Please enter a string for an anagram: opt Matching word opt Matching word pot Matching word top Please enter a string for an anagram: blah No matches found Requirements You must write these three functions with the exact same function signature (include case): int loadDictionary(istream &dictfile, vector& dict); Places each string in dictfile into the vector dict. Returns the number of words loaded into dict. int permute (string word, vector& dict, vector& results); Places all the permutations of word, which are found in dict into results. Returns the number of matched words found. void display (vector& results); Displays size number of strings from results. The results can be printed in any order. For words with double letters you may find that different permutations match the same word in the dictionary. For example, if you find all the permutations of the string kloo using the algorithm we've discussed you may find that the word look is found twice. The o's in kloo take turns in front. Your program should ensure that matches are unique, in other words, the results array returned from the permute function should have no duplicates.
Please enter a string for an anagram: opt Matching word opt Matching word pot Matching word top Please enter a string for an anagram: blah No matches found Requirements You must write these three functions with the exact same function signature (include case): int loadDictionary(istream &dictfile, vector& dict); Places each string in dictfile into the vector dict. Returns the number of words loaded into dict. int permute (string word, vector& dict, vector& results); Places all the permutations of word, which are found in dict into results. Returns the number of matched words found. void display (vector& results); Displays size number of strings from results. The results can be printed in any order. For words with double letters you may find that different permutations match the same word in the dictionary. For example, if you find all the permutations of the string kloo using the algorithm we've discussed you may find that the word look is found twice. The o's in kloo take turns in front. Your program should ensure that matches are unique, in other words, the results array returned from the permute function should have no duplicates.
C++ for Engineers and Scientists
4th Edition
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Bronson, Gary J.
Chapter8: I/o Streams And Data Files
Section: Chapter Questions
Problem 8PP: (Data processing) A bank’s customer records are to be stored in a file and read into a set of arrays...
Related questions
Question
Please write the code for the loadDictionary, permute, and display functions. Will thumbs up if correct! :)
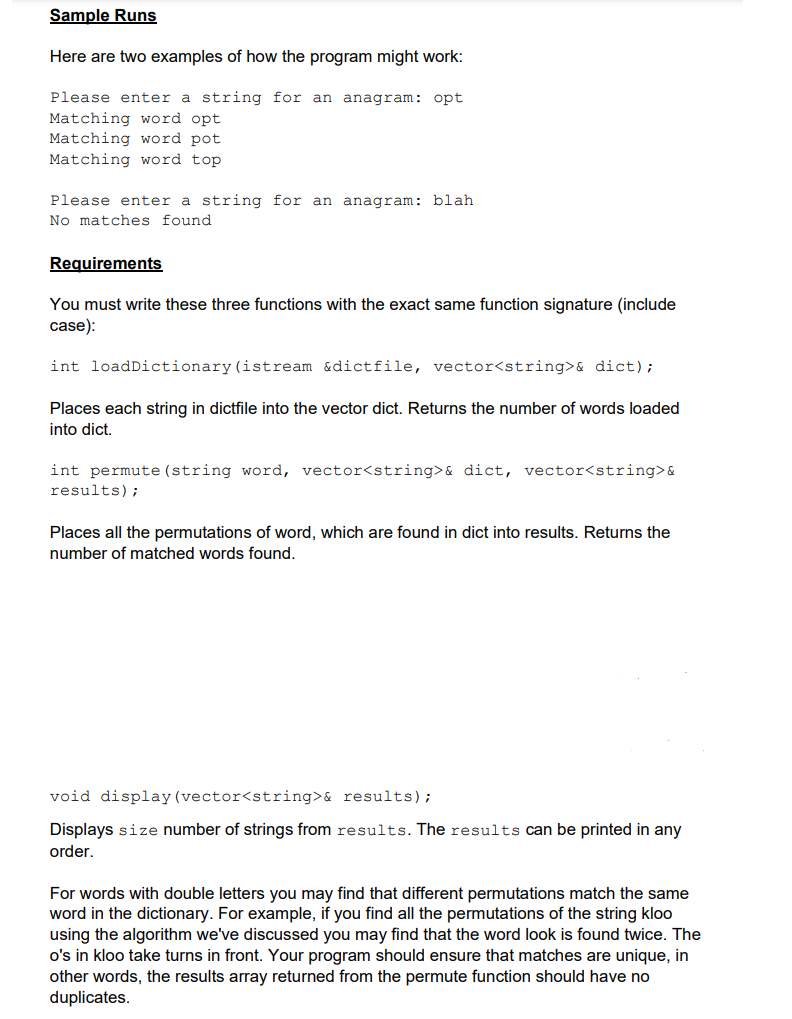
Transcribed Image Text:Sample Runs
Here are two examples of how the program might work:
Please enter a string for an anagram: opt
Matching word opt
Matching word pot
Matching word top
Please enter a string for an anagram: blah
No matches found
Requirements
You must write these three functions with the exact same function signature (include
case):
int loadDictionary(istream &dictfile, vector<string>& dict);
Places each string in dictfile into the vector dict. Returns the number of words loaded
into dict.
int permute (string word, vector<string>& dict, vector<string>&
results);
Places all the permutations of word, which are found in dict into results. Returns the
number of matched words found.
void display (vector<string>& results);
Displays size number of strings from results. The results can be printed in any
order.
