Review the program Warning java that reads in a file of student academic credit data. Each line of the input file will contain the student name (a single String with no spaces), the number of semester hours earned (an integer), the total quality points earned (a double). Here is the students.dat data file: students.dat Smith 27 83.7 Jones 21 28.35 Malker 96 182.4 Doe 60 150 Wood 100 400 Street 33 S7.4 Taylor 83 190 Davis 110 198 Smart 75 292.5 Bird 84 168 Summers 52 83.2 The program should compute the GPA (grade point or quality point average) for cach student (the total quality points divided by the number of semester hours), and display the student name if the gpa is less than 2.0. The file Warning java contains a skeleton of the program. Do the following: 1. Add the code to caleculate the gpa. 2. Display the name if the gpa is less than 2.0. 3. Test to ensure the output is accurate. 4. Add code to catch the following exceptions: • A FileNotFoundException if the input file does not exist. How will you test for this exception? A NumberFormatException if it can't parse an int or double when it tries to – this indicates an ere in the input file format. How will you test for this error? Display the record number in error. error
Review the program Warning java that reads in a file of student academic credit data. Each line of the input file will contain the student name (a single String with no spaces), the number of semester hours earned (an integer), the total quality points earned (a double). Here is the students.dat data file: students.dat Smith 27 83.7 Jones 21 28.35 Malker 96 182.4 Doe 60 150 Wood 100 400 Street 33 S7.4 Taylor 83 190 Davis 110 198 Smart 75 292.5 Bird 84 168 Summers 52 83.2 The program should compute the GPA (grade point or quality point average) for cach student (the total quality points divided by the number of semester hours), and display the student name if the gpa is less than 2.0. The file Warning java contains a skeleton of the program. Do the following: 1. Add the code to caleculate the gpa. 2. Display the name if the gpa is less than 2.0. 3. Test to ensure the output is accurate. 4. Add code to catch the following exceptions: • A FileNotFoundException if the input file does not exist. How will you test for this exception? A NumberFormatException if it can't parse an int or double when it tries to – this indicates an ere in the input file format. How will you test for this error? Display the record number in error. error
A+ Guide To It Technical Support
10th Edition
ISBN:9780357108291
Author:ANDREWS, Jean.
Publisher:ANDREWS, Jean.
Chapter18: Macos, Linux, And Scripting
Section: Chapter Questions
Problem 14TC
Related questions
Question
100%
![Q Search the web...
f a
New Tab
1 https://ccbcmd-bb.blackboard x
b My Questions | bartleby
O CS220 / CS250 Laboratory #1
O https://ccbcmd-bb.blackboarc x
C Write A Program That Will Rea x
A https://ccbcmd-bb.blackboard.com/bbcswebdav/pid-10404035-dt-content-rid-47331005_1/courses/CSIT210.9. *
Sign in
...
//
//
Warning.java
Java Foundations
//
//
Reads student data from a text file and writes data to another text file.
/ **率率*於於 ** 事
import java.util.Scanner;
import java.io.*;
public class Warning
{
public static void main (String[] args)throws IOException
int creditHrs;
double qualityPts;
// number of semester hours earned
// number of quality points earned
// grade point (quality point) average
double gpa;
String name;
// Set up scanner to input file
Scanner infile = new Scanner (new File("g:\\students.dat"));
System.out.println ("\n
Students on Academic Warning\n");
// Process the input file, one token at a time
while (inFile.hasNext())
{
// Get the credit hours and quality points and
// determine if the student is on warning. If so,
// display the student's name.
name = inFile.next();
creditHrs = Integer.parseInt(inFile.next());
qualityPts = Double.parseDouble(inFile.next());
// Insert gpa calculation
//
and statement to determine if the student name is listed.
}
inFile.close();
//insert catch statements
}
2:19 PM
P Type here to search
Bb
Address
11/14/2020
近](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fff17d012-70ad-4782-80ac-5077bdfc24e1%2Fc07358e9-9910-4904-9609-2f07a70ed70d%2Ffrh52p6_processed.png&w=3840&q=75)
Transcribed Image Text:Q Search the web...
f a
New Tab
1 https://ccbcmd-bb.blackboard x
b My Questions | bartleby
O CS220 / CS250 Laboratory #1
O https://ccbcmd-bb.blackboarc x
C Write A Program That Will Rea x
A https://ccbcmd-bb.blackboard.com/bbcswebdav/pid-10404035-dt-content-rid-47331005_1/courses/CSIT210.9. *
Sign in
...
//
//
Warning.java
Java Foundations
//
//
Reads student data from a text file and writes data to another text file.
/ **率率*於於 ** 事
import java.util.Scanner;
import java.io.*;
public class Warning
{
public static void main (String[] args)throws IOException
int creditHrs;
double qualityPts;
// number of semester hours earned
// number of quality points earned
// grade point (quality point) average
double gpa;
String name;
// Set up scanner to input file
Scanner infile = new Scanner (new File("g:\\students.dat"));
System.out.println ("\n
Students on Academic Warning\n");
// Process the input file, one token at a time
while (inFile.hasNext())
{
// Get the credit hours and quality points and
// determine if the student is on warning. If so,
// display the student's name.
name = inFile.next();
creditHrs = Integer.parseInt(inFile.next());
qualityPts = Double.parseDouble(inFile.next());
// Insert gpa calculation
//
and statement to determine if the student name is listed.
