The International Standard Book Number (ISBN) is a number that uniquely identifies a book. Publishers can buy or receive ISBNs from the International ISBN Agency. There are two types of ISBNs: ISBN-13 and ISBN-10, which are 10- and 13-digits long, respectively.ISBN-10 has a pattern, where the last digit can be found using the first 9. Additionally, ISBN-10 can also be used to calculate its ISBN-13 equivalent (and vice versa). Write two functions in ISBN.java. The main method is implemented for you so you can test your functions. Instructions on how to run this file to make use of the test.txt file are detailed below. calculateISBN10(int[] isbn) computes the last digit of the ISBN-10 number. The first 9 of the 10 digits of the ISBN will be passed to the function as the parameter isbn integer array of size 10. The last integer in the array will be -1. Your job is to calculate the last digit (called the check digit), and update the last index of the isbn array with the computed value. Here is how to compute the check digit: Step 1. multiply each isbn digit (starting at the first digit) by an integer weight (starting at 10 and descending to 2). Add all the products together, let’s call this number sumOfProducts. Step 2. Find the remainder when sumOfProducts is divided by 11, let’s call this number rem. Step 3. Find the difference between 11 and rem, call this number diff. Step 4. The check digit is the remainder of diff divided by 11. Update the last array index with this value. Let’s do an example. The 10-digit ISBN number for Pride and Prejudice by Jane Austen is 0141439513. Here is how the last digit 3 is computed: The isbn array will contain the first 9 digits 014143951: Step 1: (0*10) + (1*9) + (4*8) + (1*7) + (4*6) + (3*5) + (9*4) + (5*3) + (1*2) = 140 Step 2: 140 % 11 = 8 Step 3: 11 – 8 = 3 Step 4: 3 % 11 = 3 Our calculation gave us 3 as our check digit. Update the last index of the array with this value. Note 1: The check digit will be a number between 0 and 10. (Do not worry if it is a 10, as the main method will print it out as an “X” so as to not make it an 11-digit ISBN). Note 2: All of the 9 integers of the incomplete ISBN will be between indexes 0 and 9. In this function you are practicing updating the input array. Once calculateISBN10 is done executing, the array update made by the function is visible to the caller function. calculateISBN13(int[] shortIsbn) uses the parameter integer array shortIsbn to compute the ISBN-13 number. The integer array parameter, shortIsbn, is of size 10, it contains a ISBN-10 number. Your job is to create a new integer array of size 13, compute the ISBN-13 number, store the number in the created array and return it. Here is how to computer the ISBN-13 number: Step 1: The first 3 digits of an ISBN-13 are always 978. The rest of the 10 numbers will be the ISBN-10. This gives us a 13-digit number. Store all 13 digits into the array of size 13 you created. Recalculate the last digit (the check digit). Step 2: Multiply each of the first 12 digits with 1 or 3, alternatively. Add all the twelve products together, let’s call this number sumOfProducts. Step 3: Find the remainder when sumOfProducts divided by 10, let’s call this number rem. Step 4: If rem is 0 (zero), then the check digit is zero. If rem isn’t 0 (zero) the check digit is the difference between 10 and rem. Update the last array index with this value. (Step 4 UPDATED 10/24 10:15pm) Let’s do an example. The ISBN-13 of Pride and Prejudice by Jane Austen, is 9780141439518. Let us see how to compute this number. The method receives, through the shortIsbn array, the ISBN-10 for Pride and Prejudice by Jane Austen, which is – 0141439513: Step 1: Insert 978 in front the ISBN-10 number, 9780141439513. Store all 13 digits in the array size 13 you created. Step 2: (9*1) + (7*3) + (8*1) + (0*3) + (1*1) + (4*3) + (1*1) + (4*3) + (3*1) + (9*3) + (5*1) + (1*3) = 102 Step 3: 102 % 10 = 2 Step 4: 2 is not zero, so 10 – 2 = 8 (Step 4 UPDATED 10/24 10:15pm) Our calculation gave us 8 as our check digit. Update the last index of the array with this value. In this function you are practicing creating and returning a new array to the caller function. The main method is implemented for you to test your functions using the input file books.txt. Execute the program that reads the input file, and calls the two function as follows: java ISBN < books.txt
Control structures
Control structures are block of statements that analyze the value of variables and determine the flow of execution based on those values. When a program is running, the CPU executes the code line by line. After sometime, the program reaches the point where it has to make a decision on whether it has to go to another part of the code or repeat execution of certain part of the code. These results affect the flow of the program's code and these are called control structures.