For words with double letters you may find that different permutations match the same
word in the dictionary. For example, if you find all the permutations of the string kloo
using the algorithm we've discussed you may find that the word look is found twice. The
o's in kloo take turns in front. Your program should ensure that matches are unique, in
other words, the results array returned from the permute function should have no
duplicates.
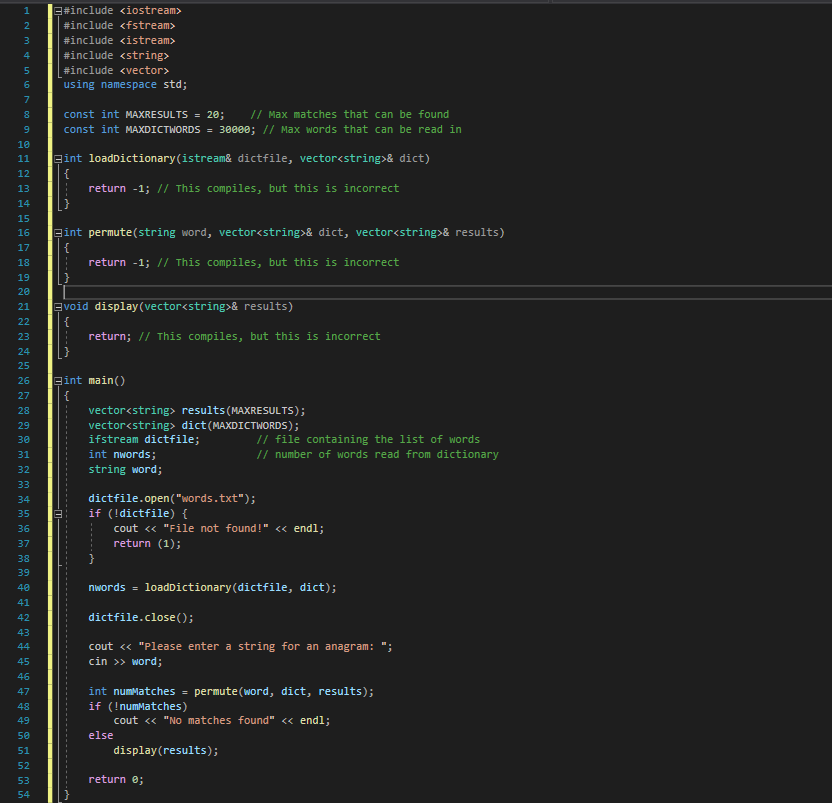
Transcribed Image Text:1
3#include <iostream>
#include <fstream>
#include <istream>
4
#include <string>
#include <vector>
using namespace std;
7
const int MAXRESULTS ▪ 20;
// Max matches that can be found
9.
const int MAXDICTWORDS = 30000; // Max words that can be read in
10
Bint loadDictionary(istream& dictfile, vector<string>& dict)
11
12
{
13
return -1; // This compiles, but this is incorrect
14
15
Bint permute(string word, vector<string>& dict, vector<string>& results)
16
17
{
18
return -1; // This compiles, but this is incorrect
19
20
Evoid display(vector<string>& results)
21
22
23
return; // This compiles, but this is incorrect
24
25
26
Bint main()
{
vector<string> results(MAXRESULTS);
vector<string> dict(MAXDICTWORDS);
ifstream dictfile;
int nwords;
27
28
29
// file containing the list of words
// number of words read from dictionary
30
31
32
string word;
33
dictfile.open("words.txt");
if (!dictfile) {
34
35
cout <« "File not found!" « endl;
return (1);
36
37
38
39
40
nwords = loadDictionary(dictfile, dict);
41
42
dictfile.close();
43
44
cout <« "Please enter a string for an anagram:
45
cin >> word;
46
int numMatches = permute (word, dict, results);
if (!numMatches)
47
48
49
cout « "No matches found" <« endl;
50
else
51
display(results);
52
53
return e;
54
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
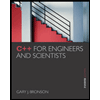
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage
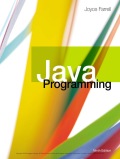
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
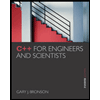
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage
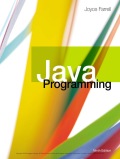
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT