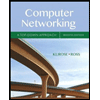
URGENT!!!
Add screenshot of output as well
using System;
using System.Collections.Generic;
namespace Proj1
{
//DO NOT add or delete the 'using' directives metioned above.
//DO NOT change existing classes and their methods.
//The expected output from this
//at the end of this file.
//Define the methods mySelect and myWhere.
//You may use a system-defined class in the body of these methods.
//WRITE CODE BELOW.
public class Employee
{
public string Name { get; set; }
public string Car { get; set; }
public Employee(string name, string car)
{ Name = name; Car = car; }
}
public class Student
{
public string Name { get; set; }
public string Course { get; set; }
public Student(string name, string course)
{ Name = name; Course = course; }
}
public class Program
{
private static IEnumerable<Employee> employees = new List<Employee>() {
new Employee("Abha", "Ford"),
new Employee("Krista", "Honda")
};
private static IEnumerable<Student> students = new List<Student>() {
new Student("Mellisa", "Math"),
new Student("Camila", "Bio")
};
private static void projectAll()
{
Console.WriteLine("************");
Console.WriteLine("projectAll: ");
Console.WriteLine("************");
//projects all attributes.
var query1 = employees.mySelect( (e) => e );
foreach (var item in query1)
{
Console.WriteLine(item.Name + " " + item.Car);
}
var query2 = students.mySelect((e) => e);
foreach (var item in query2)
{
Console.WriteLine(item.Name + " " + item.Course);
}
}
private static void filter()
{
Console.WriteLine("********");
Console.WriteLine("filter: ");
Console.WriteLine("********");
//filter on Car == "Honda"
var query1 = employees.myWhere( (e) => e.Car == "Honda" );
foreach (var item in query1)
{
Console.WriteLine(item.Name);
}
//filter on Course == "Math"
var query2 = students.myWhere((e) => e.Course == "Math");
foreach (var item in query2)
{
Console.WriteLine(item.Name);
}
}
public static void Main(string[] args)
{
projectAll();
Console.WriteLine("");
filter();
Console.WriteLine("");
Console.ReadKey(); //halt execution
}
}
}
/*
The output from the above program should be as follows.
************
projectAll:
************
Abha Ford
Krista Honda
Mellisa Math
Camila Bio
*************
projectName:
*************
Abha
Krista
Mellisa
Camila
********
filter:
********
Krista
Mellisa
*/

Step by stepSolved in 2 steps

- Helparrow_forwardMake any necessary modifications to the RushJob class so that it can be sorted by job number. Modify the JobDemo3 application so the displayed orders have been sorted. using System; using static System.Console; using System.Globalization; class JobDemo4 { static void Main() { RushJob[] jobs = new RushJob[5]; int x, y; double grandTotal = 0; bool goodNum; for(x = 0 ; x < jobs.Length; ++x) { jobs[x] = new RushJob(); Write("Enter job number "); jobs[x].JobNumber = Convert.ToInt32(ReadLine()); goodNum = true; for(y = 0; y < x; ++y) { if(jobs[x].Equals(jobs[y])) goodNum = false; } while(!goodNum) { Write("Sorry, the job number " + jobs[x].JobNumber + " is a duplicate. " + "\nPlease reenter "); jobs[x].JobNumber = Convert.ToInt32(ReadLine()); goodNum = true;…arrow_forwardPLZ help with the following: True/False In java it is possible to throw an exception, catch it, then re-throw that same exception if it is desired GUIs are windowing interfaces that handle user input and output. An interface can contain defined constants as well as method headings or instead of method headings. When a recursive call is encountered, computation is temporarily suspended; all of the information needed to continue the computation is saved and the recursive call is evaluated. Can you have a static method in a nonstatic inner class?arrow_forward
- Answer correctly with explaination No copy paste pleasearrow_forwardg) Make a class Class (as in a class of students, not a class as in a class of objects) that stores several Student objects. Store a list of students in an attribute called students. The initializer method should initialize the class as an empty class (no students). Implement a method add_student() that takes an object of class Student and adds it to class. Implement method pass_rate() that determines the fraction of students that pass the class (have a grade of >= 6). python code!!arrow_forward• RectangleTest class: o Perform the following actions in the main method: • Create an object named r1 of the Rectangle class using the default constructor. • Using the set methods, initialize rl to have width 3.0 and length 5.2. • Use the printDimensions method to output all the data (width & length) related to r1. • Create another object named r2 of the Rectangle class with width 2.6 and length 5.4 using the overloaded constructor. • Print out r2 (width & length) using the get methods. • Print out the area and the perimeter of r1 using the calculateArea and calculatePerimeter methods. • Print out the area and the perimeter of r2 using the calculateArea and calculatePerimeter methods.arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
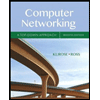
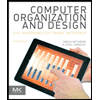
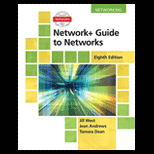
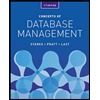
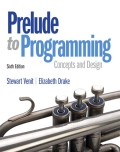
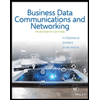