2#define YSTART 0 #define DOWN 0#define RIGHT 1 #define UP 2#define LEFT 3 void mazeTraverse(char maze [12][12],int xCor,int yCor,int dir);int checkForEdge(int x , int y);int correctMoves (char maze[][12],int r , int c );void displayMaze (char maze [][12]); int main (void){ char maze [12] [12] ={{'#','#','#','#','#','#','#','#','#','#','#','#'}, {'#','.','.','.','#','.','.','.','.','.','.','#'}, {'.','.','#','.','#','.','#','#','#','#','.','#'}, {'#','#','#','.','#','.','.','.','.','#','.','#'}, {'#','.','.','.','.','#','#','#','.','#','.','.'}, {'#','#','#','#','.','#','.','#','.','#','.','#'}, {'#','.','.','#','.','#','.','#','.','#','.','#'}, {'#','#','.','#','.','#','.','#','.','#','.','#'}, {'#','.','.','.','.','.','.','.','.','#','.','#'}, {'#','#','#','#','#','#','.','#','#','#','.','#'}, {'#','.','.','.','.','.','.','#','.','.','.','#'}, {'#','#','#','#','#','#','#','#','#','#','#','#'} }; mazeTraverse(maze,XSTART,YSTART,RIGHT); return 0;} void mazeTraverse(char maze[12] [12] , int xCor ,int yCor , int dir) { static int flag =0; maze[xCor][yCor]='X'; displayMaze(maze); if (checkForEdge(xCor,yCor) && xCor != XSTART && yCor != YSTART) { printf("\n successfully exited the maze\n\n"); return; } else if (xCor == XSTART && yCor == YSTART && flag == 1) { printf("\nArrived back to starting position.\n\n"); return ; } else { int counter; int nextMove; flag = 1; for ( nextMove = dir , counter=0; counter < 4 ; ++counter,++nextMove, nextMove %= 4) { switch (nextMove) { case DOWN: if(correctMove (maze, xCor + 1, yCor )) { mazeTraverse(maze,xCor + 1,yCor,LEFT); return; } break; case RIGHT : if ( correctMove(maze,xCor,yCor + 1)) { mazeTraverse(maze, xCor,yCor + 1 , DOWN); return ; } break; case UP: if (correctMove(maze, xCor - 1,yCor)) { mazeTraverse(maze, xCor - 1 , yCor,RIGHT); return; } break; case LEFT : if(correctMove(maze, xCor,yCor - 1)) { mazeTraverse( maze , xCor,yCor -1, UP); return; } break; } } } int correctMove(char maze [][12],int r , int c ){ return(r>=0 && r <= 11 && c>= 0 && c <= 11 && maze[r][c] != '#' );} int checkForEdge(int x , int y ){ if ((x == 0 || x == 11)&& (y >= 0 && y <= 11)) { return 1; } else if ((y == 0 || y == 11) && (x >= 0 && x <= 11) ) { return 1; } else { return 0; }} void displayMaze(char maze [] [12]){ int x ; int y ; for (x = 0; x < 12 ; x++ ) { for (y = 0; y < 12 ; y++) { printf ( " %c ", maze[x] [y]); } printf("\n"); } printf("\n hit the return key to get next move"); getchar();}}
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
100%
my code is getting errors and i cant figiure out why , can you check my code and help me fix the errors . written in c code. It needs to print out a maze and as you hit enter you go through the maze.
#include <stdio.h>
#include <stdlib.h>
#include <stdlib.h>
#define XSTART 2
#define YSTART 0
#define YSTART 0
#define DOWN 0
#define RIGHT 1
#define RIGHT 1
#define UP 2
#define LEFT 3
#define LEFT 3
void mazeTraverse(char maze [12][12],int xCor,int yCor,
int dir);
int checkForEdge(int x , int y);
int correctMoves (char maze[][12],int r , int c );
void displayMaze (char maze [][12]);
int dir);
int checkForEdge(int x , int y);
int correctMoves (char maze[][12],int r , int c );
void displayMaze (char maze [][12]);
int main (void)
{
char maze [12] [12] =
{{'#','#','#','#','#','#','#','#','#','#','#','#'},
{'#','.','.','.','#','.','.','.','.','.','.','#'},
{'.','.','#','.','#','.','#','#','#','#','.','#'},
{'#','#','#','.','#','.','.','.','.','#','.','#'},
{'#','.','.','.','.','#','#','#','.','#','.','.'},
{'#','#','#','#','.','#','.','#','.','#','.','#'},
