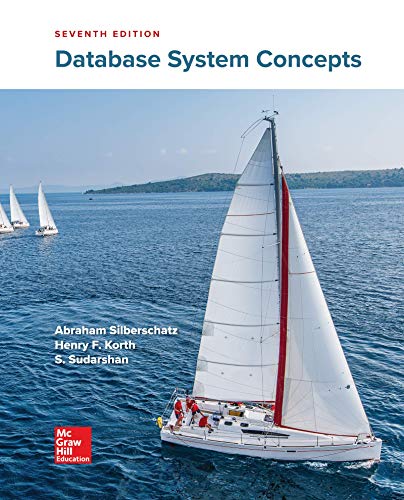
Concept explainers
Task 2 Get Binary 1:
Implement the get_bin_1 function as directed in the comment above the function. If the directions are unclear, run the code, and look at the expected answer for a few inputs. In order to be able to compare your solution to the answer, you cannot simply print out the binary directly to the console. Instead, you have been provided with a very rudimentary stringbuilder implementation that allows you to build a string one character at a time, without the ability to erase. There are comments at the top of the code explaining how to use the stringbuilder, however you could also see how it is being used in task_2_check.
Note: if you create a stringbuilder and append ‘a’, ‘b’, then ‘c’, the resulting string will be “abc” when printed. You are free to read through the stringbuilder implementation, but it is quite complicated in order to make it easy to use.
As specified in the comment, you may not use division (/) or mod (%) to implement this function (as the check function uses), and should instead rely on bitwise operations to read the underlying binary representation of the number. Recall how you implemented the is_negative function. Could you use something similar to figure out what each individual bit is, and build a string with it?
------- code
#include <stdio.h>
#include <stdbool.h>
#include <stdlib.h>
// BEGIN STRINGBUILDER IMPLEMENTATION
// This code is a very rudimentary stringbuilder-like implementation
// To create a new stringbuilder, use the following line of code
//
// stringbuilder sb = new_sb();
//
// If you want to append a character to the stringbuilder, use the
// following line of code. Replace whatever character you want to
// append where the 'a' is.
//
// sb_append_char(sb, 'a');
//
// Though there are some other functions that might be useful to you,
// the driver code provided uses the functions, so there is no need
// to use them manually.
typedef struct {
char** cars;
size_t* len;
size_t* alloc_size;
} stringbuilder;
stringbuilder new_sb() {
stringbuilder sb;
sb.cars = malloc(sizeof(char*));
*sb.cars = malloc(8*sizeof(char));
(*sb.cars)[0] = 0;
sb.len = malloc(sizeof(size_t));
*sb.len = 0;
sb.alloc_size = malloc(sizeof(size_t));
*sb.alloc_size = 8;
return sb;
}
void sb_append(stringbuilder sb, char a) {
int len = *sb.len;
if (len >= (*sb.alloc_size)-1) {
*sb.alloc_size = (*sb.alloc_size)*2;
char* newcars = malloc((*sb.alloc_size)*sizeof(char));
for (int i = 0; i < *sb.len; i++) {
newcars[i] = (*sb.cars)[i];
}
free(*sb.cars);
(*sb.cars) = newcars;
}
(*sb.cars)[len] = a;
len++;
(*sb.cars)[len] = 0;
*sb.len = len;
}
void delete_sb(stringbuilder sb) {
free(*sb.cars);
free(sb.cars);
free(sb.len);
free(sb.alloc_size);
}
bool sb_is_equal(stringbuilder sb1, stringbuilder sb2) {
if (*sb1.len != *sb2.len)
return false;
for (int i = 0; i < *sb1.len; i++) {
if ((*sb1.cars)[i] != (*sb2.cars)[i])
return false;
}
return true;
}
void print_sb(const stringbuilder sb) {
printf("%s", *sb.cars);
}
// END STRINGBUILDER IMPLEMENTATION
// ============================================================
// Write your solutions to the tasks below
const unsigned UNS_MAX = -1; // 1111...
const unsigned UNS_MIN = 0; // 0000...
const int INT_MAX = UNS_MAX >> 1; // 0111...
const int INT_MIN = ~INT_MAX; // 1000...
