Driver class CarInLine: import java.io.*; class CarInLine{ static int ctr=0; static int cars,cash; static int DepartureTime=0; static int arrivalTime=0; static int ctr_tax=0; CarInLine(){ ctr++; cars=0; cash=0; } void pcar(){ System.out.print(“\nEnter the amount of toll:”); cash=cash+cash; cars++; } void npcar(){ cars++; } void disp(){ System.out.print(” \n\Number of cashiers\n\n”); System.out.print(“\tAverage time\n\n”); System.out.print(“\Optimum number of cashiers is"); } } void tax(int a){ if(a==1) ctr_tax++; } } class booth{ public static void main(String arg[]){ DataInputStream ins=new DataInputStream(System.in); int ans=1,ncar=5; do{ CarInLine c=new car(); try{ System.out.print("Press 1 if u want to pay tax "); ans=Integer.parseInt(ins.readLine()); c.tax(ans); System.out.print("Another car? Press 5 "); ncar=Integer.parseInt(ins.readLine()); } catch(IOException e){ } } while(ncar==5); System.out.println("Average Procesing time: "+car.ctr); int non = car.ctr - car.ctr_tax; System.out.println("No. of cars which have not paid tax: "+non); int amt = car.ctr_tax*50; System.out.println("Amount of cash collected: "+amt); } } Define ten queues, simulating the functionality of the process, increasing the number of cashiers from one, and collecting the average waiting time for each scenario. Each simulation will work with the same number of cars, which is considered 100. The maximum number of cashiers/toll booths is 10. Create the queue with link-based implementation. Create each queue with the corresponding number of cashiers, from 1 to 10, and record the average processing time. Save the processing time in an array of integer values representing the processing time. At the end of the simulation, display the results in a table with the number of cashiers and the average waiting time, measured in seconds. Choose the optimum number of cashiers, considering that the desired wait time is 1.5 minutes (90 seconds) Display the result of your simulation, which is the optimum number of cashiers. If you implement all the required methods correctly, the driver program should generate outputs similar to the following
Driver class CarInLine: import java.io.*; class CarInLine{ static int ctr=0; static int cars,cash; static int DepartureTime=0; static int arrivalTime=0; static int ctr_tax=0; CarInLine(){ ctr++; cars=0; cash=0; } void pcar(){ System.out.print(“\nEnter the amount of toll:”); cash=cash+cash; cars++; } void npcar(){ cars++; } void disp(){ System.out.print(” \n\Number of cashiers\n\n”); System.out.print(“\tAverage time\n\n”); System.out.print(“\Optimum number of cashiers is"); } } void tax(int a){ if(a==1) ctr_tax++; } } class booth{ public static void main(String arg[]){ DataInputStream ins=new DataInputStream(System.in); int ans=1,ncar=5; do{ CarInLine c=new car(); try{ System.out.print("Press 1 if u want to pay tax "); ans=Integer.parseInt(ins.readLine()); c.tax(ans); System.out.print("Another car? Press 5 "); ncar=Integer.parseInt(ins.readLine()); } catch(IOException e){ } } while(ncar==5); System.out.println("Average Procesing time: "+car.ctr); int non = car.ctr - car.ctr_tax; System.out.println("No. of cars which have not paid tax: "+non); int amt = car.ctr_tax*50; System.out.println("Amount of cash collected: "+amt); } } Define ten queues, simulating the functionality of the process, increasing the number of cashiers from one, and collecting the average waiting time for each scenario. Each simulation will work with the same number of cars, which is considered 100. The maximum number of cashiers/toll booths is 10. Create the queue with link-based implementation. Create each queue with the corresponding number of cashiers, from 1 to 10, and record the average processing time. Save the processing time in an array of integer values representing the processing time. At the end of the simulation, display the results in a table with the number of cashiers and the average waiting time, measured in seconds. Choose the optimum number of cashiers, considering that the desired wait time is 1.5 minutes (90 seconds) Display the result of your simulation, which is the optimum number of cashiers. If you implement all the required methods correctly, the driver program should generate outputs similar to the following
Chapter9: Working With Text Functions And Creating Custom Formats
Section: Chapter Questions
Problem 3RA
Related questions
Question
Written in java,
Your Tasks:
- Driver class CarInLine:
- import java.io.*;
class CarInLine{
static int ctr=0;
static int cars,cash;
static int DepartureTime=0;
static int arrivalTime=0;
static int ctr_tax=0;
CarInLine(){
ctr++;
cars=0;
cash=0;
}
void pcar(){
System.out.print(“\nEnter the amount of toll:”);
cash=cash+cash;
cars++;
}
void npcar(){
cars++;
}
void disp(){
System.out.print(” \n\Number of cashiers\n\n”);
System.out.print(“\tAverage time\n\n”);
System.out.print(“\Optimum number of cashiers is");
}
}
void tax(int a){
if(a==1)
ctr_tax++;
}
}
class booth{
public static void main(String arg[]){
DataInputStream ins=new DataInputStream(System.in);
int ans=1,ncar=5;
do{
CarInLine c=new car();
try{
System.out.print("Press 1 if u want to pay tax ");
ans=Integer.parseInt(ins.readLine());
c.tax(ans);
System.out.print("Another car? Press 5 ");
ncar=Integer.parseInt(ins.readLine());
}
catch(IOException e){
}
}
while(ncar==5);
System.out.println("Average Procesing time: "+car.ctr);
int non = car.ctr - car.ctr_tax;
System.out.println("No. of cars which have not paid tax: "+non);
int amt = car.ctr_tax*50;
System.out.println("Amount of cash collected: "+amt);
}
}
- import java.io.*;
- Define ten queues, simulating the functionality of the process, increasing the number of cashiers from one, and collecting the average waiting time for each scenario.
- Each simulation will work with the same number of cars, which is considered 100.
- The maximum number of cashiers/toll booths is 10.
- Create the queue with link-based implementation.
- Create each queue with the corresponding number of cashiers, from 1 to 10, and record the average processing time.
- Save the processing time in an array of integer values representing the processing time.
- At the end of the simulation, display the results in a table with the number of cashiers and the average waiting time, measured in seconds.
- Choose the optimum number of cashiers, considering that the desired wait time is 1.5 minutes (90 seconds)
- Display the result of your simulation, which is the optimum number of cashiers.
- If you implement all the required methods correctly, the driver
program should generate outputs similar to the following:

Transcribed Image Text:Number of cashiers:
Average time:
Optimum number of cashiers is: 9
Average processing time: 90
Press any key to continue..
1
2
3
4
5
6
7
9
10
4050
1805
1060
690
470
325
223
147
90
90
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
Np Ms Office 365/Excel 2016 I Ntermed
Computer Science
ISBN:
9781337508841
Author:
Carey
Publisher:
Cengage
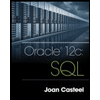
Np Ms Office 365/Excel 2016 I Ntermed
Computer Science
ISBN:
9781337508841
Author:
Carey
Publisher:
Cengage
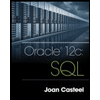