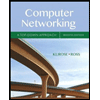
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN: 9780133594140
Author: James Kurose, Keith Ross
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Working with Queues
1. Create a method on the Queue class that calculates the number of times a given value occurs in the queue.
int Queue::count( const T & data ) const;
Example: count(…) function Result Queue count (5) 3 front->(1) (1) (5) (3) (5) (5) (2)<-back count (10) 0 front->(1) (1) (5) (3) (5) (5) (5) (2)<-back
2. Create a method on the Queue class that determines whether the queue is in descending order.
bool Queue::isDecreasing( ) const;
Example: isDecreasing() function Result Queue count (5) true front->(10) (9) (8) (7) (1)<-back count (10) false front->(1) (1) (5) (3) (5) (5) (5) (2)<-back 2
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution
Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 1 images

Knowledge Booster
Similar questions
- class Queue { private static int front, rear, capacity; private static int queue[]; Queue(int c) { front = rear = 0; capacity = c; queue = new int[capacity]; } static void queueEnqueue(int data) { if (capacity == rear) { System.out.printf("\nQueue is full\n"); return; } else { queue[rear] = data; rear++; } return; } static void queueDequeue() { if (front == rear) { System.out.printf("\nQueue is empty\n"); return; } else { for (int i = 0; i < rear - 1; i++) { queue[i] = queue[i + 1]; } if (rear < capacity) queue[rear] = 0; rear--; } return; } static void queueDisplay() { int i; if (front == rear) { System.out.printf("\nQueue is Empty\n"); return; } for (i = front; i < rear; i++) { System.out.printf(" %d <-- ", queue[i]); } return; } static void queueFront() { if (front == rear) { System.out.printf("\nQueue is Empty\n"); return; } System.out.printf("\nFront Element is: %d", queue[front]);…arrow_forwardThe queue operation that is required when using an array implementation, but is not required when using a linked list implementation, is ___. isFull() isEmpty() peek() dequeue()arrow_forwardChange the __str__ method of the Queue class (provided below) so that it prints each object in the queue along with its order in the queue (see sample output below). class Queue(): def __init__(self): self.queue = [] # implement with Python lists! # start of physical Python list == front of a queue # end of physical Python list == back of a queue def enqueue(self, new_obj): self.queue.append(new_obj); def dequeue(self): return self.queue.pop(0) def peek(self): return self.queue[0] def bad_luck(self): return self.queue[-1] def __str__(self): return str(self.queue) # let's try a more fun waySample output: >>> my_queue = Queue()>>> everyone = ["ESC", "ABC", "YOLO", "HTC"]>>> for initials in everyone:>>> my_queue.enqueue(initials)>>> print(my_queue)Output: 1: ESC2: ABC3: YOLO4: HTCarrow_forward
- Problem 7: Assume that a queue class called XQueue has a no argument constructor, an enqueue() method, a dequeue() method and an isEmpty() method. Write a method that merges two given queues by copying the elements in an alternating sequence and returning the resulting combined queue. Note: make sure that you don't include unnecessary spaces in your answer! public XQueue merge(XQueue q1, XQueue q2) { XQueue neWQueue = new XQueue(); while (!q1.isEmpty() && !q2.isEmpty()) { newQueue.enqueue( #01 ); newQueue.enqueue( #02); } while (!q1.isEmpty()) { #03 ; } while (!q2.is Empty()) { #04 ; } return newQueue (); }arrow_forwardAsap do this? .arrow_forwardDONT use any of the list methods (append, pop etc.). class mycircularqueue(): def __init__(self, m = 10): self.maxsize = m self.array = [None]*m self.FRONT = self.REAR = -1 def enqueue(self, item): # Inserts item at the rear of a non full queue and returns True. If unable to insert the item returns false ####################################################################### # Remove the pass statement and write your code ####################################################################### pass def dequeue(self): # Removes the item from the front of the queue and returns it. For unsuccessful dequeue return False ####################################################################### # Remove the pass statement and write your code ####################################################################### pass def peek(self): # Returns the…arrow_forward
- java Suppose queue is defined as LinkedQueue<Integer> queue = new LinkedQueue<>(); Show what is written by the following segment of code. SHOW YOUR WORK.arrow_forwardimport java.util.*;import java.io.*; public class HuffmanCode { private Queue<HuffmanNode> queue; private HuffmanNode overallRoot; public HuffmanCode(int[] frequencies) { queue = new PriorityQueue<HuffmanNode>(); for (int i = 0; i < frequencies.length; i++) { if (frequencies[i] > 0) { HuffmanNode node = new HuffmanNode(frequencies[i]); node.ascii = i; queue.add(node); } } overallRoot = buildTree(); } public HuffmanCode(Scanner input) { overallRoot = new HuffmanNode(-1); while (input.hasNext()) { int asciiValue = Integer.parseInt(input.nextLine()); String code = input.nextLine(); overallRoot =…arrow_forward
arrow_back_ios
arrow_forward_ios
Recommended textbooks for you
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
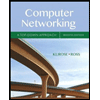
Computer Networking: A Top-Down Approach (7th Edi...
Computer Engineering
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:PEARSON
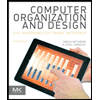
Computer Organization and Design MIPS Edition, Fi...
Computer Engineering
ISBN:9780124077263
Author:David A. Patterson, John L. Hennessy
Publisher:Elsevier Science
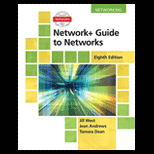
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:9781337569330
Author:Jill West, Tamara Dean, Jean Andrews
Publisher:Cengage Learning
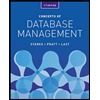
Concepts of Database Management
Computer Engineering
ISBN:9781337093422
Author:Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:Cengage Learning
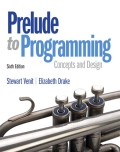
Prelude to Programming
Computer Engineering
ISBN:9780133750423
Author:VENIT, Stewart
Publisher:Pearson Education
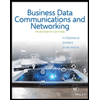
Sc Business Data Communications and Networking, T...
Computer Engineering
ISBN:9781119368830
Author:FITZGERALD
Publisher:WILEY