// Task 1
// For this function, you must return an integer holding the value
// x+1, however you may not use any constants or the symbol '+'
// anywhere in your solution. This means that
//
// return x - (-1);
//
// is not a valid soltion, because it uses the constant -1.
//
// Hint: Consider what internally happens when you do -x.
int plus_one(int x) {
return x;
}
// Task 2
// For this function, you must build a string that when printed,
// will output the entire binary representation of the integer x,
// no matter how many bits an integer is. You may NOT use
// division (/) or mod (%) anywhere in your code, and should
// instead rely on bitwise operations to read the underlying binary
// representation of x.
stringbuilder get_bin_1(int x) {
stringbuilder sb = new_sb();
sb_append(sb, '$');
return sb;
}

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 1 images

- Change this code to show strings that help you cast twelve dice. They should be shown one at a time like this:The first one was A. The second one was B. Afterward, the sum should be shown on a different line.arrow_forwardJava - Text Message Expanderarrow_forwardIn the classic problem FizzBuzz, you are told to print the numbers from 1 to n. However,when the number is divisible by 3, print "Fizz''. When it is divisible by 5, print "Buzz''. When it isdivisible by 3 and 5, print"FizzBuzz''. In this problem, you are asked to do this in a multithreaded way.Implement a multithreaded version of FizzBuzz with four threads. One thread checks for divisibilityof 3 and prints"Fizz''. Another thread is responsible for divisibility of 5 and prints"Buzz''. A third threadis responsible for divisibility of 3 and 5 and prints "FizzBuzz''. A fourth thread does the numbers.arrow_forward
- Assignment: Check the Sample run first, then carefully read the instructions and write aprogram that prompts the user for first name, last name, then secret number. The output willgenerate a default email address and a default password. 1. The main method will do the following:• Asks the user for first name, last name, and secret number using Scanner. (first lettercan be in upper case)• Call defaultInfo(String, String) method to PRINT default Kean email address.• Call defaultInfo(String, int) method to PRINT default Kean password.• Note: You are using overloaded methods (i.e., same method name with differentparameter lists) 2. Write an overloaded method, defaultInfo, which does the following:• public static void defaultInfo(String firstName, String lastName) [Note:you can use your own variable name]‒ print default email address all in low caps: concatenate first letter offirstName, full lastName, and @gmail.com. [Hint: string.charAt(index),string.toLowerCase()]• public static void…arrow_forwardpublic static String[] getTokens(String raw, String delimiter){ // Line #1 goes here... int numTokens = st.countTokens(); // Line #3 goes here... for(int i = 0; i < numTokens; i++){ // Line #5 goes here } // Line #7 goes here } Using the code above, which one of these should be the code for "Line #7": ret[i] = st.nextToken(); return ret; String[] ret = new String[numTokens]; StringTokenizer st = new StringTokenizer(raw, delimiter);arrow_forwardString spell = "Redikulus"; How would you find the index of the 'u' just before the last 's' using a single line of code? Multiple answers may be correct. Given the string String spell = "Redikulus"; How would you find the index of the 'u' just before the last 's' using a single line of code? Multiple answers may be correct. A. spell.substring(5, spell.length()).indexOf('u'); B. spell.indexOf('u', 5); C. spell.lastIndexOf('u'); D. spell.contains('u'); E. spell.indexOf('u', spell.length() - 2); F. spell.indexOf('u', spell.indexOf('l'));arrow_forward
