Write a Java class called HotelRoom that represents a hotel room. The class will contain the following attributes: •RoomNo (int) •PhoneNumber (string) •Capacity (int) – Number of people that room can accommodate. •Grade (int) – Grade of room ranging 1-5, 5 being most valuable. Your class should have a constructor that takes a single parameter: RoomNo. The necessary accessors (i.e. get methods) and mutators (i.e. set methods) for class variables: Room number only accessor Phone number both mutator and accessor Capacity both mutator and accessor Grade both mutator and accessor 2.Write a Java class called Hotel that represents a hotel. The class will contain the following attributes: •Name (string) •ReceptionPhone (string) •RoomList (ArrayList of HotelRoom's) hotel class should have a constructor that takes two parameters: Hotel name and reception phone. The necessary accessors (i.e. get methods) and mutators (i.e. set methods) for class variables: Hotel name only accessor Reception phone both mutator and accessor 2) Hotel class should have a public method addRoom that gets as parameter a Room object, and adds this Room object to RoomList: public void addRoom(HotelRoom room) Hotel class should have a public method getRoomCount that returns the number of rooms in the hotel (i.e. number of elements of RoomList): public static int getRoomCount() Hotel class should have a public method getRoom that returns the room from the room list given the index: public static HotelRoom getRoom(int index) Hotel class should have one public method in addition to the accessors and mutators: public String toString() It returns the information about the hotel rooms in the following format. ATTENTION: In this class, the toString method only returns the string representation of the rooms in the hotel, not whole information. 3) Write a Java application class that does the following: •Input a text file name entered by the user from keyboard. •Create a Hotel object that contains information read from a text file, using the readHotelmethod. Write a private static readHotel method that gets a file name as a parameter, reads the information about the hotel and rooms of the hotel from an input file and returns a Hotel object. In this method, create Hotel and HotelRoom objects as the input file is read, and insert HotelRoom objects to the ArrayList in the hotel object. private static Hotel readHotel(String filename) Format of the input file is assumed to be: a. The first line is the name of the hotel, b. The second line is the reception phone number of the hotel. Then several times c.Room no d.Phone number of rooms, e.The capacity of the room, 3 f.Quality (Grade) of room. Sample input file with two rooms (green text are comments, they do not exist in the actual input file): Examine the provided sample text file hotel.txt. hotel.txt = Crappy Hotel 911 101 91101 1 2 102 91102 2 1 103 91103 3 3 201 91201 4 2 202 91202 2 1 301 NoPhone 1 1
Write a Java class called HotelRoom that represents a hotel room. The class will contain the following attributes:
•RoomNo (int)
•PhoneNumber (string)
•Capacity (int) – Number of people that room can accommodate.
•Grade (int) – Grade of room ranging 1-5, 5 being most valuable.
Your class should have a constructor that takes a single parameter: RoomNo. The necessary accessors (i.e. get methods) and mutators (i.e. set methods) for class variables:
Room number only accessor
Phone number both mutator and accessor
Capacity both mutator and accessor Grade both mutator and accessor
2.Write a Java class called Hotel that represents a hotel. The class will contain the following attributes:
•Name (string)
•ReceptionPhone (string)
•RoomList (ArrayList of HotelRoom's)
hotel class should have a constructor that takes two parameters: Hotel name and reception phone. The necessary accessors (i.e. get methods) and mutators (i.e. set methods) for class variables:
Hotel name only accessor
Reception phone both mutator and accessor
- Hotel class should have a public method addRoom that gets as parameter a Room object, and adds this Room object to RoomList:
public void addRoom(HotelRoom room)
- Hotel class should have a public method getRoomCount that returns the number of rooms in the hotel (i.e. number of elements of RoomList): public static int getRoomCount()
- Hotel class should have a public method getRoom that returns the room from the room list given the index: public static HotelRoom getRoom(int index)
Hotel class should have one public method in addition to the accessors and mutators: public String toString() It returns the information about the hotel rooms in the following format.
ATTENTION: In this class, the toString method only returns the string representation of the rooms in the hotel, not whole information.
3) Write a Java application class that does the following:
•Input a text file name entered by the user from keyboard.
