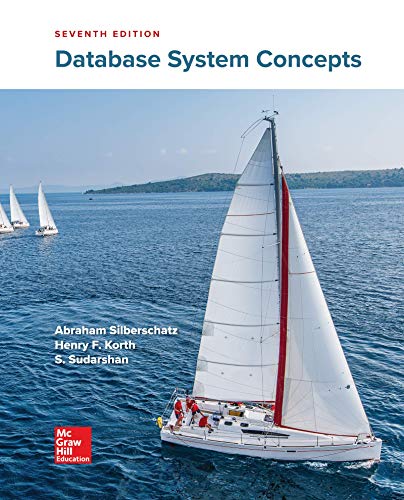
it says cannot convert string to char please help, it needs to be string. c++ i will include the source main and .h classes
//bt.cpp - file contains member functions
#include "bt.h"
#include <iostream>
using namespace std;
//Constructor
BT::BT()
{
root = NULL;
}
//Insert new item in BST
void BT::insert(string d)
{
node* t = new node;
node* parent;
t->data = d; //THE ERROR MESSAGE IS FROM HERE
t->left = NULL;
t->right = NULL;
parent = NULL;
if (isEmpty())
root = t;
else
{
//Note: ALL insertions are as leaf nodes
node* curr;
curr = root;
// Find the Node's parent
while (curr)
{
parent = curr;
if (t->data > curr->data)
curr = curr->right;
else
curr = curr->left;
}
if (t->data < parent->data)
parent->left = t;
else
parent->right = t;
}
}
___________________________________________________________________________________________
#include "bt.h"
#include<string>
#include <iostream>
using namespace std;
int main()
{
BT b;
int ch, num;
string d;
cout << "-----------Menu-------------" << endl;
cout << endl;
cout << "1. Insert node(s)" << endl;
cout << "2. Traverse Preorder" << endl;
cout << "3. Traverse Inorder" << endl;
cout << "4. Traverse Inorde" << endl;
cout << "5. Quit" << endl << endl;
do
{
cout << endl << "Enter Your Choice <1 - 5> ";
cin >> ch;
switch (ch)
{
case 1:
cout << "Enter number of nodes to insert: ";
cin >> num;
for (int i = 0; i<num; i++)
{
cout << endl;
cout << "Enter node: ";
cin >> d;
//b.insert(toupper(d));
cout << "Inserted." << endl;
}
____________________________________________________________________________________________
//bt.h - header file contains the object description
#include <iostream>
#ifndef BT_H
#define BT_H
using namespace std;
class BT
{
private:
struct node
{
char data; //whatever is the data type; use that
node* left;
node* right;
};
node* root;
public:
BT(); //Constructor
bool isEmpty() const { return root == NULL; } //Check for empty
void insert(string); //Insert item in BST
}

Step by stepSolved in 2 steps

- please use DEQUE #include <iostream>#include <string>#include <deque> using namespace std; const int AIRPORT_COUNT = 12;string airports[AIRPORT_COUNT] = {"DAL","ABQ","DEN","MSY","HOU","SAT","CRP","MID","OKC","OMA","MDW","TUL"}; int main(){// define stack (or queue ) herestring origin;string dest;string citypair;cout << "Loading the CONTAINER ..." << endl;// LOAD THE STACK ( or queue) HERE// Create all the possible Airport combinations that could exist from the list provided.// i.e DALABQ, DALDEN, ...., ABQDAL, ABQDEN ...// DO NOT Load SameSame - DALDAL, ABQABQ, etc .. cout << "Getting data from the CONTAINER ..." << endl;// Retrieve data from the STACK/QUEUE here } Using the attached shell program (AirportCombos.cpp), create a list of strings to process and place on a STL DEQUE container. Using the provided 3 char airport codes, create a 6 character string that is the origin & destination city pair. Create all the possible…arrow_forwardC++arrow_forwardExercise2: Thestrcmp(string1,string2)function comparesstring1to string2. It is a value returning function that returns a negative integer if string1 < string2, 0 if string1 == string2, and a positive integer if string1 > string2. Write a program that reads two names (last name first followed by a comma followed by the first name) and then prints them in alphabetical order. The two names should be stored in separate character arrays holding a maximum of 25 characters each. Use the strcmp() func- tion to make the comparison of the two names. Remember that 'a' < 'b', 'b' < 'c', etc. Be sure to include the proper header file to use strcmp(). Sample Run 1: Please input the first name Brown, George Please input the second name Adams, Sally The names are as follows: Adams, SallyBrown, George Sample Run 2: Please input the first name Brown, George Please input the second name Brown, George The names are as follows: Brown, GeorgeBrown, GeorgeThe names are the samearrow_forward
- # dates and times with lubridateinstall.packages("nycflights13") library(tidyverse)library(lubridate)library(nycflights13) Qustion: Create a function called date_quarter that accepts any vector of dates as its input and then returns the corresponding quarter for each date Examples: “2019-01-01” should return “Q1” “2011-05-23” should return “Q2” “1978-09-30” should return “Q3” Etc. Use the flight's data set from the nycflights13 package to test your function by creating a new column called quarter using mutate()arrow_forwardIN C++ Write code that: creates 3 integer values - one can be set to 0, the other two should NOT multiples of one another (for example 3 and 6, or 2 and 8) take you largest value and perform a modulus operation using the smaller (non zero) value as the divisor print out the result. create an alias for the string type call the alias "name" then create an instance of the "name" class and assign it a value using an appropriate cout statement - print out the value Please screenshot your input and output, as the format tends to get messed up. Thank you!arrow_forwardC++ help with this fixing code .cpp file #include<iostream>#include<string>#include<vector>#include"Food.h"using namespace std; class Nutrition{ private: string name; double Calories double Fat double Sugars double Protein double Sodium Nutrition(string n, double c, double f, double s, double p, double S): name(n), Calories(c), Fat(f),Sugars(s), Protein(p),Sodium(S){} food[Calories]= food[Fat]=food[Sugars]=food[Protein]=food[Sodium]=0; name = "";} Nutrition(string type, double calories, double fat, double sugar,double protein, double sodium){ food[Calories]= calories; food[Fat]=fat; food[Sugars]= sugar; food[Protein]=protein; food[Sodium]=sodium; name= type; } void setName(string type){ name = type; } void setCalories(double calories){ food [Calories]= calories; } void setFat(double fat){ food [Fat]= fat; } void setSugars(double sugar){ food [Sugars]= sugar; } void setProtein(double protein){ food [Protein]= protein; } void…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
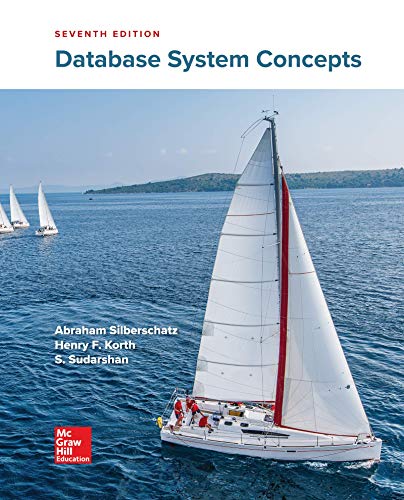
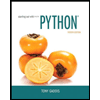
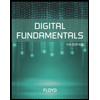
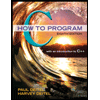
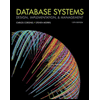
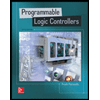