P2: Bucket Sort In previous years, midterm tests were written on paper. After marking all the tests, we would sort all the tests (by student last names) so that entering grades and handing back tests was more efficient. Here is how we would sort the tests. The first step is to create several piles of tests (which we'll call buckets). Each bucket (which is just a pile of tests) will correspond to all the students with last names in a given range. For example, we could make 26 buckets with each bucket holding all students with last names starting with one of the 26 different letters in the alphabet (that is, one bucket for all students with last name starting with "A", one for all students with last name starting with "B", etc.). We then sort each bucket (pile of exams) individually using something like insertion sort. Once each bucket is sorted, if the buckets are arranged in alphabetical order, we can then just pile each bucket on top of each other to have a completely sorted pile of exams. So, we take the "Y" bucket and put it on top of the "Z" bucket, then add the "X" bucket on top of that... until we finally add the "A" bucket to the very top. The end result is one pile of all tests in sorted order. If the names of the students in the class are evenly spread out across the alphabet this makes the sorting process fairly efficient. If everyone in the class has a last name starting with "J", for example, then this method is really bad... (unless we define our buckets differently). This is a form of a bucket sort. In general, a bucket sort does the following: • Create many buckets to store the data needed to be sorted o Each bucket wix hold values in a certain unique range that does not overlap with any other bucket o All values in the data to be sorted must be able to go into some bucket • Take each item in the collection to be sorted and put it in the appropriate bucket • Sort each individual bucket using some other sorting method (like insertion sort) • Combine all the sorted buckets (in the right order) to create one sorted colection of all the data Typically, a bucket sort is used to sort floating point numbers in a given range. Suppose we have a list of numbers where each number is in the range [0,1). That is, each number x satisfies 0sx<1. If the numbers in the list are spread out across this range fairly evenly, we can sort them very efficiently using the bucket sort.
In Python
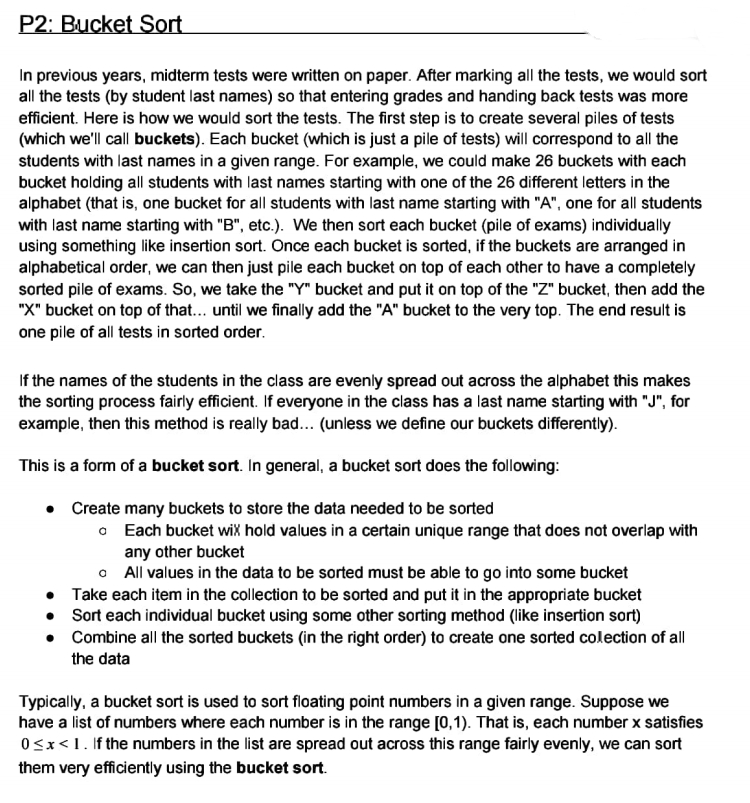
![In order to sort N values, each in the range [0,1), we create a secondary list of length N that will
store our buckets. Each bucket is just a list of numbers (hopefully very small; many will be
empty). Each bucket will be used to store numbers in a given sub-range. For example, the first
bucket will store all numbers in the range [0, 1/N), the second bucket will store all numbers in
the range [1/N, 2/N), ..., the last bucket will store all the numbers in the range [(N-1)/N, 1). So, if
there are N values in total, each bucket will store values in a subrange of size 1/N. No two
sub-ranges overlap at all.