Switch Statement
The switch statement is a key feature that is used by the programmers a lot in the world of programming and coding, as well as in information technology in general. The switch statement is a selection control mechanism that allows the variable value to change the order of the individual statements in the software execution via search.
The International Standard Book Number (ISBN) is a number that uniquely identifies a book. Publishers can buy or receive ISBNs from the International ISBN Agency. There are two types of ISBNs: ISBN-13 and ISBN-10, which are 10- and 13-digits long, respectively.ISBN-10 has a pattern, where the last digit can be found using the first 9. Additionally, ISBN-10 can also be used to calculate its ISBN-13 equivalent (and vice versa).
Write two functions in ISBN.java. The main method is implemented for you so you can test your functions. Instructions on how to run this file to make use of the test.txt file are detailed below.
-
-
calculateISBN10(int[] isbn) computes the last digit of the ISBN-10 number.The first 9 of the 10 digits of the ISBN will be passed to the function as the parameter isbn integer array of size 10. The last integer in the array will be -1. Your job is to calculate the last digit (called the check digit), and update the last index of the isbn array with the computed value. Here is how to compute the check digit:
- Step 1. multiply each isbn digit (starting at the first digit) by an integer weight (starting at 10 and descending to 2). Add all the products together, let’s call this number sumOfProducts.
- Step 2. Find the remainder when sumOfProducts is divided by 11, let’s call this number rem.
- Step 3. Find the difference between 11 and rem, call this number diff.
- Step 4. The check digit is the remainder of diff divided by 11. Update the last array index with this value.
Let’s do an example. The 10-digit ISBN number for Pride and Prejudice by Jane Austen is 0141439513. Here is how the last digit 3 is computed:
- The isbn array will contain the first 9 digits 014143951:
- Step 1: (0*10) + (1*9) + (4*8) + (1*7) + (4*6) + (3*5) + (9*4) + (5*3) + (1*2) = 140
- Step 2: 140 % 11 = 8
- Step 3: 11 – 8 = 3
- Step 4: 3 % 11 = 3
- Our calculation gave us 3 as our check digit. Update the last index of the array with this value.
-
-
- Note 1: The check digit will be a number between 0 and 10. (Do not worry if it is a 10, as the main method will print it out as an “X” so as to not make it an 11-digit ISBN).
- Note 2: All of the 9 integers of the incomplete ISBN will be between indexes 0 and 9.
- In this function you are practicing updating the input array. Once calculateISBN10 is done executing, the array update made by the function is visible to the caller function.
- calculateISBN13(int[] shortIsbn) uses the parameter integer array shortIsbn to compute the ISBN-13 number.
The integer array parameter, shortIsbn, is of size 10, it contains a ISBN-10 number. Your job is to create a new integer array of size 13, compute the ISBN-13 number, store the number in the created array and return it.
Here is how to computer the ISBN-13 number:
-
- Step 1: The first 3 digits of an ISBN-13 are always 978. The rest of the 10 numbers will be the ISBN-10. This gives us a 13-digit number. Store all 13 digits into the array of size 13 you created.
- Recalculate the last digit (the check digit).
- Step 2: Multiply each of the first 12 digits with 1 or 3, alternatively. Add all the twelve products together, let’s call this number sumOfProducts.
- Step 3: Find the remainder when sumOfProducts divided by 10, let’s call this number rem.
- Step 4: If rem is 0 (zero), then the check digit is zero. If rem isn’t 0 (zero) the check digit is the difference between 10 and rem. Update the last array index with this value. (Step 4 UPDATED 10/24 10:15pm)
-
- Let’s do an example.
- The ISBN-13 of Pride and Prejudice by Jane Austen, is 9780141439518. Let us see how to compute this number.