•Create a Hotel object that contains information read from a text file, using the readHotelmethod. Write a private static readHotel method that gets a file name as a parameter, reads the information about the hotel and rooms of the hotel from an input file and returns a Hotel object. In this method, create Hotel and HotelRoom objects as the input file is read, and insert HotelRoom objects to the ArrayList in the hotel object. private static Hotel readHotel(String filename) Format of the input file is assumed to be:
a. The first line is the name of the hotel, b. The second line is the reception phone number of the hotel. Then several times
c.Room no
d.Phone number of rooms, e.The capacity of the room,
3 f.Quality (Grade) of room. Sample input file with two rooms (green text are comments, they do not exist in the actual input file): Examine the provided sample text file hotel.txt.
hotel.txt =
Crappy Hotel
911
101
91101
1
2
102
91102
2
1
103
91103
3
3
201
91201
4
2
202
91202
2
1
301
NoPhone
1
1
![Your program code may look as follows:
. comment lines containing your name, surname, student-id and department
import java.util.Scanner;
import java.util.ArrayList;
import java.io.*;
public class Hw5_Lastname_Firstname
{
public static void main (String(] args) throws IOException
{
Hotel hotel = readHotel(infileName);
}
private static Hotel readHotel(String infileName) throws IOException
{
Hotel myHotel = new Hotel(hotelName,reception Phone);
return myHotel;
}
} // end class Hw5_Lastname_Firstname
class HotelRoom
{
....
class Hotel
{
....
| }](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F1cad1424-51d5-48f1-899b-bbbae8c5df58%2Ffc9ae1b7-aee7-4330-a326-dc6b15845b0a%2Ffmtbbed_processed.png&w=3840&q=75)
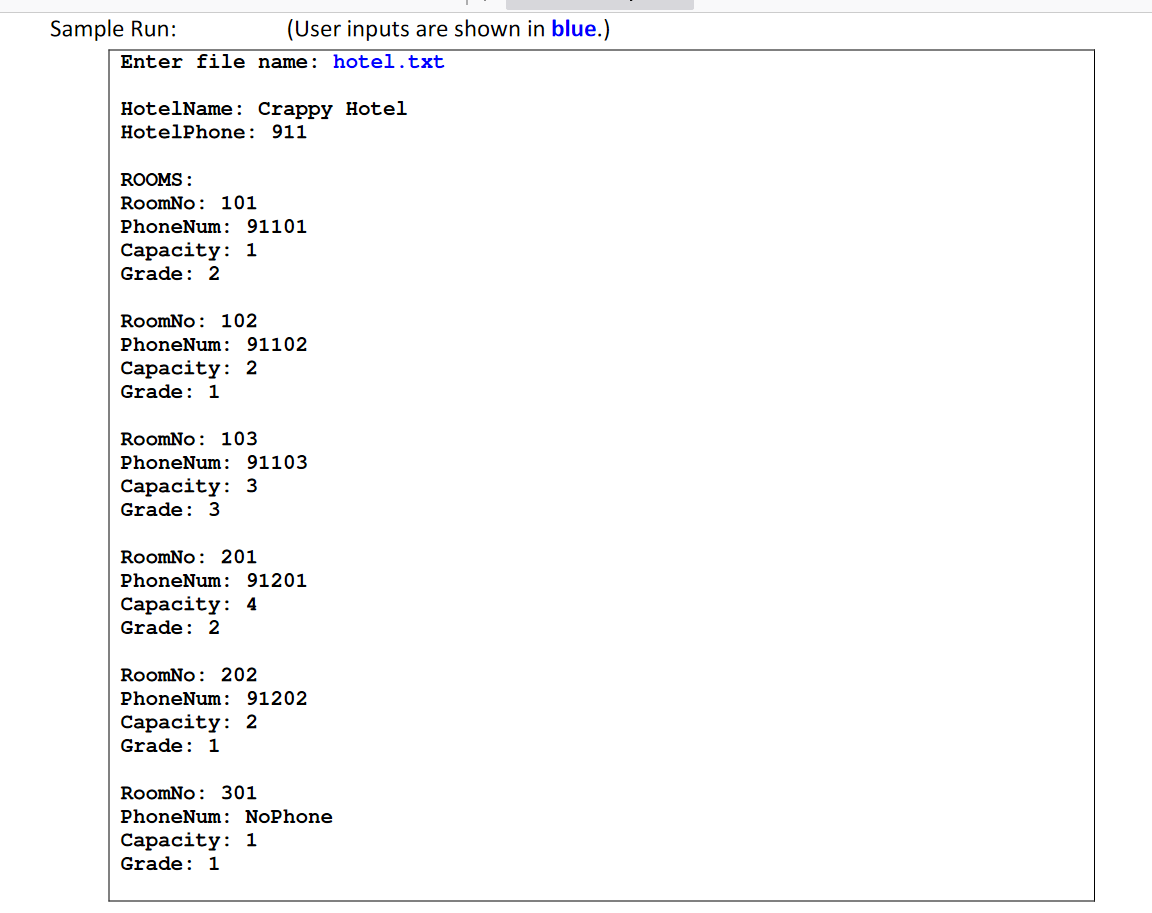

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 5 images

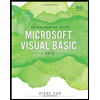
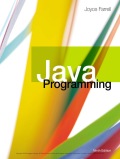
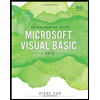
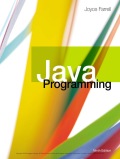
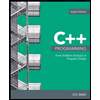