Task 4- Aquarium (Topics: classes/objects, flow-control, string formatting, multi-file, collections This task simulates a text-based aquarium with five random "sea creatures" that move back and forth on the screen. This task uses the class defined in the utilities.py file named Sealife. The class has already defined several class variables that are useful to perform this task. You need to complete the class definition as follows: Sealife class Class data (already defined for you). category = ["fish1", "fish2", "jelly", "crab"] draw = { "fish1":["><>", "<><"], "fish2":[">()", "()<"], "jelly":["~>)", "(<~"], "crab":["^_^", "^_^"] } # categories of sea life # characters used for each category # and for the two directions e or 1 speed_range = {"fish1":(5,10), "fish2":(1,10), "jelly":(1,5), "crab":(10,20)} # speed ranges Instance data (4) cat a string that records what category an object is: fish1, fish2, jelly, or crab direction an integer that is 0 or 1 to indicate the direction the object is moving -- see move() and getStr() a float that represents the sea life's current position a float between (0.1- 2.0] depending on the category that is the object's speed -- see_init_() pos speed Methods (3) _init_(params: direction, pos) - assert if direction isn't 1 or 0 with error message "direction must be 0 or 1". - assert if pos isn't greater than 0 and less than 39 with error message "pos must be between 1 and 38". - set instance variables direction and pos to the parameters direction and pos. two parameters for the starting direction and position. - randomly set instance variables cat to be "fish1", "fish2", "jelly", or "crab". - Depending on the category, look up the speed range in the class variable speed_range and set instance variable speed to a random int between the speed range for the object's category and divide by 10. For example, if the category for this object is "crab", look up the speed range in the speed_range class dictionary. The speed range for crab is (10, 20), so set speed to a random int between 10 and 20 and then divide by 10 (so a crab's speed is between 1.0 and 2.0). If the category is "jelly", set speed to random int between 1 and 5 and divide by 10 (so a jelly's speed is between 0.1 and 0.5). move() - if the direction is 0, set pos equal to pos + speed (i.e, moving from left to right) - if the direction is 1, set pos equal to pos - speed (i.e., moving from right to left) - if the pos is greater than 40, set direction to 1 (i.e., reverse direction) - if the pos is less than 1, set direction to 0 (i.e., reverse direction) no parameters; no return getStr() - this method returns a string that depicts the sea life moving in the aquarium. The string depends on the object's category and direction. The characters that you should draw are in the class dictionary variable draw. no parameters; returns a string -- see details below - Example #1, if the object's category is "fish1" and direction is 0, then draw "><>" for the sea life. - Example #2, if the object's category is "jelly" and the direction is 1, then draw "(<~" for the sea life. - See the diagram below on how to format the string. For this example, assume the object's instance variables are as follows: int(pos) is 18 (recall, pos is a float, so int() is converting it to an integer) cat is "jelly", direction is 1 Return a string as follows: +"(<~"+', ;+'jelly' pos (18) spaces (42-pos) spaces cat category characters for direction 1
Task 4- Aquarium (Topics: classes/objects, flow-control, string formatting, multi-file, collections This task simulates a text-based aquarium with five random "sea creatures" that move back and forth on the screen. This task uses the class defined in the utilities.py file named Sealife. The class has already defined several class variables that are useful to perform this task. You need to complete the class definition as follows: Sealife class Class data (already defined for you). category = ["fish1", "fish2", "jelly", "crab"] draw = { "fish1":["><>", "<><"], "fish2":[">()", "()<"], "jelly":["~>)", "(<~"], "crab":["^_^", "^_^"] } # categories of sea life # characters used for each category # and for the two directions e or 1 speed_range = {"fish1":(5,10), "fish2":(1,10), "jelly":(1,5), "crab":(10,20)} # speed ranges Instance data (4) cat a string that records what category an object is: fish1, fish2, jelly, or crab direction an integer that is 0 or 1 to indicate the direction the object is moving -- see move() and getStr() a float that represents the sea life's current position a float between (0.1- 2.0] depending on the category that is the object's speed -- see_init_() pos speed Methods (3) _init_(params: direction, pos) - assert if direction isn't 1 or 0 with error message "direction must be 0 or 1". - assert if pos isn't greater than 0 and less than 39 with error message "pos must be between 1 and 38". - set instance variables direction and pos to the parameters direction and pos. two parameters for the starting direction and position. - randomly set instance variables cat to be "fish1", "fish2", "jelly", or "crab". - Depending on the category, look up the speed range in the class variable speed_range and set instance variable speed to a random int between the speed range for the object's category and divide by 10. For example, if the category for this object is "crab", look up the speed range in the speed_range class dictionary. The speed range for crab is (10, 20), so set speed to a random int between 10 and 20 and then divide by 10 (so a crab's speed is between 1.0 and 2.0). If the category is "jelly", set speed to random int between 1 and 5 and divide by 10 (so a jelly's speed is between 0.1 and 0.5). move() - if the direction is 0, set pos equal to pos + speed (i.e, moving from left to right) - if the direction is 1, set pos equal to pos - speed (i.e., moving from right to left) - if the pos is greater than 40, set direction to 1 (i.e., reverse direction) - if the pos is less than 1, set direction to 0 (i.e., reverse direction) no parameters; no return getStr() - this method returns a string that depicts the sea life moving in the aquarium. The string depends on the object's category and direction. The characters that you should draw are in the class dictionary variable draw. no parameters; returns a string -- see details below - Example #1, if the object's category is "fish1" and direction is 0, then draw "><>" for the sea life. - Example #2, if the object's category is "jelly" and the direction is 1, then draw "(<~" for the sea life. - See the diagram below on how to format the string. For this example, assume the object's instance variables are as follows: int(pos) is 18 (recall, pos is a float, so int() is converting it to an integer) cat is "jelly", direction is 1 Return a string as follows: +"(<~"+', ;+'jelly' pos (18) spaces (42-pos) spaces cat category characters for direction 1
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
In python, thank you
class variables needed for this task link https://pastebin.com/LH0u9ihn
![Task 4- Aquarium (Topics: classes/objects, flow-control, string formatting, multi-file, collections
This task simulates a text-based aquarium with five random "sea creatures" that move back and forth on the screen.
This task uses the class defined in the utilities.py file named Sealife. The class has already defined several class
variables that are useful to perform this task. You need to complete the class definition as follows:
Sealife class
Class data (already defined for you).
# categories of sea life
# characters used for each category
category = ["fish1", "fish2", "jelly", "crab"]
{ "fish1":["><>", "<><"], "fish2":[">()", "()<"],
"jelly":["~>)", "(<~"], "crab":["^_^", "^_^"] }
{"fish1":(5,10), "fish2":(1,10), "jelly":(1,5), "crab":(10,20)}
draw =
# and for the two directions e or 1
speed_range =
# speed ranges
Instance data (4)
cat
a string that records what category an object is: fish1, fish2, jelly, or crab
direction
an integer that is 0 or 1 to indicate the direction the object is moving -- see move() and getStr()
pos
a float that represents the sea life's current position
speed
a float between [0.1–2.0] depending on the category that is the object's speed - see_init_()
Methods (3)
_init_(params: direction, pos)
- assert if direction isn't 1 or 0 with error message "direction must be 0 or 1".
two parameters for the starting direction and position.
- assert if pos isn't greater than 0 and less than 39 with error message "pos must be between 1 and 38".
- set instance variables direction and pos to the parameters direction and pos.
- randomly set instance variables cat to be "fish1", "fish2", "jelly", or "crab".
- Depending on the category, look up the speed range in the class variable speed_range
and set instance variable speed to a random int between the speed range for the object's category and divide by 10.
For example, if the category for this object is "crab", look up the speed range in the speed_range class dictionary.
The speed range for crab is (10, 20), so set speed to a random int between 10 and 20 and then divide by 10 (so a crab's
speed is between 1.0 and 2.0). If the category is "jelly", set speed to random int between 1 and 5 and divide by 10
(so a jelly's speed is between 0.1 and 0.5).
move()
- if the direction is 0, set pos equal to pos + speed (i..e, moving from left to right)
no parameters; no return
- if the direction is 1, set pos equal to pos - speed (i.e., moving from right to left)
- if the pos is greater than 40, set direction to 1 (i.e., reverse direction)
- if the pos is less than 1, set direction to 0 (i.e., reverse direction)
getStr()
no parameters; returns a string - see details below
- this method returns a string that depicts the sea life moving in the aquarium. The string depends on the object's
category and direction. The characters that you should draw are in the class dictionary variable draw.
- Example #1, if the object's category is "fish1" and direction is 0, then draw "><>" for the sea life.
- Example #2, if the object's category is "jelly" and the direction is 1, then draw"(<~" for the sea life.
- See the diagram below on how to format the string.