- Assignment 5B: Keeping Score. Now that we know about arrays, we can keep track of when events occur during our program. To prove this, let's create a game of Rock-Paper-Scissors. For those not familiar with this game, the basic premise is that each player says "Rock-Paper- Scissors!" and then makes their hand into the shape of one of the three objects. Winners are determined based on the following rules: Rock beats Scissors Scissors beats Paper Paper beats Rock At the start of the program, it will ask the player how many rounds of Rock-Paper- Scissors they want to play. After this, the game will loop for that many number of times. Each loop, it will ask the player what item they want to use – Rock, Paper, or Scissors. The computer will randomly generate its own item, and a winner will be determined. The game will then save the result as an element of an array, and the next round will begin. Once all the rounds have been played, the program will say "Game Over" and display a list of who…arrow_forwardEx: Use getline() to get a line of user input into a string. Output the line. Enter text: IDK if I'll go. It's my BFF's birthday. You entered: IDK if I'll go. It's my BFF's birthday. Search the string (using find()) for common abbreviations and print a list of each found abbreviation along with its decoded meaning. Ex: Enter text: IDK if I'll go. It's my BFF's birthday. You entered: IDK if I'll go. It's my BFF's birthday. BFF: best friend forever IDK: I don't know Support these abbreviations: BFF -- best friend forever IDK -- I don't know . JK -- just kidding TMI -- too much information TTYL-talk to you later 1 #include 2 // FIXME include the string Library 3 using namespace std; 4 5 int main() { 6 7 8 9 10 } /* Type your code here. */ return 0; main.cpparrow_forwardPrimeAA.java Write a program that will tell a user if their number is prime or not. Your code will need to run in a loop (possibly many loops) so that the user can continue to check numbers. A prime is a number that is only divisible by itself and the number 1. This means your code should loop through each value between 1 and the number entered to see if it’s a divisor. If you only check for a small handful of numbers (such as 2, 3, and 5), you will lose most of the credit for this project. Include a try/catch to catch input mismatches and include a custom exception to catch negative values. If the user enters 0, the program should end. Not only will you tell the user if their number is prime or not, you must also print the divisors to the screen (if they exist) on the same line as shown below AND give a count of how many divisors there are. See examples below. Your program should run the test case exactly as it appears below, and should work on any other case in general. Output…arrow_forward
- You will be reading in a series of names from the keyboard. Count how many matching pairs there are – a matching pair is when two consecutive names are equal regardless of their case - and print the pairs. Input stops when the word ‘end’ is entered (also ignoring case). For example, Jack Jose jose Faith Robert Cynthia cynthia Cynthia Pierre ENd would print: Jose jose Cynthia cynthia cynthia Cynthia There were 3 matching pairs.arrow_forwardJAVA PPROGRAM ASAP Please create this program ASAP BECAUSE IT IS MY LAB ASSIGNMENT #2 so it passes all the test cases. The program must pass the test case when uploaded to Hypergrade. Chapter 9. PC #8. 8. Sum of Numbers in a String (page 610) Write a program that asks the user to enter a series of numbers separated by commas. Here are two examples of valid input: 7,9,10,2,18,6 8.777,10.9,11,2.2,-18,6 The program should calculate and display the sum of all the numbers. Input Validation 1. Make sure the string only contains numbers and commas (valid symbols are '0'-'9', '-', '.', and ','). 2. If the string is empty, a messages should be displayed, saying that the string is empty. Test Case 1 Enter a series of numbers separated only by commas or type QUIT to exit:\n7,9,10,2,18,6ENTERThe sum of those numbers is 52.00\nEnter a series of numbers separated only by commas or type QUIT to exit:\nENTEREmpty string.\nEnter a series of numbers separated only by…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
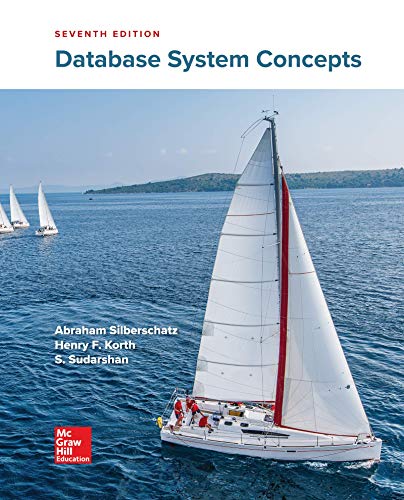
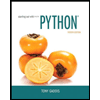
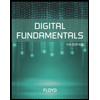
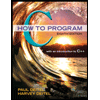
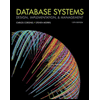
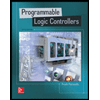