Write the definition of a recursive function int simpleSqrt(int n) The function returns the integer square root of n, meaning the biggest integer whose square is less than or equal to n. You may assume that the function is always called with a nonnegative value for n. Use the following algorithm: If n is 0 then return 0. Otherwise, call the function recursively with n-1 as the argument to get a number t. Check whether or not t+1 squared is strictly greater than n. Based on that test, return the correct result. For example, a call to simpleSqrt(8) would recursively call simpleSqrt(7) and get back 2 as the answer. Then we would square (2+1) = 3 to get 9. Since 9 is bigger than 8, we know that 3 is too big, so return 2 in this case. On the other hand a call to simpleSqrt(9) would recursively call simpleSqrt(8) and get back 2 as the answer. Again we would square (2+1) = 3 to get back 9. So 3 is the correct return value in this case.
Write the definition of a recursive function int simpleSqrt(int n) The function returns the integer square root of n, meaning the biggest integer whose square is less than or equal to n. You may assume that the function is always called with a nonnegative value for n. Use the following algorithm: If n is 0 then return 0. Otherwise, call the function recursively with n-1 as the argument to get a number t. Check whether or not t+1 squared is strictly greater than n. Based on that test, return the correct result. For example, a call to simpleSqrt(8) would recursively call simpleSqrt(7) and get back 2 as the answer. Then we would square (2+1) = 3 to get 9. Since 9 is bigger than 8, we know that 3 is too big, so return 2 in this case. On the other hand a call to simpleSqrt(9) would recursively call simpleSqrt(8) and get back 2 as the answer. Again we would square (2+1) = 3 to get back 9. So 3 is the correct return value in this case.
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
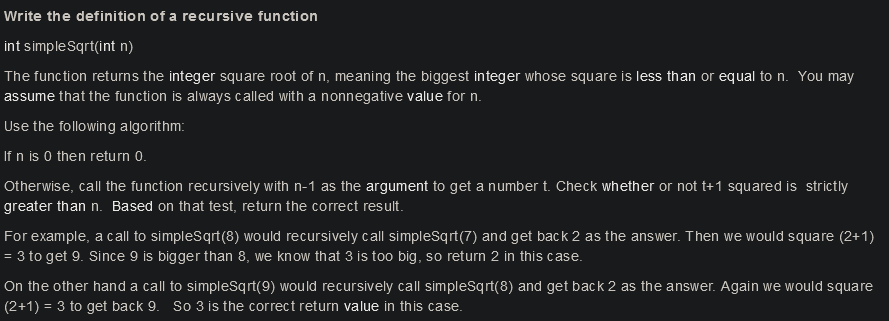
Transcribed Image Text:Write the definition of a recursive function
int simpleSqrt(int n)
The function returns the integer square root of n, meaning the biggest integer whose square is less than or equal to n. You may
assume that the function is always called with a nonnegative value for n.
Use the following algorithm:
If n is 0 then return 0.
Otherwise, call the function recursively with n-1 as the argument to get a number t. Check whether or not t+1 squared is strictly
greater than n. Based on that test, return the correct result.
For example, a call to simpleSqrt(8) would recursively call simpleSqrt(7) and get back 2 as the answer. Then we would square (2+1)
= 3 to get 9. Since 9 is bigger than 8, we know that 3 is too big, so return 2 in this case.
On the other hand a call to simpleSqrt(9) would recursively call simpleSqrt(8) and get back 2 as the answer. Again we would square
(2+1) = 3 to get back 9. So 3 is the correct return value in this case.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Recommended textbooks for you
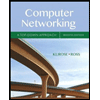
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
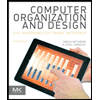
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
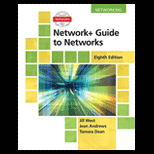
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
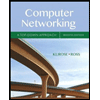
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
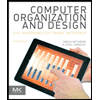
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
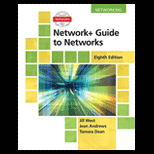
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
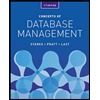
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
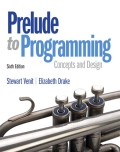
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
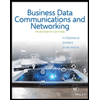
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY