Create organized functions with the single lines of code by declaring the functions, calling the function, then defining the function. Please help me make this look cleaner... The code should operate the same. C++ #include #include #include #include using namespace std; int main() { srand(static_cast (time(0))); const int N = 4; string wordP[N] = { "engineer", "cooper union", "final", "new york university" }; for (int i = 0; i <= N; i++) { cout << wordP[i]<< endl; } label: int z = rand() % N; string word = wordP[z]; string scrambled = word; const int rp = scrambled.size(); for (int letter = 0; letter < rp; letter++) { int z = rand() % rp; char game = scrambled[z]; scrambled[z] = scrambled[letter]; scrambled[letter] = game; } unsigned int t1 = time(0); string begin; do { cout << "Lets play a game.." << endl << " Unscramble the following words in order to proceed" << " " <> begin; if (begin == word) { cout << "Congratulations you beat the game" << endl; break; } } while (begin != "Quit"); unsigned int t2 = time(0); unsigned int score = (t2 - t1); cout << "It took " << score << "seconds" << endl; cout << " Thank you, come again!!" << endl; char approval; cout<<"Do you wish to play again (y for yes, n for no): "; cin>>approval; if(approval == 'y'){ cout<<"\n\n"; goto label; } else cout<<"\nExiting...."; return 0; }
Create organized functions with the single lines of code by declaring the functions, calling the function, then defining the function. Please help me make this look cleaner... The code should operate the same. C++
#include <iostream>
#include <vector>
#include <string>
#include <ctime>
using namespace std;
int main()
{
srand(static_cast<unsigned int> (time(0)));
const int N = 4;
string wordP[N] = { "engineer",
"cooper union", "final", "new york university" };
for (int i = 0; i <= N; i++) {
cout << wordP[i]<< endl;
}
label:
int z = rand() % N;
string word = wordP[z];
string scrambled = word;
const int rp = scrambled.size();
for (int letter = 0; letter < rp; letter++) {
int z = rand() % rp;
char game = scrambled[z];
scrambled[z] = scrambled[letter];
scrambled[letter] = game;
}
unsigned int t1 = time(0);
string begin;
do {
cout << "Lets play a game.." << endl << " Unscramble the following words in order to proceed" << " " <<endl<< "word:"<<" "<<scrambled << endl;
cin >> begin;
if (begin == word) {
cout << "Congratulations you beat the game" << endl;
break;
}
} while (begin != "Quit");
unsigned int t2 = time(0);
unsigned int score = (t2 - t1);
cout << "It took " << score << "seconds" << endl;
cout << " Thank you, come again!!" << endl;
char approval;
cout<<"Do you wish to play again (y for yes, n for no): ";
cin>>approval;
if(approval == 'y'){
cout<<"\n\n";
goto label;
}
else
cout<<"\nExiting....";
return 0;
}

Answer:
I have done code and also I have attached code and code screenshot as well as output
Step by step
Solved in 5 steps with 2 images

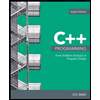
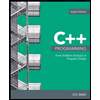