For this example, assume the object's instance variables are as follows:
int (pos) is 18 (recall, pos is a float, so int() is converting it to an integer)
cat is "jelly", direction is 1
Return a string as follows:
+'jelly'
pos (18) spaces
(42-pos) spaces
category
cat
characters for direction 1](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fbeb43acd-4bfb-4c49-84a8-7d6db7b72498%2Fd12af002-1f18-4814-8383-aa9074f44aea%2Fa7m33le_processed.png&w=3840&q=75)
Transcribed Image Text:Task 4- Aquarium (Topics: classes/objects, flow-control, string formatting, multi-file, collections
This task simulates a text-based aquarium with five random "sea creatures" that move back and forth on the screen.
This task uses the class defined in the utilities.py file named Sealife. The class has already defined several class
variables that are useful to perform this task. You need to complete the class definition as follows:
Sealife class
Class data (already defined for you).
# categories of sea life
# characters used for each category
category = ["fish1", "fish2", "jelly", "crab"]
{ "fish1":["><>", "<><"], "fish2":[">()", "()<"],
"jelly":["~>)", "(<~"], "crab":["^_^", "^_^"] }
{"fish1":(5,10), "fish2":(1,10), "jelly":(1,5), "crab":(10,20)}
draw =
# and for the two directions e or 1
speed_range =
# speed ranges
Instance data (4)
cat
a string that records what category an object is: fish1, fish2, jelly, or crab
direction
an integer that is 0 or 1 to indicate the direction the object is moving -- see move() and getStr()
pos
a float that represents the sea life's current position
speed
a float between [0.1–2.0] depending on the category that is the object's speed - see_init_()
Methods (3)
_init_(params: direction, pos)
- assert if direction isn't 1 or 0 with error message "direction must be 0 or 1".
two parameters for the starting direction and position.
- assert if pos isn't greater than 0 and less than 39 with error message "pos must be between 1 and 38".
- set instance variables direction and pos to the parameters direction and pos.
- randomly set instance variables cat to be "fish1", "fish2", "jelly", or "crab".
- Depending on the category, look up the speed range in the class variable speed_range
and set instance variable speed to a random int between the speed range for the object's category and divide by 10.
For example, if the category for this object is "crab", look up the speed range in the speed_range class dictionary.
The speed range for crab is (10, 20), so set speed to a random int between 10 and 20 and then divide by 10 (so a crab's
speed is between 1.0 and 2.0). If the category is "jelly", set speed to random int between 1 and 5 and divide by 10
(so a jelly's speed is between 0.1 and 0.5).
move()
- if the direction is 0, set pos equal to pos + speed (i..e, moving from left to right)
no parameters; no return
- if the direction is 1, set pos equal to pos - speed (i.e., moving from right to left)
- if the pos is greater than 40, set direction to 1 (i.e., reverse direction)
- if the pos is less than 1, set direction to 0 (i.e., reverse direction)
getStr()
no parameters; returns a string - see details below
- this method returns a string that depicts the sea life moving in the aquarium. The string depends on the object's
category and direction. The characters that you should draw are in the class dictionary variable draw.
- Example #1, if the object's category is "fish1" and direction is 0, then draw "><>" for the sea life.
- Example #2, if the object's category is "jelly" and the direction is 1, then draw"(<~" for the sea life.
- See the diagram below on how to format the string.