-
- The method receives, through the shortIsbn array, the ISBN-10 for Pride and Prejudice by Jane Austen, which is – 0141439513:
- Step 1: Insert 978 in front the ISBN-10 number, 9780141439513. Store all 13 digits in the array size 13 you created.
- Step 2: (9*1) + (7*3) + (8*1) + (0*3) + (1*1) + (4*3) + (1*1) + (4*3) + (3*1) + (9*3) + (5*1) + (1*3) = 102
- Step 3: 102 % 10 = 2
- Step 4: 2 is not zero, so 10 – 2 = 8 (Step 4 UPDATED 10/24 10:15pm)
- Our calculation gave us 8 as our check digit. Update the last index of the array with this value.
- The method receives, through the shortIsbn array, the ISBN-10 for Pride and Prejudice by Jane Austen, which is – 0141439513:
- In this function you are practicing creating and returning a new array to the caller function.
The main method is implemented for you to test your functions using the input file books.txt. Execute the program that reads the input file, and calls the two function as follows:
- java ISBN < books.txt
![public static void main(String[] args) {
// DO NOT EDIT THIS CODE
int[] isbn = new int [10];
int[] isbn_13 = new int [13];
int numOfBooks = StdIn. read Int ();
for (int i =
StdIn.readLine();
String name = StdIn.readLine();
System.out.println(name);
// Reads all the 9 integers
for (int j · 0; j < 9; j++ ) {
isbn [j] = StdIn. readInt ();
}
calculateISBN10 (isbn);
System.out.print(s:"ISBN-10 - ");
0; i < num0fBooks; i++) {
}
} else
// Enhanced for-loop to display the array that has been updated by calculateISBN10
for (int n : isbn) {
if (n == 10 ) {
System.out.print(s:"X");
System.out.println();
}
System.out.print(n);
System.out.print(s:"ISBN-13 - ");
}
// reads an integer from the file
isbn_13 = calculateISBN13(isbn); // calls calculateISBN13
System.out.print(s: "0");
System.out.print(n);
} else {
// reads an entire line from the file (the remaining of the line)
// reads an entire line from the file (the book's name)
// Enhanced for-loop to display the array that has been updated by calculateISBN13
for (int n : isbn_13) {
if (n == 10 ) {
// reads an integer from the file
System.out.println();
// calls calculateISBN10
Ln 1, Col 1](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fcbf3ab80-4492-450e-846b-8d4bf9bf5144%2Fefbc1e18-0d3c-4d7c-b074-cf45fc19319b%2Frudf5og_processed.png&w=3840&q=75)

Step by step
Solved in 4 steps

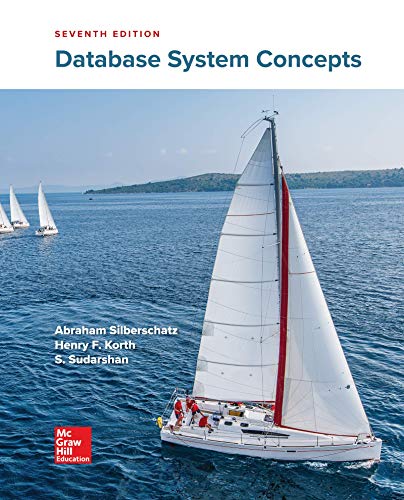
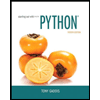
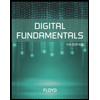
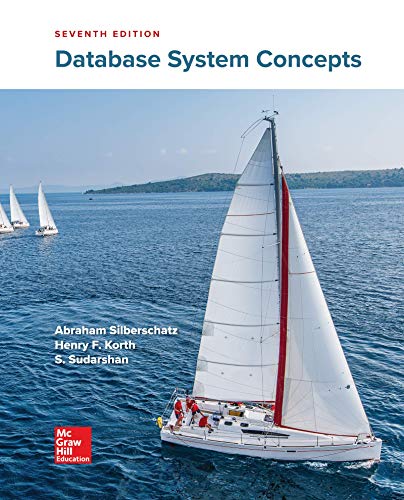
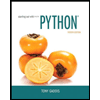
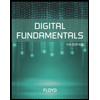
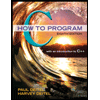
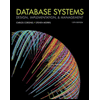
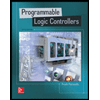