Using Classes Write a program that inputs, processes, and outputs a set of student records organized as a vector of class StudentRec objects. write a program where each student record is constructed using the class StudentRec. The variables in this case are "private" and the functions are "public." Note: only the first_name and last_name variables will require "getter" functions that return the name in all caps: get_first_name_upper() and get_last_name_upper() functions. Your class declaration section should look something like this: class StudentRec
Using Classes Write a
- write a program where each student record is constructed using the class StudentRec.
- The variables in this case are "private" and the functions are "public."
- Note: only the first_name and last_name variables will require "getter" functions that return the name in all caps:
get_first_name_upper() and get_last_name_upper() functions.
- Your class declaration section should look something like this:
class StudentRec
{
private:
string last_name = ""; // Last name
string first_name = ""; // First name
int year_grad = 0; // Year expected to graduate
float gpa = 0.0; // Current gpa
public:
void set_last_name(string last_name_param);
string get_last_name() const;
string get_last_name_upper() const;
// the rest of the "setter" and "getter" functions for each variable above go here
}; // NOTE: a class declaration ends with a semicolon
- The program should ask for the data to fill a studentRec of structure StudentRec and then ask y/n if they want to add another studentRec.
- Each studentRec will go into the vector of type StudentRec called student_list.
- After the student records have been entered into the vector student_list, find the average gpa of all the students in the vector.
- Output all the student records and give the average gpa for the students. Use the iomanip tools to make the data look as nice a possible.

Code:
/******************************************************************************
Online C++ Compiler.
Code, Compile, Run and Debug C++ program online.
Write your code in this editor and press "Run" button to compile and execute it.
*******************************************************************************/
#include <iostream>
using namespace std;
class StudentRec {
private:
string last_name = "";
string first_name = "";
int year_grad = 0;
float gpa = 0.0;
public:
StudentRec(string ln,string fn,int yg,float g) {
last_name = ln;
first_name = fn;
year_grad = yg;
gpa = g;
}
void display() {
cout << last_name<< ", "<<first_name<< ", "<<year_grad<< ", "<<gpa << endl;
}
void set_last_name(string last_name_param){
last_name=last_name_param;
}
string get_last_name() const{
return(last_name);
}
string get_last_name_upper() const{
return(last_name);
}
};
int main ()
{
StudentRec sai("chetla","sai",2021, 84);
sai.display();
cout << endl;
sai.set_last_name("tree");
sai.display();
return 0;
}
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

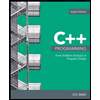
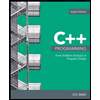