Question 1. Write a Pizza class so that this client code works.
Please use Python for this question:
Question 1. Write a Pizza class so that this client code works.
The Pizza class should have two attributes(data items):
size - a single character str, one of 'S','M',L"
toppings - a set containing the toppings.
The Pizza class should have the following methods/operators):
__init__ - constructs a Pizza of a given size (defaults to 'M') and with a given set of toppings (defaults to empty set). I highly recommend you look at the Queue class in the book to see how to get this to work correctly.
setSize - set pizza size to one of 'S','M'or 'L'
getSize - returns size
addTopping - adds a topping to the pizza, no duplicates, i.e., adding 'pepperoni' twice only adds it once
removeTopping - removes a topping from the pizza
price - returns the price of the pizza according to the following scheme:
'S': $6.25 plus 70 cents per topping
'M': $9.95 plus $1.45 per topping
'L': $12.95 plus $1.85 per topping
__repr__ - returns representation as a string - see output sample above. Note that toppings may be listed in a different order.
__eq__ - two pizzas are equal if they have the same size and same toppings (toppings don't need to be in the same order)
Output for Question 1:
M
mushroom
onion
garlic
L
calamari
garlic
S
# hw8TEST.py
above lines are user inputs to be used for code
that requires user input
>>> from hw8 import *
##### Pizza #####
>>> pie = Pizza()
>>> pie
Pizza('M',set())
>>> pie.setSize('L')
>>> pie.getSize()
'L'
>>> pie.addTopping('pepperoni')
>>> pie
Pizza('L',{'pepperoni'})
>>> pie.addTopping('anchovies')
>>> pie.addTopping('mushrooms')
>>> pie==Pizza('L',{'anchovies', 'mushrooms', 'pepperoni'})
True
>>> pie.addTopping('pepperoni')
>>> pie==Pizza('L',{'anchovies', 'mushrooms', 'pepperoni'})
True
>>> pie.removeTopping('anchovies')
>>> pie==Pizza('L',{'mushrooms', 'pepperoni'})
True
>>> pie.price()
16.65
Note: In the following code,
p==p2 shoudl be False. If True
than they are sharing the same set (or list) of toppings
You need to make sure that the set() constructor is
called in all cases in the body of the Pizza
constructor. See course notes for the Queue class.
>>> p = Pizza()
>>> p2 = Pizza()
>>> p.addTopping('mushroom')
>>> p2.addTopping('onion')
>>> p.addTopping('mushroom')
>>> p
Pizza('M',{'mushroom'})
>>> p2
Pizza('M',{'onion'})
>>> p==p2 # see note in TEST file
False
>>>
# reroute stdin #
code below directs input to be received from above
should not cause an error
>>> import sys
>>> si = sys.stdin
>>> sys.stdin = open('hw8TEST.py')
Question 2: Write a function orderPizza that allows the user input to build a pizza. It then prints a thank you message, the cost of the pizza and then returns the Pizza that was built.
Output for Question 2:
##### orderPizza #####
>>> orderPizza()==Pizza('M',{'garlic', 'onion', 'mushroom'})
Welcome to Python Pizza!
What size pizza would you like (S,M,L): Type topping to add (or Enter to quit): Type topping to add (or Enter to quit): Type topping to add (or Enter to quit): Type topping to add (or Enter to quit): Thanks for ordering!
Your pizza costs $14.299999999999999
True
>>> orderPizza()==Pizza('L',{'calamari', 'garlic'})
Welcome to Python Pizza!
What size pizza would you like (S,M,L): Type topping to add (or Enter to quit): Type topping to add (or Enter to quit): Type topping to add (or Enter to quit): Thanks for ordering!
Your pizza costs $16.65
True
>>> orderPizza()==Pizza('S',set())
Welcome to Python Pizza!
What size pizza would you like (S,M,L): Type topping to add (or Enter to quit): Thanks for ordering!
Your pizza costs $6.25
True
#stdin back #
put stdin back to original, again, shouldnt cause error
>>> sys.stdin = si # return stdin

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

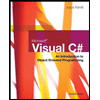
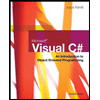