The algorithm will iterate over the values we want to sort, adding each value to the correct
bucket in the bucket list. After all values are added to a bucket, we sort each bucket using
insertion sort. Once each bucket is sorted, we combine all the buckets back together (in the
right order) so that we have all the numbers in sorted order.
Write a function, called bucket_sort(numberList), that takes a list of numbers (floats) and
returns a new list with the same values in sorted order (from smalest to largest). The input list
must not be changed when the function ends.
For example,
>>> s = [0.1, 0.6, 0.4]
>>> sorteds = bucket_sort (S)
>>> S
[0.1, 0.6, 0.4]
>>> sorteds
[0.1, 0.4, 0.6]
Save your function in a file called "bucket.py".
Note: If the data is spread out randomly over the range [0,1), then the expected runtime of the
bucket sort is linear in the size of the list to be sorted. That is, it is expected to be O(N). This is
because we expect the number of elements in each bucket to be very small. We might get
unlucky though and one of the buckets might be very large which wik result in a much slower
sort time. If we use insertion sort for each bucket then the worst case runtime of the bucket sort
is quadratic. You will be able to prove the expected runtime of bucket sort after you have taken
COMP2804.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F8045ff8d-9d63-4990-8b6d-3a9291442d05%2F60f60727-d59d-49d9-afe0-16d1683841a7%2F0h7xvz_processed.png&w=3840&q=75)

python code:-
def insertionSort(b):
for i in range(1, len(b)):
up = b[i]
j = i - 1
while j >= 0 and b[j] > up:
b[j + 1] = b[j]
j -= 1
b[j + 1] = up
return b
def bucket_sort(x):
arr = []
slot_num = 10 # 10 means 10 slots, each
# slot's size is 0.1
for i in range(slot_num):
arr.append([])
# Put array elements in different buckets
for j in x:
index_b = int(slot_num * j)
arr[index_b].append(j)
# Sort individual buckets
for i in range(slot_num):
arr[i] = insertionSort(arr[i])
# concatenate the result
k = 0
for i in range(slot_num):
for j in range(len(arr[i])):
x[k] = arr[i][j]
k += 1
return x
# Driver Code
S = [0.1,0.6,0.4]
print("list before sorting",*S)
sortedS=bucket_sort(S)
print("list after sorting",*sortedS)
Step by step
Solved in 2 steps

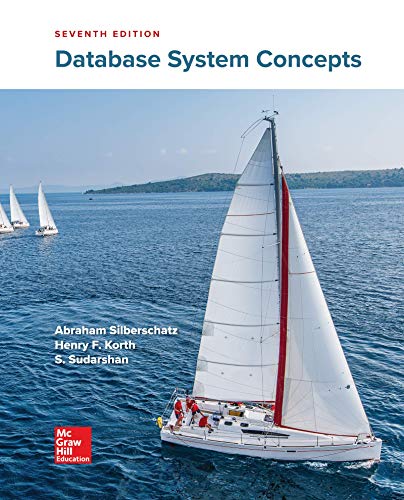
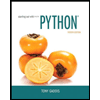
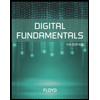
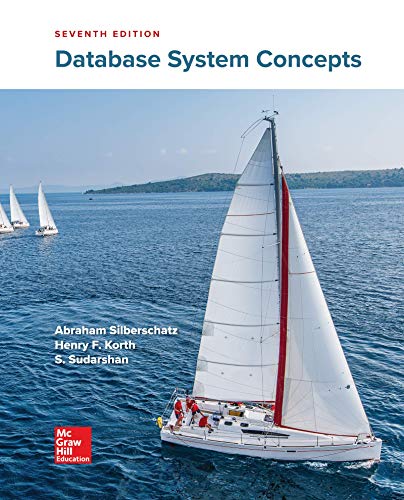
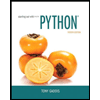
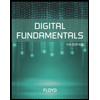
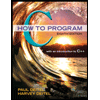
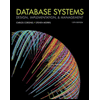
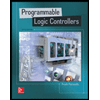