e. Problem 5. Devise a function that receives a string and computes how many asterisks are in the string with a recursive method. For instance, if the input is "Able was I ere 1 *** Elba", the function should output 3. If the input is "Peanuts", the function should return 0. Approach: Let's assume the signature of our function is countAsterisks(s) where s is the input string. In the body of your function, check if s[0] is equal to an asterisk. If the string contains only a single character and it is an asterisk, return 1. If the string contains only a single character and it is not an asterisk, return 0. If the string is longer than one character, determine if the first character is an asterisk and count it if this is the case. Then, create a new string s1 that is the same as the input string s but without the first character. Recursively call countAsterisks (s1) and return the value returned from this recursive call, plus one (if there is an asterisk in s[0]). Also, writing any explicit loop in your code results a 0 for this question. As ever, don't forget to provide pre- and post- conditions. Now copy the trace table on the next page in your Word file and trace your function countAsterisks (n) for when n is initially “P**nut*”. As a guideline, we have populated the tables with the values for the first calls and the corresponding returned values for each of those calls. call# S value returned to this 1 2 "P**nut*" "** "nut*” call 3 3 Task 1: In this task, you will complete four recursive functions and trace them for some sample inputs. a. Create an HTML file that includes five buttons with the following labels: "recursive find A", "recursive find B", "recursive find C", "recursive palindrome", and "recursive count asterisks”. Create a div in your HTML to hold the results of calls to corresponding javascript methods. You can style this page as you like. Feel free to use the HTML and CSS from prior labs as a template, if you like. b. Create a JavaScript file that responds to clicks of these buttons. All implementations should be recursive. a. Problem 1. Create an array of 30 random numbers that range between 1 and 100. Then, write a function that will receive a number from the user and determine if that number exists in the array or not. For instance, assume the array is: [2, 93, 14, 89, 12, 3, 81, 15, 14, 89, 52, 96, 71, 82, 5, 2, 41, 23, 52, 59, 44, 44, 88, 39, 49, 50, 97, 45, 48, 36] Now, assume the user enters 89, the program should output true. But, if the user enters 77, the program should output false. Approach: We will be implementing this method in two different ways. Both will be recursive. First, implement a method called findA (x,A), where x is the number we are looking for and A is an array. In the body of the function, compare x with the FIRST item that is in the array. If this first item is equal to X, return true. If not, remove the first item from A and call findA(x,A) on the revised list. If you call find on an empty list, you will want to return false. Writing any explicit loop in your code results a 0 for this question. Remember to provide pre- and post-conditions. How many recursive calls will you need to search the entire list? Next, copy the following trace table in your Word file and trace the input values to the function findA(x,A) for when A and x are initially [1, 6, 10, 14, 77, 82, 100] and 77, respectively. As a guideline, we have populated the tables with the values for the first 2 calls and the corresponding returned values for each of those calls. Note that in this example, the call numbers will be populated top down (i.e. time elapses from row x to row x+1) while returned values are populated bottom-up (i.e. the value in row x is returned before the value in rowx-1). You may want to use console.log to observe these values when you write your solution. call# x A value returned to this call 1 77 [1, 6, 10, 14, 77, 82, 100] TRUE 2 77 [6, 10, 14, 77, 82, 100] TRUE
e. Problem 5. Devise a function that receives a string and computes how many asterisks are in the string with a recursive method. For instance, if the input is "Able was I ere 1 *** Elba", the function should output 3. If the input is "Peanuts", the function should return 0. Approach: Let's assume the signature of our function is countAsterisks(s) where s is the input string. In the body of your function, check if s[0] is equal to an asterisk. If the string contains only a single character and it is an asterisk, return 1. If the string contains only a single character and it is not an asterisk, return 0. If the string is longer than one character, determine if the first character is an asterisk and count it if this is the case. Then, create a new string s1 that is the same as the input string s but without the first character. Recursively call countAsterisks (s1) and return the value returned from this recursive call, plus one (if there is an asterisk in s[0]). Also, writing any explicit loop in your code results a 0 for this question. As ever, don't forget to provide pre- and post- conditions. Now copy the trace table on the next page in your Word file and trace your function countAsterisks (n) for when n is initially “P**nut*”. As a guideline, we have populated the tables with the values for the first calls and the corresponding returned values for each of those calls. call# S value returned to this 1 2 "P**nut*" "** "nut*” call 3 3 Task 1: In this task, you will complete four recursive functions and trace them for some sample inputs. a. Create an HTML file that includes five buttons with the following labels: "recursive find A", "recursive find B", "recursive find C", "recursive palindrome", and "recursive count asterisks”. Create a div in your HTML to hold the results