python language Identify what is wrong with the following code. Find at least 6 issues. There are a total of 10 issues (anything over 6 is bonus). # Node Class class Node1 def __init__(self, cargo, next=None): self.cargo = self self.next = next def __str__(self): return str(self.cargo) # Priority Queue Class class PriorityQueue(): def __init__(): self.items = [] def is_empty(self): return not self.items def insert(self, item): self.items.add(item) def remove(self): maxi = 0 for i in range(1, len(self.items)): if self.items[i] > self.items[maxi] then: maxi = i item = self.items[maxi] delete self.items[maxi] return item # Golfer Class classes Golfer: def __init__(self, name, score): self.name = name self.score = _score def __str__(self): return "{0}: {1}".format(self.name, self.score) def gt__(self, other): return self.score < other.score // Main Program pq = PriorityQueue(Golfer) tiger = new Golfer("Tiger Woods", 61) phil = Golfer("Phil Mickelson", 72) hal = Golfer("Hal Sutton", 69) seth = Golfer("Seth Volpe", 95) jeff = Golfer("Jeff Volpe", 87) mike = Golfer(75, "Mike Voltz") for g in [tiger, phil, hal, seth, jeff, mike]: PriorityQueue.insert(g) while not pq.is_empty(): print(pq.remove())
python language
Identify what is wrong with the following code. Find at least 6 issues. There are a total of 10 issues (anything over 6 is bonus).
- # Node Class
-
class Node1
-
def __init__(self, cargo, next=None):
-
self.cargo = self
-
self.next = next
-
def __str__(self):
- return str(self.cargo)
-
-
# Priority Queue Class
-
class PriorityQueue():
- def __init__():
- self.items = []
- def is_empty(self):
-
return not self.items
-
def insert(self, item):
-
self.items.add(item)
-
def remove(self):
-
maxi = 0
-
for i in range(1, len(self.items)):
-
if self.items[i] > self.items[maxi] then:
-
maxi = i
-
item = self.items[maxi]
-
delete self.items[maxi]
-
return item
-
-
# Golfer Class
-
classes Golfer:
-
def __init__(self, name, score):
-
self.name = name
-
self.score = _score
-
def __str__(self):
-
return "{0}: {1}".format(self.name, self.score)
-
def gt__(self, other):
-
return self.score < other.score
-
-
// Main Program
-
pq = PriorityQueue(Golfer)
-
tiger = new Golfer("Tiger Woods", 61)
-
phil = Golfer("Phil Mickelson", 72)
-
hal = Golfer("Hal Sutton", 69)
-
seth = Golfer("Seth Volpe", 95)
-
jeff = Golfer("Jeff Volpe", 87)
-
mike = Golfer(75, "Mike Voltz")
-
for g in [tiger, phil, hal, seth, jeff, mike]:
-
PriorityQueue.insert(g)
-
while not pq.is_empty():
-
print(pq.remove())

Step by step
Solved in 2 steps

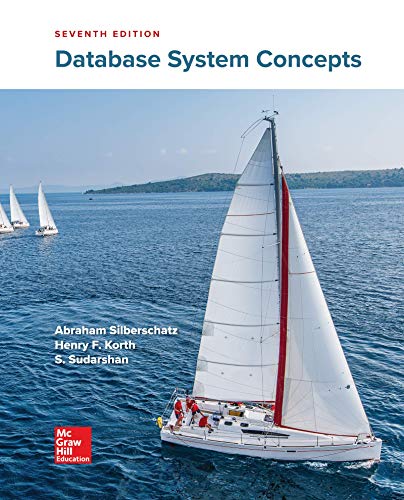
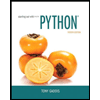
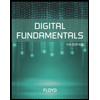
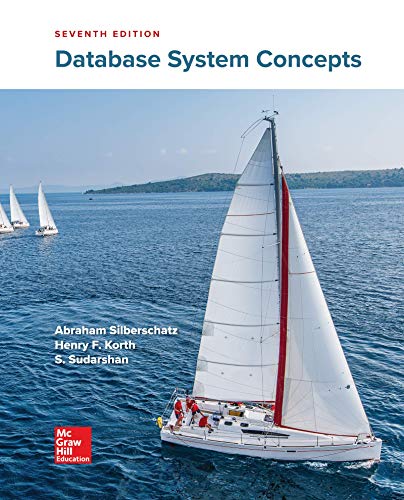
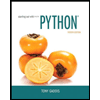
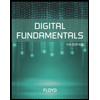
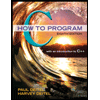
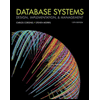
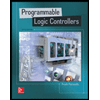