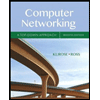
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN: 9780133594140
Author: James Kurose, Keith Ross
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Please help with coding this in Java
In this part, you will implement a class edu.ucdenver.morse.Morse that will provide two instance methods: encode(String) and decode(String).
- Create the class Morse in the package edu.ucdenver.morse
- Add two private hash maps (instance variables) between strings, to map the encoding representation.
- One will map the actual character to the sequence of dots and dashes to represent that character.
- The other will do the reverse mapping.
- Initialize these maps in the constructor.
- Implement the instance methods to encode/decode as follows:
- Implement the encode(String) method, that given a clean string (normat text) will return the Morse Code encoded representation
- Example:
- Input String: "Hello World"
- Output String: "....=.=.-..=.-..=---= .--=---=.-.=.-..=-..="
- Implement the decode(String) method, that given the encoded string (Morse Code) will return the clean text representation.
- Example:
- Input String: "....=.=.-..=.-..=---= .--=---=.-.=.-..=-..="
- Output String: "HELLO WORLD"
- Encoding/Decoding characteristics:
- Use the representation given in the image above.
- After each character add an equal sign (=).
- After each word, add an space. Do not add space after the last word (at the end of the sentence).
- Implement the encode(String) method, that given a clean string (normat text) will return the Morse Code encoded representation
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution
Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 1 images

Knowledge Booster
Similar questions
- import java.util.HashSet; import java.util.Set; // Define a class named LinearSearchSet public class LinearSearchSet { // Define a method named linearSearch that takes in a Set and an integer target // as parameters public static boolean linearSearch(Set<Integer> set, int target) { // Iterate over all elements in the Set for () { // Check if the current value is equal to the target if () { // If so, return true } } // If the target was not found, return false } // Define the main method public static void main(String[] args) { // Create a HashSet of integers and populate integer values Set<Integer> numbers = new HashSet<>(); // Define the target to search for numbers.add(3); numbers.add(6); numbers.add(2); numbers.add(9); numbers.add(11); // Call the linearSearch method with the set…arrow_forwardJava Code: Build the two variable classes that we need: InterpreterDataType and a subclass InterpreterArrayDataType. I will abbreviate these as IDT and IADT. IDT holds a string and has two constructors - one with and one without an initial value supplied. IADT holds a HashMap<String,IDT>. Its constructor should create that hash map. We will use these as our variable storage classes as the interpreter runs. Let's start the interpreter itself. Create a new class (Interpreter). Inside of it, we will have an inner class - LineManager. We will also need a HashMap<String,IDT> that will hold our global variables. We will also have a member HashMap<String, FunctionDefinitionNode> - this will be our source when someone calls a function. The LineManager should take a List<String> as a parameter to the constructor and store it in a member. This will be our read-in input file. It will need a method : SplitAndAssign. This method will get the next line and split it by looking…arrow_forwardWrite a generic class Students.java which has a constructor that takes three parameters – id, name, and type. Type will represent if the student is ‘remote’ or ‘in-person’. A toString() method in this class will display these details for any student. A generic method score() will be part of this class and it will be implemented by inherited classes. Write accessors and mutators for all data points. Write two classes RemoteStudents.java and InPersonStudents.java that inherits from Student class. Show the use of constructor from parent class (mind you, RemoteStudents have one additional parameter – discussion). Implement the abstract method score() of the parent class to calculate the weighted score for both types of students. Write a driver class JavaProgramming.java which has the main method. Create one remote student object and one in-person student object. The output should show prompts to enter individual scores – midterm, finals, ...... etc. and the program will…arrow_forward
