File Stream Problem: Implement a Caesar cipher that can encrypt and decrypt text and file. A Caesar cipher is a substitution cipher that shifts the letters in the original message x spaces ascending the alphabet where x is the key. Example if the key for the cipher is 3, and the message is “I will be there.” Then the coded message would be “L zloo eh wkhuh” Program requirements: Create a class call CCipher that has two member functions encrypt and decrypt. The program should be able to encrypt/decrypt a message from a file as well as a keyboard input. The default key should be 3. The user should be able to select between encrypting and decrypting and should be allowed to do so multiple times until s/he decides to end the program. The file format to be encrypted should be a “.txt” and the user should be able to provide the name of the file to be encrypted, after which the file should be deleted. The user should have the option to view and/or save an encrypted/decrypted message. If no file name was specified, the encrypted message should be saved as “encrypt.dat”. If no file name was specified, the decrypted message should be saved as “decrypt.txt”. N.B: THIS SHOULD BE A C++ PROGRAM
File Stream Problem: Implement a Caesar cipher that can encrypt and decrypt text and file. A Caesar cipher is a substitution cipher that shifts the letters in the original message x spaces ascending the alphabet where x is the key. Example if the key for the cipher is 3, and the message is “I will be there.” Then the coded message would be “L zloo eh wkhuh” Program requirements: Create a class call CCipher that has two member functions encrypt and decrypt. The program should be able to encrypt/decrypt a message from a file as well as a keyboard input. The default key should be 3. The user should be able to select between encrypting and decrypting and should be allowed to do so multiple times until s/he decides to end the program. The file format to be encrypted should be a “.txt” and the user should be able to provide the name of the file to be encrypted, after which the file should be deleted. The user should have the option to view and/or save an encrypted/decrypted message. If no file name was specified, the encrypted message should be saved as “encrypt.dat”. If no file name was specified, the decrypted message should be saved as “decrypt.txt”. N.B: THIS SHOULD BE A C++ PROGRAM
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
File Stream
Problem:
Implement a Caesar cipher that can encrypt and decrypt text and file. A Caesar cipher is a
substitution cipher that shifts the letters in the original message x spaces ascending the alphabet
where x is the key.
Example if the key for the cipher is 3, and the message is “I will be there.” Then the coded
message would be “L zloo eh wkhuh”
Program requirements:
- Create a class call CCipher that has two member functions encrypt and decrypt.
- The program should be able to encrypt/decrypt a message from a file as well as a
keyboard input.
- The default key should be 3.
- The user should be able to select between encrypting and decrypting and should be
allowed to do so multiple times until s/he decides to end the program.
- The file format to be encrypted should be a “.txt” and the user should be able to provide
the name of the file to be encrypted, after which the file should be deleted.
- The user should have the option to view and/or save an encrypted/decrypted message.
- If no file name was specified, the encrypted message should be saved as “encrypt.dat”.
- If no file name was specified, the decrypted message should be saved as “decrypt.txt”.
N.B: THIS SHOULD BE A C++ PROGRAM
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 6 images

Recommended textbooks for you
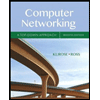
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
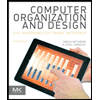
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
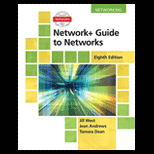
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
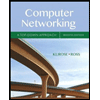
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
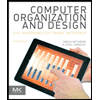
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
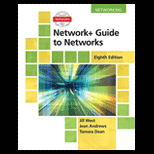
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
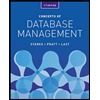
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
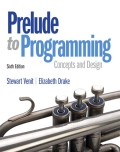
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
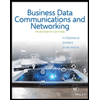
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY