Please use the information on the first screenshot about a class BST and node class to implement a method named “randomArray” in the file named “BSTTest.java” that tests a specific sorting algorithm. This method will take one parameter: size of a randomly generated array (int): e.g., 10 This method will: Generate and return a random array of the length specified by the parameter The value range of each element should be between 0 and 1000 (inclusive) >>> randomArray(5). >>> [1, 2, 3, 7, 10] Implement a method named “test” in the file named “BSTTest.java”. This method should take advantage of the implemented randomArray and print out information as such: Testing of BST starts. Insert 3 Insert 2 Insert 5 Insert 100 Delete 3. The traverse gives a BST as 3, 5, 100 Testing of BST ends. Please note that the goal is not to manually code the printings of each line. Try your best to make this method as automatic as possible. There are no limits on what parameters this method shall accept or what it returns. However, please make sure to use the method to perform testing for at least two cases in your main method of BSTTest.java. Please use the following code as a base. import java.util.*; public class BSTTest{ public static void main(String[] args) { // perform at least one test on each operation of your BST } private int[] randomArray(int size) { // remove the two lines int[] arr = new int[1]; return arr; } // the parameters and return are up to you to define; this method needs to be uncommented // private test() { // // } }
Please use the information on the first screenshot about a class BST and node class to implement a method named “randomArray” in the file named “BSTTest.java” that tests a specific sorting
- size of a randomly generated array (int): e.g., 10
This method will:
- Generate and return a random array of the length specified by the parameter
- The value range of each element should be between 0 and 1000 (inclusive)
>>> randomArray(5).
>>> [1, 2, 3, 7, 10]
Implement a method named “test” in the file named “BSTTest.java”. This method should take advantage of the implemented randomArray and print out information as such:
Testing of BST starts.
Insert 3
Insert 2
Insert 5
Insert 100
Delete 3.
The traverse gives a BST as 3, 5, 100
Testing of BST ends.
Please note that the goal is not to manually code the printings of each line. Try your best to make this method as automatic as possible. There are no limits on what parameters this method shall accept or what it returns. However, please make sure to use the method to perform testing for at least two cases in your main method of BSTTest.java.
Please use the following code as a base.
import java.util.*;
public class BSTTest{
public static void main(String[] args) {
// perform at least one test on each operation of your BST
}
private int[] randomArray(int size) {
// remove the two lines
int[] arr = new int[1];
return arr;
}
// the parameters and return are up to you to define; this method needs to be uncommented
// private test() {
//
// }
}
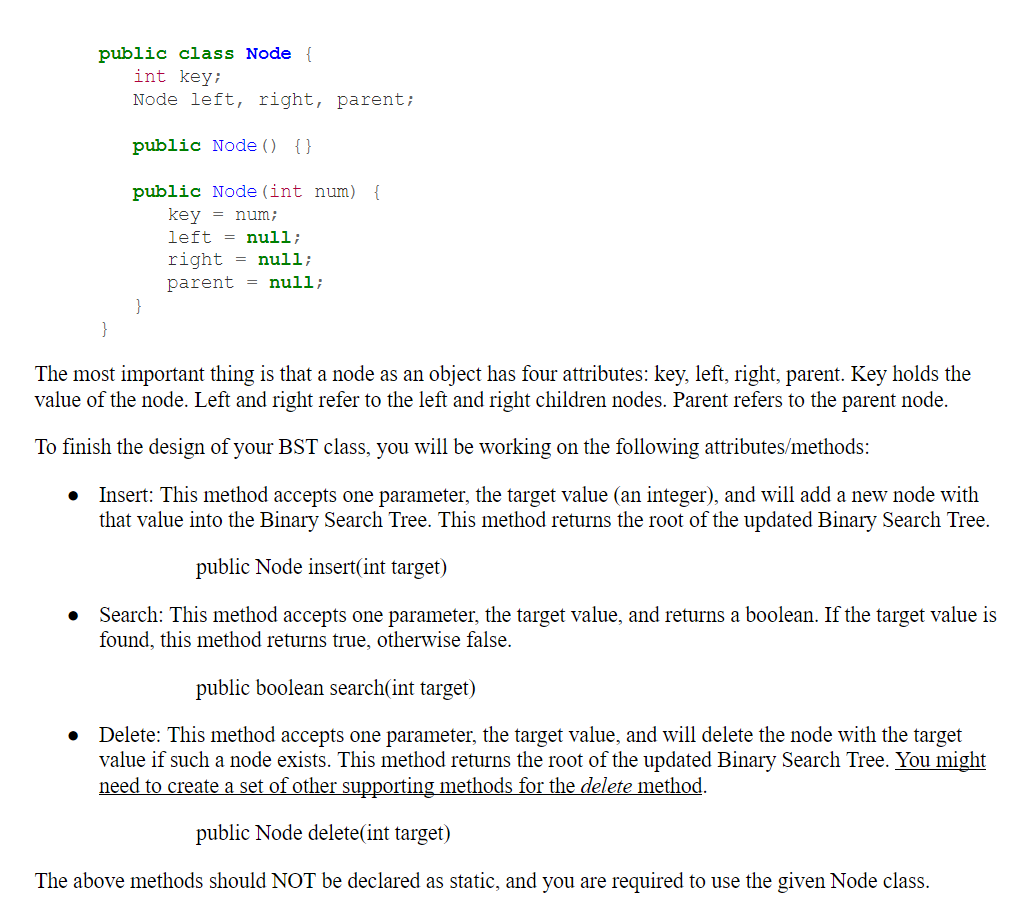

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

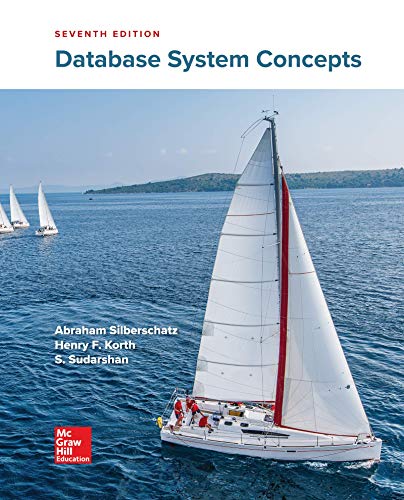
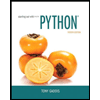
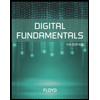
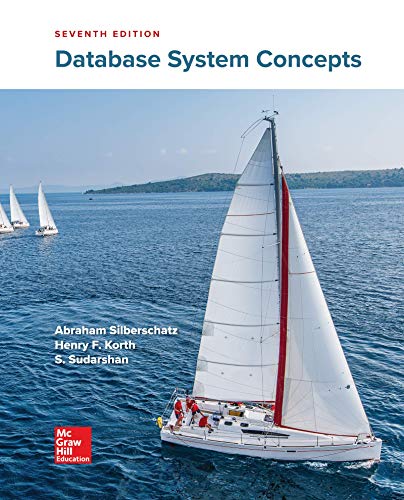
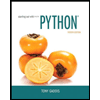
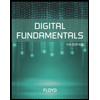
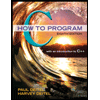
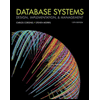
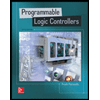