- Please do not give solution in image format thanku USING JAVA Modify the code to use Arraylist Instead of HashmapCODE:import java.util.ArrayList;import java.util.HashMap;import java.util.List;import java.util.Map;class Student {private String name;private List<String> certificates;public Student(String name) {this.name = name;this.certificates = new ArrayList<>();}public void addCertificate(String certificate) { certificates.add(certificate);}public boolean hasCertificate(String certificate) {return certificates.contains(certificate);}public String getName() {return name;}}public class CertificateManagement {public static void main(String[] args) { Map<String, Student> students = new HashMap<>();Student student1 = new Student("John");student1.addCertificate("Java");student1.addCertificate("Python");students.put("John", student1);Student student2 = new Student("Alice");student2.addCertificate("C++");students.put("Alice", student2);Student student3 = new…arrow_forwardGiven that an ArrayList of Strings named friendList has already been created and names have already been inserted, write the statement necessary to change the name "Tyler", stored at index 8, to "Bud". This is needed in Javaarrow_forwardWrite a method called inversePrint. This method gets a head of a linkedlist and Recursively print from the last item to the first one. This is a Recursive method. If Recursive is not used you will get no mark for this question Do not reverse the linkedlist, only print it in reverse example call: in the main I will call your method like: buffer = myList.head; //This is a buffer to copy the head address inversePrint(buffer); After this, the order for myList should not changed. if myList has: 1->2->3->4->5 your function should print: 5-> 4 -> 3->2->1 but if I check myList the order should still be 1->2->3->4->5 you can assume the LinkedList code for all other operations exist and if you need you can use them Do Not create a new linkedlist in inverse Hint: use recursion does this for you really easyarrow_forward
- In Java, Create Movie class, which has private fields for title, director, rating. It has a constructor to initialize these fields and a toString method for printing movie details. There is also a getRating method to retrieve the movie rating. Create Rating Comparator class which implements the Comparator interface for comparing movies based on their ratings. Create DemoMovies Class. Initialize necessary data structures like LinkedList, TreeMap for rating as key and Movie from Movie class as value, TreeSet, and ProtiyQueue. Use a loop to take user input for movie detailsuntil the user enters 'WWW' as the movie title.Inside the loop, for each movie, create a Movie object, add it to various data structures that is title, director and rating based on the constructor. Add the Movie object to the LinkedList. Add the rating and the object to the TreeMap.Add the rating to the TreeSet.Add the Movie object to the Priority Queue.Display the entered movies. Sort and display Movies by rate by…arrow_forwardPlease Code in JAVA. Type the code out neatly. Add comments . (No need for long comments). Dont add any unnecesary code or comments. Do what the attached file says. And call it a day. Thanks !arrow_forwardCan someone help me with this code, I not really understanding how to do this? import java.util.ArrayList;import java.util.HashMap;import java.util.Map;/*** @version Spring 2019* @author Kyle*/public class MapProblems {/*** Modify and return the given map as follows: if the key "a" has a value, set the key "b" to* have that value, and set the key "a" to have the value "". Basically "b" is confiscating the* value and replacing it with the empty string.** @param map to be edited* @return map*/public Map<String, String> confiscate(Map<String, String> map) {if (map.containsKey("a") && map.containsValue("a")) {}}/*** Modify and return the given map as follows: if the key "duck" has a value, set the key* "goose" to have that same value. In all cases remove the key "swan", the rest of the map* should not change.** @param map to be edited* @return map*/public Map<String, String> mapBird1(Map<String, String> map) {throw new UnsupportedOperationException("Not…arrow_forward
arrow_back_ios
arrow_forward_ios
Recommended textbooks for you
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
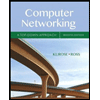
Computer Networking: A Top-Down Approach (7th Edi...
Computer Engineering
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:PEARSON
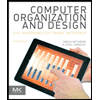
Computer Organization and Design MIPS Edition, Fi...
Computer Engineering
ISBN:9780124077263
Author:David A. Patterson, John L. Hennessy
Publisher:Elsevier Science
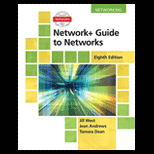
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:9781337569330
Author:Jill West, Tamara Dean, Jean Andrews
Publisher:Cengage Learning
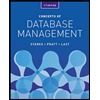
Concepts of Database Management
Computer Engineering
ISBN:9781337093422
Author:Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:Cengage Learning
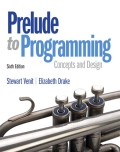
Prelude to Programming
Computer Engineering
ISBN:9780133750423
Author:VENIT, Stewart
Publisher:Pearson Education
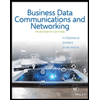
Sc Business Data Communications and Networking, T...
Computer Engineering
ISBN:9781119368830
Author:FITZGERALD
Publisher:WILEY