{'#','.','.','#','.','#','.','#','.','#','.','#'},
{'#','#','.','#','.','#','.','#','.','#','.','#'},
{'#','.','.','.','.','.','.','.','.','#','.','#'},
{'#','#','#','#','#','#','.','#','#','#','.','#'},
{'#','.','.','.','.','.','.','#','.','.','.','#'},
{'#','#','#','#','#','#','#','#','#','#','#','#'}
};
};
mazeTraverse(maze,XSTART,YSTART,RIGHT);
return 0;
}
return 0;
}
void mazeTraverse(char maze[12] [12] , int xCor ,int yCor , int dir)
{
static int flag =0;
static int flag =0;
maze[xCor][yCor]='X';
displayMaze(maze);
displayMaze(maze);
if (checkForEdge(xCor,yCor) && xCor != XSTART && yCor != YSTART)
{
printf("\n successfully exited the maze\n\n");
return;
}
else if (xCor == XSTART && yCor == YSTART && flag == 1)
{
printf("\nArrived back to starting position.\n\n");
return ;
}
{
printf("\n successfully exited the maze\n\n");
return;
}
else if (xCor == XSTART && yCor == YSTART && flag == 1)
{
printf("\nArrived back to starting position.\n\n");
return ;
}
else
{
int counter;
int nextMove;
flag = 1;
{
int counter;
int nextMove;
flag = 1;
for ( nextMove = dir , counter=0; counter < 4 ; ++counter,++nextMove, nextMove %= 4)
{
switch (nextMove)
{
{
switch (nextMove)
{
case DOWN:
if(correctMove (maze, xCor + 1, yCor ))
{
mazeTraverse(maze,xCor + 1,yCor,LEFT);
return;
}
{
mazeTraverse(maze,xCor + 1,yCor,LEFT);
return;
}
break;
case RIGHT :
if ( correctMove(maze,xCor,yCor + 1))
{
mazeTraverse(maze, xCor,yCor + 1 , DOWN);
return ;
}
if ( correctMove(maze,xCor,yCor + 1))
{
mazeTraverse(maze, xCor,yCor + 1 , DOWN);
return ;
}
break;
case UP:
if (correctMove(maze, xCor - 1,yCor))
{
mazeTraverse(maze, xCor - 1 , yCor,RIGHT);
return;
}
{
mazeTraverse(maze, xCor - 1 , yCor,RIGHT);
return;
}
break;
case LEFT :
if(correctMove(maze, xCor,yCor - 1))
{
mazeTraverse( maze , xCor,yCor -1, UP);
return;
}
{
mazeTraverse( maze , xCor,yCor -1, UP);
return;
}
break;
}
}
}
}
}
int correctMove(char maze [][12],int r , int c )
{
return(r>=0 && r <= 11 && c>= 0 && c <= 11 && maze[r][c] != '#' );
}
int checkForEdge(int x , int y )
{
if ((x == 0 || x == 11)&& (y >= 0 && y <= 11))
{
return 1;
}
else if ((y == 0 || y == 11) && (x >= 0 && x <= 11) )
{
return 1;
}
else
{
return 0;
}
}
{
if ((x == 0 || x == 11)&& (y >= 0 && y <= 11))
{
return 1;
}
else if ((y == 0 || y == 11) && (x >= 0 && x <= 11) )
{
return 1;
}
else
{
return 0;
}
}
void displayMaze(char maze [] [12])
{
int x ;
{
int x ;
int y ;
for (x = 0; x < 12 ; x++ )
{
for (y = 0; y < 12 ; y++)
{
printf ( " %c ", maze[x] [y]);
}
printf("\n");
}
printf("\n hit the return key to get next move");
{
for (y = 0; y < 12 ; y++)
{
printf ( " %c ", maze[x] [y]);
}
printf("\n");
}
printf("\n hit the return key to get next move");
getchar();
}
}
}
}
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
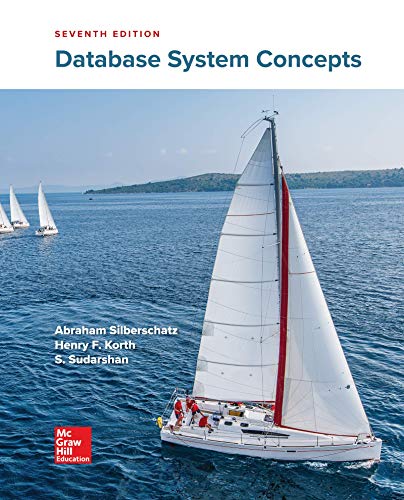
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
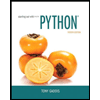
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
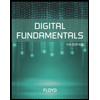
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
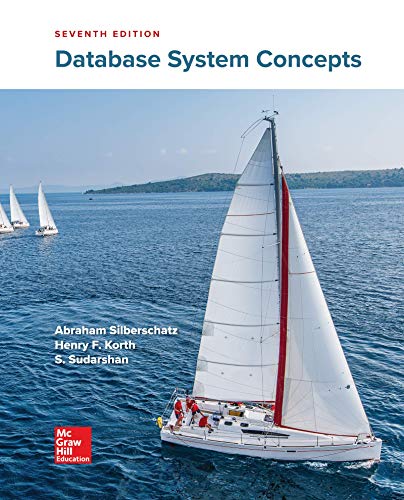
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
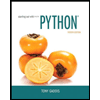
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
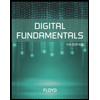
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
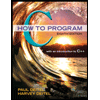
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
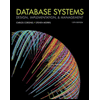
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
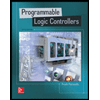
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education