of calls to corresponding javascript methods. You can style this page as you like. Feel free to use the HTML and CSS from prior labs as a template, if you like. b. Create a JavaScript file that responds to clicks of these buttons. All implementations should be recursive. a. Problem 1. Create an array of 30 random numbers that range between 1 and 100. Then, write a function that will receive a number from the user and determine if that number exists in the array or not. For instance, assume the array is: [2, 93, 14, 89, 12, 3, 81, 15, 14, 89, 52, 96, 71, 82, 5, 2, 41, 23, 52, 59, 44, 44, 88, 39, 49, 50, 97, 45, 48, 36] Now, assume the user enters 89, the program should output true. But, if the user enters 77, the program should output false. Approach: We will be implementing this method in two different ways. Both will be recursive. First, implement a method called findA (x,A), where x is the number we are looking for and A is an array. In the body of the function, compare x with the FIRST item that is in the array. If this first item is equal to X, return true. If not, remove the first item from A and call findA(x,A) on the revised list. If you call find on an empty list, you will want to return false. Writing any explicit loop in your code results a 0 for this question. Remember to provide pre- and post-conditions. How many recursive calls will you need to search the entire list? Next, copy the following trace table in your Word file and trace the input values to the function findA(x,A) for when A and x are initially [1, 6, 10, 14, 77, 82, 100] and 77, respectively. As a guideline, we have populated the tables with the values for the first 2 calls and the corresponding returned values for each of those calls. Note that in this example, the call numbers will be populated top down (i.e. time elapses from row x to row x+1) while returned values are populated bottom-up (i.e. the value in row x is returned before the value in rowx-1). You may want to use console.log to observe these values when you write your solution. call# x A value returned to this call 1 77 [1, 6, 10, 14, 77, 82, 100] TRUE 2 77 [6, 10, 14, 77, 82, 100] TRUE
COMPREHENSIVE MICROSOFT OFFICE 365 EXCE
1st Edition
ISBN:9780357392676
Author:FREUND, Steven
Publisher:FREUND, Steven
Chapter5: Working With Multiple Worksheets And Workbooks
Section: Chapter Questions
Problem 4AYK
Related questions
Question
Please help me with these two questions. I am having trouble understanding what to do
Just provide the code. Use Js, css, and html
Thank you
![e. Problem 5. Devise a function that receives a string and computes how many asterisks
are in the string with a recursive method. For instance, if the input is "Able was I ere
1 *** Elba", the function should output 3. If the input is "Peanuts", the function should
return 0.
Approach: Let's assume the signature of our function is countAsterisks(s) where s
is the input string. In the body of your function, check if s[0] is equal to an asterisk. If
the string contains only a single character and it is an asterisk, return 1. If the string
contains only a single character and it is not an asterisk, return 0. If the string is
longer than one character, determine if the first character is an asterisk and count it if
this is the case. Then, create a new string s1 that is the same as the input string s
but without the first character. Recursively call countAsterisks (s1) and return the
value returned from this recursive call, plus one (if there is an asterisk in s[0]).
Also, writing any explicit loop in your code results a 0 for this question. As ever, don't
forget to provide pre- and post- conditions.
Now copy the trace table on the next page in your Word file and trace your function
countAsterisks (n) for when n is initially “P**nut*”. As a guideline, we have
populated the tables with the values for the first calls and the corresponding returned
values for each of those calls.
call#
S
value returned to this
1
2
"P**nut*"
"**
"nut*”
call
3
3](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fe3935a4e-65b5-4e47-bf60-1d363b9e3dcc%2F4dfdb929-10cc-4080-8bdd-7ba51c6a56e3%2Fkiwkit_processed.png&w=3840&q=75)
Transcribed Image Text:e. Problem 5. Devise a function that receives a string and computes how many asterisks
are in the string with a recursive method. For instance, if the input is "Able was I ere
1 *** Elba", the function should output 3. If the input is "Peanuts", the function should
return 0.
Approach: Let's assume the signature of our function is countAsterisks(s) where s
is the input string. In the body of your function, check if s[0] is equal to an asterisk. If
the string contains only a single character and it is an asterisk, return 1. If the string
contains only a single character and it is not an asterisk, return 0. If the string is
longer than one character, determine if the first character is an asterisk and count it if
this is the case. Then, create a new string s1 that is the same as the input string s
but without the first character. Recursively call countAsterisks (s1) and return the
value returned from this recursive call, plus one (if there is an asterisk in s[0]).
Also, writing any explicit loop in your code results a 0 for this question. As ever, don't
forget to provide pre- and post- conditions.
Now copy the trace table on the next page in your Word file and trace your function
countAsterisks (n) for when n is initially “P**nut*”. As a guideline, we have
populated the tables with the values for the first calls and the corresponding returned
values for each of those calls.
call#
S
value returned to this
1
2
"P**nut*"
"**
"nut*”
call
3
3
![Task 1: In this task, you will complete four recursive functions and trace them for some sample inputs.
a.
Create an HTML file that includes five buttons with the following labels: "recursive find A",
"recursive find B", "recursive find C", "recursive palindrome", and "recursive count
asterisks”. Create a div in your HTML to hold the results of calls to corresponding javascript
methods. You can style this page as you like. Feel free to use the HTML and CSS from prior
labs as a template, if you like.
b. Create a JavaScript file that responds to clicks of these buttons. All implementations should
be recursive.
a. Problem 1. Create an array of 30 random numbers that range between 1 and 100.
Then, write a function that will receive a number from the user and determine if
that number exists in the array or not.
For instance, assume the array is:
[2, 93, 14, 89, 12, 3, 81, 15, 14, 89, 52, 96, 71, 82, 5, 2, 41, 23, 52, 59, 44, 44, 88, 39,
49, 50, 97, 45, 48, 36]
Now, assume the user enters 89, the program should output true. But, if the user
enters 77, the program should output false.
Approach:
We will be implementing this method in two different ways. Both will be recursive.
First, implement a method called findA (x,A), where x is the number we are looking
for and A is an array. In the body of the function, compare x with the FIRST item that
is in the array. If this first item is equal to X, return true. If not, remove the first item
from A and call findA(x,A) on the revised list. If you call find on an empty list, you
will want to return false.
Writing any explicit loop in your code results a 0 for this question. Remember to provide
pre- and post-conditions. How many recursive calls will you need to search the entire
list?
Next, copy the following trace table in your Word file and trace the input values to
the function findA(x,A) for when A and x are initially [1, 6, 10, 14, 77, 82, 100]
and 77, respectively. As a guideline, we have populated the tables with the values for
the first 2 calls and the corresponding returned values for each of those calls. Note
that in this example, the call numbers will be populated top down (i.e. time elapses
from row x to row x+1) while returned values are populated bottom-up (i.e. the
value in row x is returned before the value in rowx-1). You may want to use
console.log to observe these values when you write your solution.
call#
x
A
value returned to
this call
1
77
[1, 6, 10, 14, 77, 82, 100]
TRUE
2
77
[6, 10, 14, 77, 82, 100]
TRUE](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fe3935a4e-65b5-4e47-bf60-1d363b9e3dcc%2F4dfdb929-10cc-4080-8bdd-7ba51c6a56e3%2Fe70at7q_processed.png&w=3840&q=75)
Transcribed Image Text:Task 1: In this task, you will complete four recursive functions and trace them for some sample inputs.
a.
Create an HTML file that includes five buttons with the following labels: "recursive find A",
"recursive find B", "recursive find C", "recursive palindrome", and "recursive count
asterisks”. Create a div in your HTML to hold the results of calls to corresponding javascript
methods. You can style this page as you like. Feel free to use the HTML and CSS from prior
labs as a template, if you like.
b. Create a JavaScript file that responds to clicks of these buttons. All implementations should
be recursive.
a. Problem 1. Create an array of 30 random numbers that range between 1 and 100.
Then, write a function that will receive a number from the user and determine if
that number exists in the array or not.
For instance, assume the array is:
[2, 93, 14, 89, 12, 3, 81, 15, 14, 89, 52, 96, 71, 82, 5, 2, 41, 23, 52, 59, 44, 44, 88, 39,
49, 50, 97, 45, 48, 36]
Now, assume the user enters 89, the program should output true. But, if the user
enters 77, the program should output false.
Approach:
We will be implementing this method in two different ways. Both will be recursive.
First, implement a method called findA (x,A), where x is the number we are looking
for and A is an array. In the body of the function, compare x with the FIRST item that
is in the array. If this first item is equal to X, return true. If not, remove the first item
from A and call findA(x,A) on the revised list. If you call find on an empty list, you
will want to return false.
Writing any explicit loop in your code results a 0 for this question. Remember to provide
pre- and post-conditions. How many recursive calls will you need to search the entire
list?
Next, copy the following trace table in your Word file and trace the input values to
the function findA(x,A) for when A and x are initially [1, 6, 10, 14, 77, 82, 100]
and 77, respectively. As a guideline, we have populated the tables with the values for
the first 2 calls and the corresponding returned values for each of those calls. Note
that in this example, the call numbers will be populated top down (i.e. time elapses
from row x to row x+1) while returned values are populated bottom-up (i.e. the
value in row x is returned before the value in rowx-1). You may want to use
console.log to observe these values when you write your solution.
call#
x
A
value returned to
this call
1
77
[1, 6, 10, 14, 77, 82, 100]
TRUE
2
77
[6, 10, 14, 77, 82, 100]
TRUE
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps

Recommended textbooks for you
COMPREHENSIVE MICROSOFT OFFICE 365 EXCE
Computer Science
ISBN:
9780357392676
Author:
FREUND, Steven
Publisher:
CENGAGE L
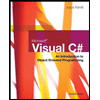
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
COMPREHENSIVE MICROSOFT OFFICE 365 EXCE
Computer Science
ISBN:
9780357392676
Author:
FREUND, Steven
Publisher:
CENGAGE L
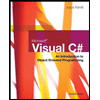
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,