For this example, assume the object's instance variables are as follows:
int (pos) is 18 (recall, pos is a float, so int() is converting it to an integer)
cat is "jelly", direction is 1
Return a string as follows:
+'jelly'
pos (18) spaces
(42-pos) spaces
category
cat
characters for direction 1
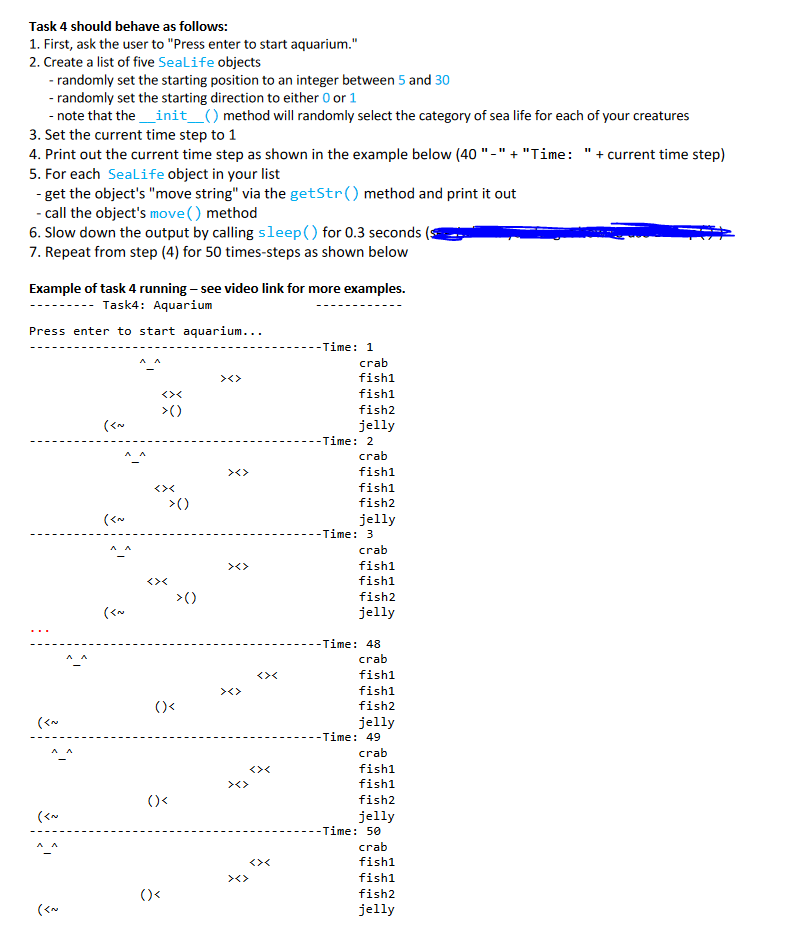
Transcribed Image Text:Task 4 should behave as follows:
1. First, ask the user to "Press enter to start aquarium."
2. Create a list of five Sealife objects
- randomly set the starting position to an integer between 5 and 30
- randomly set the starting direction to either 0 or 1
- note that the_init_() method will randomly select the category of sea life for each of your creatures
3. Set the current time step to 1
4. Print out the current time step as shown in the example below (40 "-" + "Time: "+ current time step)
5. For each Sealife object in your list
- get the object's "move string" via the getStr() method and print it out
- call the object's move() method
6. Slow down the output by calling sleep() for 0.3 seconds (
7. Repeat from step (4) for 50 times-steps as shown below
Example of task 4 running – see video link for more examples.
Task4: Aquarium
Press enter to start aquarium...
-Time: 1
crab
><>
fish1
fishl
>()
fish2
jelly
-Time: 2
crab
fish1
fish1
>()
fish2
jelly
-Time: 3
crab
fish1
<>
fishl
>()
fish2
jelly
-Time: 48
crab
fish1
><>
fish1
()<
fish2
jelly
-Time: 49
crab
<><
fish1
fish1
fish2
()<
jelly
--Time: 50
crab
fish1
fish1
()<
fish2
jelly
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
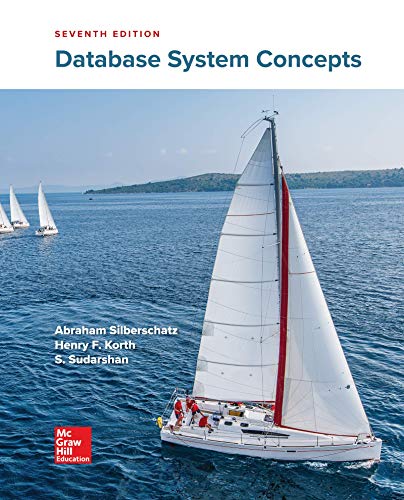
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
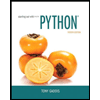
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
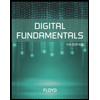
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
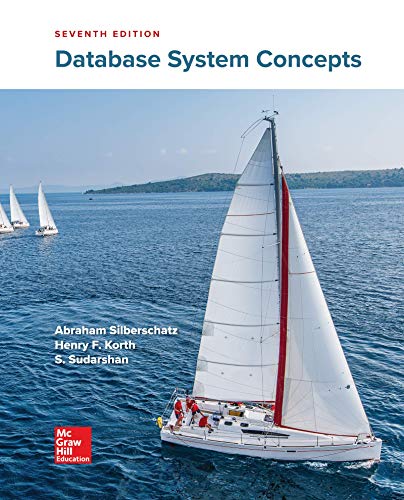
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
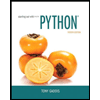
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
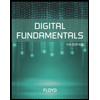
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
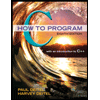
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
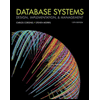
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
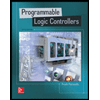
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education