n this assignment, we will be creating an HTML Checker program that will check the syntax of HTML tags. You will write a class that stores the contents of an HTML page as a queue of HTMLTags. Your class will also be able to fix invalid HTML tags. Your HTMLManager will use stacks and queues to figure out whether the tags match and fix the mistakes it finds. There will also be a number of Instructor-provided files to help with this assignment in order to make your work a little less :-)
Types of Linked List
A sequence of data elements connected through links is called a linked list (LL). The elements of a linked list are nodes containing data and a reference to the next node in the list. In a linked list, the elements are stored in a non-contiguous manner and the linear order in maintained by means of a pointer associated with each node in the list which is used to point to the subsequent node in the list.
Linked List
When a set of items is organized sequentially, it is termed as list. Linked list is a list whose order is given by links from one item to the next. It contains a link to the structure containing the next item so we can say that it is a completely different way to represent a list. In linked list, each structure of the list is known as node and it consists of two fields (one for containing the item and other one is for containing the next item address).
Overview:
In this assignment, we will be creating an HTML Checker program that will check the syntax of HTML tags.
You will write a class that stores the contents of an HTML page as a queue of HTMLTags. Your class will also be able to fix invalid HTML tags. Your HTMLManager will use stacks and queues to figure out whether the tags match and fix the mistakes it finds.
There will also be a number of Instructor-provided files to help with this assignment in order to make your work a little less :-)
Topics:
queues, stacks
Background on HTML:
Web pages are written in a language called “Hypertext Markup Language”, or HTML. An HTML file consists of text surrounded by markings called tags. Tags give information to the text, such as formatting (bold, italic, etc) or layout (paragraph, table, list, etc). Some tags specify comments or information about the document (header, title, document type).
A tag consists of a named element between less-than < and greater-than > symbols. For example, the tag for making text bold uses the element b and is written as <b>.
Many tags apply to a range of text, in which case a pair of tags is used:
- An opening tag (indicating the start of the range), which is written as: <tagname>
- A closing tag (indicating the end of the range), which is written as: </tagname>
So to make some text bold on a page, one would surround the text with opening and closing b tags:
- HTML Code: <b>like this</b>
- Displayed on screen: like this
Tags can be nested to combine effects. For example:
- HTML Code: <b><i>like this</i></b>
- Displayed on screen: like this
Some tags, such as the br tag (which inserts a line break) or the img (which inserts an image), do not cover a range of text and are considered to be “self-closing.” Self-closing tags do not need a closing tag; for a line break, only <br> is needed. Some web developers write self-closing tags with an optional / before the >, such as <br />.
Checking HTML:
One problem on the web is that many developers make mistakes in their HTML code. All tags that cover a range must eventually be closed, but some developers forget to close their tags. Also, whenever a tag is nested inside another tag, <b><i>like this</i></b>, the inner tag (i for italic, here) must be closed before the outer tag is closed. So the following tags are not valid HTML, because the </i> should appear first: <b><i>this is invalid</b></i>
This might sound like a lot if you are unfamiliar with HTML, but Don't stress! You will be provided with an
Instructions
Part 1: Get the starter files:
For this assignment, you are provided with a number of starter files: HTMLChecker.zip Download HTMLChecker.zip
- The only file that you will edit for this assignment is HTMLManager.java
- The only file that you will run is HTMLChecker.java
- The tests/ folder contains a few test HTML files
- The expected_output/ folder contains the expected "fixed" HTML for the associated test files - these are called and compared in the HTMLChecker.java file
- The other files you will mostly just ignore as they are used from the HTMLChecker, except for HTMLTag. The file HTMLTag.java you will want to study.
The HTMLTag.java file:
There is a provided file called HTMLTag.java which is the base type for your Queue (and the Stack that you will create in fixHTML), so it is fundamental that you know what methods it contains, though you will not edit it.
An HTMLTag object corresponds to an HTML tag such as <p> or </table>. An HTMLTag can either be an opening tag, a closing tag, or a self-closing tag. You don’t need to construct HTMLTag objects in your code, but you will process them. The reason we use HTMLTag objects instead of just storing the tags as strings is that the class provides extra functionality that makes processing HTMLTags easier.
