PYTHON/ COMPUTATIONAL STOICHIOMETRY Please help me build the stochiometric matrix for the chemical reaction.kindly use the code provided to guide your solution. 1.7 A + 2.1 B + 1.5 C <=> 3 D + 3.8 E + 1.2 F Code Block to build stoichiometric matrix: import numpy as np # Initialize the stoichiometric matrix as zeros_mtrx = np.zeros((len(reactions), len(species))) for (i_row, r) in enumerate(reactions): left = r.split('<=>')[0].strip() right = r.split('<=>')[1].strip() left_terms = left.split('+') left_terms = [t.strip() for t in left_terms] # in-place clean up right_terms = right.split('+') right_terms = [t.strip() for t in right_terms] # in-place clean up for t in left_terms: # reactants tmp = t.split(' ') # split stoichiometric coeff and species name if len(tmp) == 2: # stoich coeff and species name coeff = float(tmp[0].strip()) species_member = tmp[1].strip() j_col = species.index(species_member) # find id of species in the species list assert s_mtrx[i_row,j_col] == 0.0, \ 'duplicates not allowed r%r: %r %r r'%\ (i_row,r,species_member,s_mtrx[i_row,j_col]) s_mtrx[i_row,j_col] = -1.0 * coeff else: # only species name species_member = tmp[0].strip() j_col = species.index(species_member) assert s_mtrx[i_row,j_col] == 0.0, \ 'duplicates not allowed r%r: %r %r r'%\ (i_row,r,species_member,s_mtrx[i_row,j_col]) s_mtrx[i_row,j_col] = -1.0 for t in right_terms: # products tmp = t.split(' ') if len(tmp) == 2: coeff = float(tmp[0].strip()) species_member = tmp[1].strip() j_col = species.index(species_member) assert s_mtrx[i_row,j_col] == 0.0, \ 'duplicates not allowed r%r: %r %r r'%\ (i_row,r,species_member,s_mtrx[i_row,j_col]) s_mtrx[i_row,j_col] = 1.0 * coeff else: species_member = tmp[0].strip() j_col = species.index(species_member) assert s_mtrx[i_row,j_col] == 0.0, \ 'duplicates not allowed r%r: %r %r r'%\ (i_row,r,species_member,s_mtrx[i_row,j_col]) s_mtrx[i_row,j_col] = 1.0 print('m x n =',s_mtrx.shape)print('s_mtrx =\n',s_mtrx)print('')print('mole balance vector =\n', s_mtrx@np.ones(len(species)))print('mole balance vector =\n', s_mtrx.sum(1))
PYTHON/ COMPUTATIONAL STOICHIOMETRY
Please help me build the stochiometric matrix for the chemical reaction.kindly use the code provided to guide your solution.
1.7 A + 2.1 B + 1.5 C <=> 3 D + 3.8 E + 1.2 F
Code Block to build stoichiometric matrix:
import numpy as np
# Initialize the stoichiometric matrix as zero
s_mtrx = np.zeros((len(reactions), len(species)))
for (i_row, r) in enumerate(reactions):
left = r.split('<=>')[0].strip()
right = r.split('<=>')[1].strip()
left_terms = left.split('+')
left_terms = [t.strip() for t in left_terms] # in-place clean up
right_terms = right.split('+')
right_terms = [t.strip() for t in right_terms] # in-place clean up
for t in left_terms: # reactants
tmp = t.split(' ') # split stoichiometric coeff and species name
if len(tmp) == 2: # stoich coeff and species name
coeff = float(tmp[0].strip())
species_member = tmp[1].strip()
j_col = species.index(species_member) # find id of species in the species list
assert s_mtrx[i_row,j_col] == 0.0, \
'duplicates not allowed r%r: %r %r r'%\
(i_row,r,species_member,s_mtrx[i_row,j_col])
s_mtrx[i_row,j_col] = -1.0 * coeff
else: # only species name
species_member = tmp[0].strip()
j_col = species.index(species_member)
assert s_mtrx[i_row,j_col] == 0.0, \
'duplicates not allowed r%r: %r %r r'%\
(i_row,r,species_member,s_mtrx[i_row,j_col])
s_mtrx[i_row,j_col] = -1.0
for t in right_terms: # products
tmp = t.split(' ')
if len(tmp) == 2:
coeff = float(tmp[0].strip())
species_member = tmp[1].strip()
j_col = species.index(species_member)
assert s_mtrx[i_row,j_col] == 0.0, \
'duplicates not allowed r%r: %r %r r'%\
(i_row,r,species_member,s_mtrx[i_row,j_col])
s_mtrx[i_row,j_col] = 1.0 * coeff
else:
species_member = tmp[0].strip()
j_col = species.index(species_member)
assert s_mtrx[i_row,j_col] == 0.0, \
'duplicates not allowed r%r: %r %r r'%\
(i_row,r,species_member,s_mtrx[i_row,j_col])
s_mtrx[i_row,j_col] = 1.0
print('m x n =',s_mtrx.shape)
print('s_mtrx =\n',s_mtrx)
print('')
print('mole balance
print('mole balance vector =\n', s_mtrx.sum(1))

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

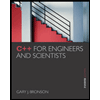
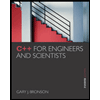