please answer correctly!! Function Growth package functiongrowth; import java.util.Scanner; public class FunctionGrowth { private long start = 0; public FunctionGrowth() { start = System.currentTimeMillis(); } public double elapsedTime() { long now = System.currentTimeMillis(); return (now - start) / 1000.0; } private static long constant_value(long paramInt) { long l = 0L; for (int i = 0; i < paramInt; i++) l += 1L; return l; } private static long logarithmic(long paramInt1, long paramInt2) { long l = 0L; for (int i = 1; i + i <= paramInt1; i += i) l += constant_value(paramInt2); return l; } private static long sqrt(long paramInt1, long paramInt2) { long l = 0L; int i = 0; for (int j = 0; j < paramInt1; i++) { l += constant_value(paramInt2);j += i; } return l; } public static long linearithmic(long paramInt1, long paramInt2) { if (paramInt1 == 0) return 0L; long l = 0L; for (int i = 0; i < paramInt1; i++) l += constant_value(paramInt2); return linearithmic(paramInt1 / 2, paramInt2) + l + linearithmic(paramInt1 / 2, paramInt2); } private static long linear(long paramInt1, long paramInt2) { long l = 0L; for (int i = 0; i < paramInt1; i++) l += constant_value(paramInt2); return l; } private static long linearsqrt(long paramInt1, long paramInt2) { long l = 0L; for (int i = 0; i < paramInt1; i++) { l += sqrt(paramInt1, paramInt2); } return l; } private static long quadratic(long paramInt1, long paramInt2) { long l = 0L; for (int i = 0; i < paramInt1; i++) for (int j = 0; j < paramInt1; j++) l += constant_value(paramInt2); return l; } private static long exponential(long paramInt1, long paramInt2) { if (paramInt1 == 0) return constant_value(paramInt2); return exponential(paramInt1 - 1, paramInt2) + exponential(paramInt1 - 1, paramInt2); } private static long factorial(long paramInt1, long paramInt2) { if (paramInt1 == 0) return constant_value(paramInt2); long l = 0L; for (int i = 0; i < paramInt1; i++) l += factorial(paramInt1 - 1, paramInt2); return l; } public static long Order(long N) { long num = 0L; int sum = 0; for(int i=0; i < N; i++) { for(int j = 1; j <= N*N; j=j*2 ) { sum++; } } num=sum; return num; } static Scanner input = new Scanner(System.in); public static void main(String[] args) { long value = 0L; value = Order(constant_value(5)); System.out.println("Constant Result: " +value); value = Order(logarithmic(5,10)); System.out.println("Logarithmic Result: " +value); value = Order(sqrt(5,10)); System.out.println("Sqrt Result: " +value); value = Order(linearithmic(5,10)); System.out.println("Linearithmic Result: " +value); value = Order(linear(5,10)); System.out.println("Linear Result: " +value); value = Order(linearsqrt(5,10)); System.out.println("Linear Sqrt Result: " +value); value = Order(quadratic(5,10)); System.out.println("Quadratic Result: " +value); value = Order(exponential(5,10)); System.out.println("Exponential Result: " +value); value = Order(factorial(5,10)); System.out.println("Factorial Result: " +value); } }
please answer correctly!!
Function Growth
package functiongrowth;
import java.util.Scanner;
public class FunctionGrowth {
private long start = 0;
public FunctionGrowth() {
start = System.currentTimeMillis();
}
public double elapsedTime() {
long now = System.currentTimeMillis();
return (now - start) / 1000.0;
}
private static long constant_value(long paramInt) {
long l = 0L;
for (int i = 0; i < paramInt; i++)
l += 1L;
return l;
}
private static long logarithmic(long paramInt1, long paramInt2) {
long l = 0L;
for (int i = 1; i + i <= paramInt1; i += i)
l += constant_value(paramInt2);
return l;
}
private static long sqrt(long paramInt1, long paramInt2) {
long l = 0L;
int i = 0; for (int j = 0; j < paramInt1; i++) {
l += constant_value(paramInt2);j += i;
}
return l;
}
public static long linearithmic(long paramInt1, long paramInt2) {
if (paramInt1 == 0) return 0L;
long l = 0L;
for (int i = 0; i < paramInt1; i++)
l += constant_value(paramInt2);
return linearithmic(paramInt1 / 2, paramInt2) + l + linearithmic(paramInt1 / 2, paramInt2);
}
private static long linear(long paramInt1, long paramInt2) {
long l = 0L;
for (int i = 0; i < paramInt1; i++)
l += constant_value(paramInt2);
return l;
}
private static long linearsqrt(long paramInt1, long paramInt2) {
long l = 0L;
for (int i = 0; i < paramInt1; i++) {
l += sqrt(paramInt1, paramInt2);
}
return l;
}
private static long quadratic(long paramInt1, long paramInt2) {
long l = 0L;
for (int i = 0; i < paramInt1; i++)
for (int j = 0; j < paramInt1; j++)
l += constant_value(paramInt2);
return l;
}
private static long exponential(long paramInt1, long paramInt2) {
if (paramInt1 == 0) return constant_value(paramInt2);
return exponential(paramInt1 - 1, paramInt2) + exponential(paramInt1 - 1, paramInt2);
}
private static long factorial(long paramInt1, long paramInt2) {
if (paramInt1 == 0) return constant_value(paramInt2);
long l = 0L;
for (int i = 0; i < paramInt1; i++)
l += factorial(paramInt1 - 1, paramInt2);
return l;
}
public static long Order(long N) {
long num = 0L;
int sum = 0;
for(int i=0; i < N; i++) {
for(int j = 1; j <= N*N; j=j*2 ) {
sum++;
}
}
num=sum;
return num;
}
static Scanner input = new Scanner(System.in);
public static void main(String[] args) {
long value = 0L;
value = Order(constant_value(5));
System.out.println("Constant Result: " +value);
value = Order(logarithmic(5,10));
System.out.println("Logarithmic Result: " +value);
value = Order(sqrt(5,10));
System.out.println("Sqrt Result: " +value);
value = Order(linearithmic(5,10));
System.out.println("Linearithmic Result: " +value);
value = Order(linear(5,10));
System.out.println("Linear Result: " +value);
value = Order(linearsqrt(5,10));
System.out.println("Linear Sqrt Result: " +value);
value = Order(quadratic(5,10));
System.out.println("Quadratic Result: " +value);
value = Order(exponential(5,10));
System.out.println("Exponential Result: " +value);
value = Order(factorial(5,10));
System.out.println("Factorial Result: " +value);
}
}


Step by step
Solved in 6 steps with 3 images

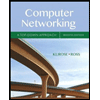
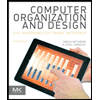
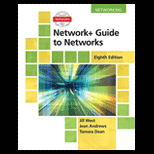
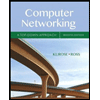
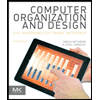
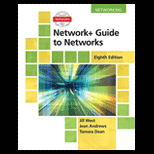
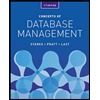
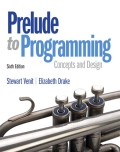
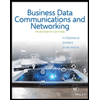