}
inFile.close();
//insert catch statements
}
2:19 PM
P Type here to search
Bb
Address
11/14/2020
近
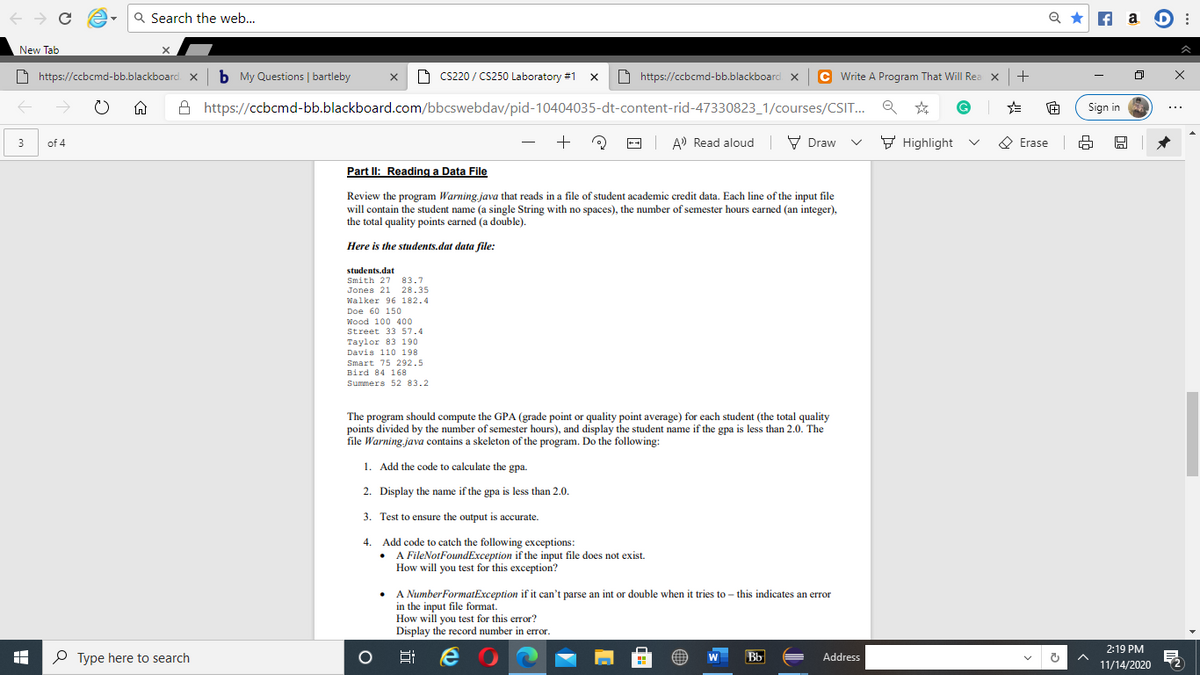
Transcribed Image Text:Q Search the web..
Q *
f a
D
New Tab
https://ccbcmd-bb.blackboard x b My Questions | bartleby
D
CS220 / CS250 Laboratory #1
O https://ccbcmd-bb.blackboard x
C Write A Program That Will Rea x+
A https://ccbcmd-bb.blackboard.com/bbcswebdav/pid-10404035-dt-content-rid-47330823_1/courses/CSIT...
Sign in
...
--
A) Read aloud V Draw
F Highlight
O Erase
3
of 4
-
Part II: Reading a Data File
Review the program Warning.java that reads in a file of student academic credit data. Each line of the input file
will contain the student name (a single String with no spaces), the number of semester hours earned (an integer),
the total quality points earned (a double).
Here is the students.dat data file:
students.dat
Smith 27 83.7
Jones 21 28.35
Walker 96 182.4
Doe 60 150
Wood 100 400
Street 33 57.4
Taylor 83 190
Davis 110 198
Smart 75 292.5
Bird 84 168
Summers 52 83.2
The program should compute the GPA (grade point or quality point average) for each student (the total quality
points divided by the number of semester hours), and display the student name if the gpa is less than 2.0. The
file Warning.java contains a skeleton of the program. Do the following:
1. Add the code to calculate the gpa.
2. Display the name if the gpa is less than 2.0.
3. Test to ensure the output is accurate.
Add code to catch the following exceptions:
• A FileNotFoundException if the input file does not exist.
How will you test for this exception?
4.
A NumberFormatException if it can't parse an int or double when it tries to – this indicates an error
in the input file format.
How will you test for this error?
Display the record number in error.
2:19 PM
P Type here to search
Bb
Address
W
11/14/2020
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 4 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
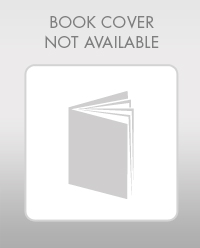
A+ Guide To It Technical Support
Computer Science
ISBN:
9780357108291
Author:
ANDREWS, Jean.
Publisher:
Cengage,
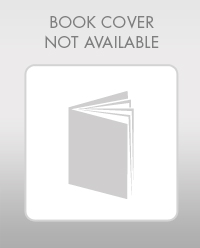
A+ Guide To It Technical Support
Computer Science
ISBN:
9780357108291
Author:
ANDREWS, Jean.
Publisher:
Cengage,