Step 1: Requirements You are developing a software package that requires users to enter their own passwords. Loop until the passwords meet the software criteria: · The password should be at least 6 characters long · The password should contain at least one uppercase and one lowercase letter · The password should have at least one digit The program should have an isValid function that will test if the password is valid. Use the following code to declare a password variable with a global constant int SIZE=80; char password[SIZE]; Next use a while(true) to continue looping until the user enters a valid password. Read in the password and call the isValid program. You can use the isupper(), islower(), and isdigit() functions. For example: if (isdigit(*password)) //if this is true, you know the password has at least one digit. Output from Program: Password requirements: - The password should be at least 6 characters long - The password should contain at least one uppercase - and one lowercase letter. - The password should have at least one digit. Enter a password: aaaaaaa The password was invalid Password requirements: - The password should be at least 6 characters long - The password should contain at least one uppercase - and one lowercase letter. - The password should have at least one digit. Enter a password: aBcdEfg The password was invalid Password requirements: - The password should be at least 6 characters long - The password should contain at least one uppercase - and one lowercase letter. - The password should have at least one digit. Enter a password: abc123D The password is valid Step 2: Processing Logic Using the pseudocode below, write the code that will meet the requirements. Declare constants SIZE and MIN Function prototype for isValid Main Function Declare the password as a character array. While true Display the password requirements Get password from user Call the isValid function Display results of isValid function isValid function Declare Boolean variables Use strlen command to determine the length of the password and if it is greater than MIN, set bool value to true Loop Test if password has a lowercase letter Test if password has an upperecase letter Test if password has a digit Go to next character (ie *password++;) End loop If all boolean values are true, return 1, else return 0
Step 1: Requirements |
You are developing a software package that requires users to enter their own passwords. Loop until the passwords meet the software criteria:
· The password should be at least 6 characters long · The password should contain at least one uppercase and one lowercase letter · The password should have at least one digit
The program should have an isValid function that will test if the password is valid. Use the following code to declare a password variable with a global constant int SIZE=80; char password[SIZE];
Next use a while(true) to continue looping until the user enters a valid password. Read in the password and call the isValid program. You can use the isupper(), islower(), and isdigit() functions. For example: if (isdigit(*password)) //if this is true, you know the password has at least one digit.
Output from Program:
Password requirements: - The password should be at least 6 characters long - The password should contain at least one uppercase - and one lowercase letter. - The password should have at least one digit.
Enter a password: aaaaaaa The password was invalid
Password requirements: - The password should be at least 6 characters long - The password should contain at least one uppercase - and one lowercase letter. - The password should have at least one digit.
Enter a password: aBcdEfg The password was invalid
Password requirements: - The password should be at least 6 characters long - The password should contain at least one uppercase - and one lowercase letter. - The password should have at least one digit.
Enter a password: abc123D The password is valid
|
Step 2: Processing Logic |
Using the pseudocode below, write the code that will meet the requirements.
Declare constants SIZE and MIN Function prototype for isValid Main Function
Declare the password as a character array. While true Display the password requirements Get password from user Call the isValid function Display results of isValid function
isValid function Declare Boolean variables Use strlen command to determine the length of the password and if it is greater than MIN, set bool value to true Loop Test if password has a lowercase letter Test if password has an upperecase letter Test if password has a digit Go to next character (ie *password++;) End loop If all boolean values are true, return 1, else return 0
|

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

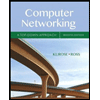
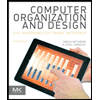
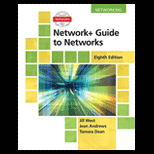
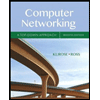
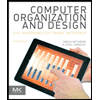
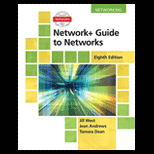
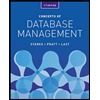
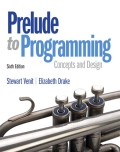
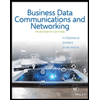