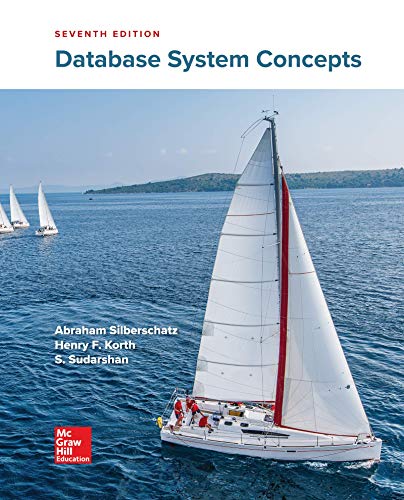
Include flowchart for this code
//C++ Code
#include<iostream>
#include<fstream>
using namespace std;
/*Create a global variable of type ofstream for the output file*/
ofstream outfile;
/*
* This function asks the user for the number of employees in the company.
This value should be returned as an int.
The function accepts no arguments (No parameter/input).
*/
int NumOfEmployees();
/*
* accepts an argument of type int for the number of employees
in the company and returns the total of missed days as an int.
This function should do the following:
Asks the user to enter the following information
for each employee:
The employee number (ID) (Assume the employee number
is 4 digits or fewer, but don't validate it).
The number of days that employee missed
during the past year.
Writes each employee number (ID)
and the number of days missed to the output file
*/
int TotDaysAbsent(int numberOfEmployees);
/*
calculates the average number of days absent.
The function takes two arguments:
the number of employees in the company
The total number of days absent for all employees during the year.
This function should return, as a double, the average number of days absent.
*/
double AverageAbsent(int numberOfEmp, int totalAbsent);
//Function Definitions
double AverageAbsent(int numberOfEmp, int totalAbsent)
{
return (double)totalAbsent / numberOfEmp;
}
int TotDaysAbsent(int numberOfEmployees)
{
int totalAbsentDays = 0;
int id, absentDays;
//open file
outfile.open("c:\cmsc140\employeeAbsences.txt ");
for (int i = 1; i <= numberOfEmployees; i++)
{
cout << "Enter Employee" << i << " id: ";
cin >> id;
cout << "Enter Number of absent days for Employee" << i << ": ";
cin >> absentDays;
//Write data to file
outfile << id << " " << absentDays << endl;
//Calculate total
totalAbsentDays += absentDays;
}
outfile.close();
return totalAbsentDays;
}
int NumOfEmployees()
{
cout << "Enter number of employees: ";
int emp;
cin >> emp;
return emp;
}
int main()
{
int numberOfEmployees = NumOfEmployees();
int totalDaysAbsent = TotDaysAbsent(numberOfEmployees);
double avgAbsent = AverageAbsent(numberOfEmployees, totalDaysAbsent);
cout << "Average Absent: " << avgAbsent << endl;
return 0;
}

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 4 images

- flowchrt for the code below //C++ Code #include<iostream>#include<fstream>using namespace std;/*Create a global variable of type ofstream for the output file*/ofstream outfile; /** This function asks the user for the number of employees in the company. This value should be returned as an int. The function accepts no arguments (No parameter/input).*/int NumOfEmployees();/** accepts an argument of type int for the number of employees in the company and returns the total of missed days as an int. This function should do the following:Asks the user to enter the following information for each employee:The employee number (ID) (Assume the employee number is 4 digits or fewer, but don't validate it).The number of days that employee missed during the past year.Writes each employee number (ID) and the number of days missed to the output file */int TotDaysAbsent(int numberOfEmployees);/* calculates the average number of days absent.The function takes two arguments:the number of…arrow_forwardA local variable is a variable defined in another file included in the program specifically inside the main function inside any function outside any functionarrow_forwardFile Handling Assignment -2 Write a program to generate a file numbers.txt that contains the first 50 odd natural numbers and each line in the file should contain 5 numbers only. Attach the screenshot of numbers.txt. In C or C++arrow_forward
- User Input Program and Analysis (Last answer to this question was incorrect.) Demonstrate an understanding of C++ programming concepts by completing the following: Program: Create a C++ program that will obtain input from a user and store it into the provided CSC450_CT5_mod5.txt Download CSC450_CT5_mod5.txtfile. Your program should append it to the provided text file, without deleting the existing data: Store the provided data in the CSC450_CT5_mod5.txt file. Create a reversal method that will reverse all of the characters in the CSC450_CT5_mod5.txt file and store the result in a CSC450-mod5-reverse.txt file. Program Analysis: Given your program implementation, discuss and identify the possible security vulnerabilities that may exist. If present, discuss solutions to minimize the vulnerabilities. Discuss and identify possible problems that can result in errors for string manipulation of data. Your analysis should be 1-2 pages in length. TXT FILE INFORMATION (Txt file should NOT…arrow_forward// Flowers.cpp - This program reads names of flowers and whether they are grown in shade or sun from an input // file and prints the information to the user's screen. // Input: flowers.dat. // Output: Names of flowers and the words sun or shade. #include <fstream> #include <iostream> #include <string> using namespace std; int main() { // Declare variables here // Open input file // Write while loop that reads records from file. fin >> flowerName; // Print flower name using the following format //cout << var << " grows in the " << var2 << endl; fin.close(); return 0; } // End of main functionarrow_forwardFile redirection allows you to redirect standard input (keyboard) and instead read from a file specified at the command prompt. It requires the use of the < operator (e.g. java MyProgram < input_file.txt) True or Falsearrow_forward
- Create the pseudocode for a program that will Create a file of functions and place them into a functions.py file Make a new program called CallFunctions and import the functions.py file Call each of the functions in the functions filearrow_forward#function read file and display reportdef displayAverage(fileName):#open the fileinfile = open(fileName, "r")#variable declaration and initializationtotal = 0count = 0#read filefor num in infile:total = total + float(num)count = count + 1#close the fileinfile.close()#calculate averageaverage = total / count#display the resultprint("Average {:.1f}".format(average)) #method callingdisplayAverage("numbers.txt")arrow_forward// Flowers.cpp - This program reads names of flowers and whether they are grown in shade or sun from an input // file and prints the information to the user's screen. // Input: flowers.dat. // Output: Names of flowers and the words sun or shade. #include <fstream> #include <iostream> #include <string> using namespace std; int main() { // Declare variables here // Open input file // Write while loop that reads records from file. fin >> flowerName; // Print flower name using the following format //cout << var << " grows in the " << var2 << endl; fin.close(); return 0; } // End of main functionarrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
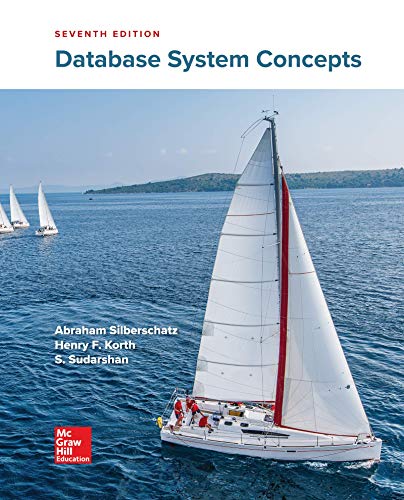
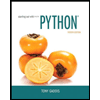
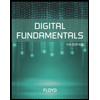
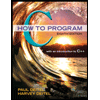
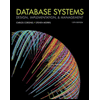
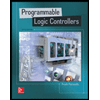