In particular, it provides the following methods:
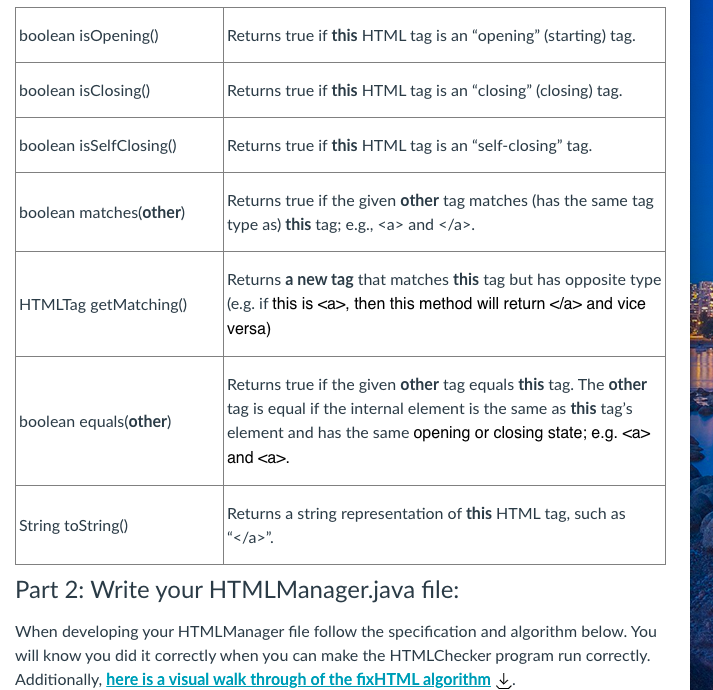
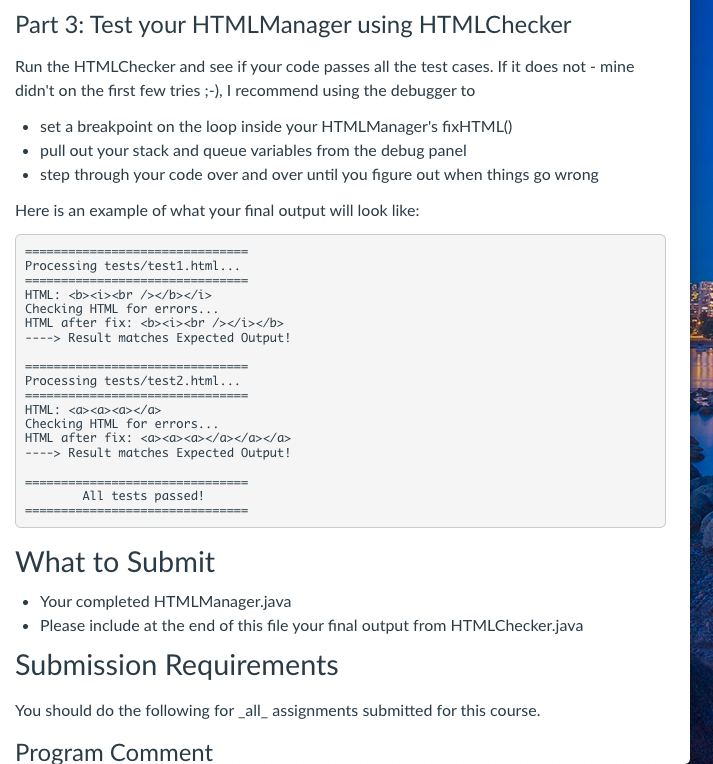

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

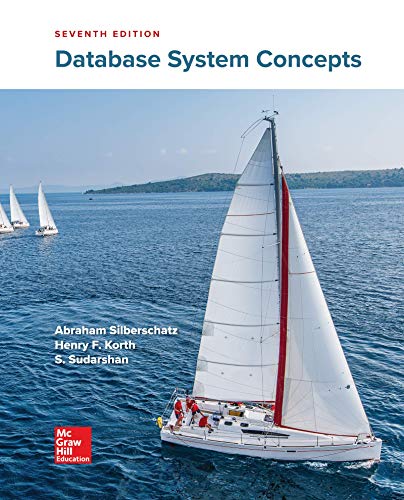
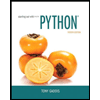
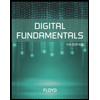
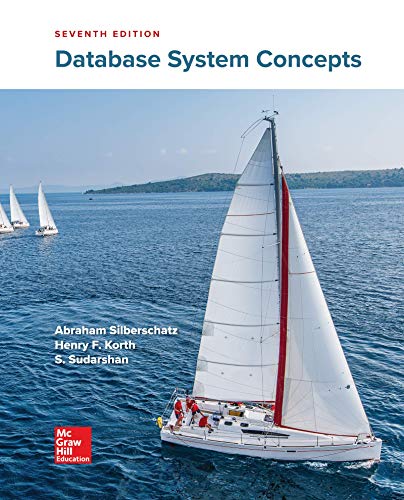
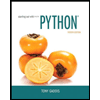
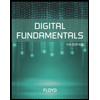
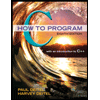
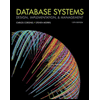
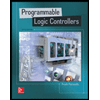