This code is a simple example of how threads can be used to speed up a * program in a multi-core system. Unfortunately it doesn't function properly. * The function run on thread creation wasn't finished, and thus the program * doesn't produce any results. Finish the function calculate_sum so that the * sum is correctly calculated. #Solve it in C language. #include #include #include #include #define NUMTHREADS 2 struct thread_data { int *valuelist; int num_values; int sum; }; void *calculate_sum(void *); int main(void) { int values[] = {1, 3, 5, 7, 9, 2, 6, 7, 0, 10, 11, 12, 13, 14, 15, 40, 50, 60, 61, 62}; int sum = 0; int *iter = values; pthread_t tids[NUMTHREADS]; /* Calculate how much data each thread will work on */ size_t num_values; num_values = sizeof(values) / sizeof(*values); int num_values_per_thread = num_values / NUMTHREADS; /** Standard C doesn't have variable length arrays. To allocate a dynamic * array, calloc can be used. This is different than dynamically creating a * list with multiple malloc calls. */ struct thread_data *d = calloc(NUMTHREADS, sizeof(struct thread_data)); for (int i = 0; i < NUMTHREADS; i++) { /* Using array notation changes -> into . */ d[i].sum = 0; d[i].num_values = num_values_per_thread; d[i].valuelist = calloc(num_values_per_thread, sizeof(int)); for (int j = 0; j < num_values_per_thread; j++) { fprintf(stderr, "assigning %d to thread %d \n", *iter, i); d[i].valuelist[j] = *iter; iter++; } fprintf(stderr, "\n"); } for (int i = 0; i < NUMTHREADS; i++) { pthread_create(&tids[i], NULL, calculate_sum, (void *)&d[i]); } for (int i = 0; i < NUMTHREADS; i++) { pthread_join(tids[i], NULL); sum += d[i].sum; } printf("Sum is: %d\n", sum); } void *calculate_sum(void *param) { struct thread_data *localdata = (struct thread_data *)param; int *iter = localdata->valuelist; /** Hint: The localdata pointer also has fields num_values and sum. */ pthread_exit(NULL); }
This code is a simple example of how threads can be used to speed up a * program in a multi-core system. Unfortunately it doesn't function properly. * The function run on thread creation wasn't finished, and thus the program * doesn't produce any results. Finish the function calculate_sum so that the * sum is correctly calculated.
#Solve it in C language.
#include <pthread.h> #include <stdio.h> #include <stdlib.h> #include <sys/types.h> #define NUMTHREADS 2 struct thread_data { int *valuelist; int num_values; int sum; }; void *calculate_sum(void *); int main(void) { int values[] = {1, 3, 5, 7, 9, 2, 6, 7, 0, 10, 11, 12, 13, 14, 15, 40, 50, 60, 61, 62}; int sum = 0; int *iter = values; pthread_t tids[NUMTHREADS]; /* Calculate how much data each thread will work on */ size_t num_values; num_values = sizeof(values) / sizeof(*values); int num_values_per_thread = num_values / NUMTHREADS; /** Standard C doesn't have variable length arrays. To allocate a dynamic * array, calloc can be used. This is different than dynamically creating a * list with multiple malloc calls. */ struct thread_data *d = calloc(NUMTHREADS, sizeof(struct thread_data)); for (int i = 0; i < NUMTHREADS; i++) { /* Using array notation changes -> into . */ d[i].sum = 0; d[i].num_values = num_values_per_thread; d[i].valuelist = calloc(num_values_per_thread, sizeof(int)); for (int j = 0; j < num_values_per_thread; j++) { fprintf(stderr, "assigning %d to thread %d \n", *iter, i); d[i].valuelist[j] = *iter; iter++; } fprintf(stderr, "\n"); } for (int i = 0; i < NUMTHREADS; i++) { pthread_create(&tids[i], NULL, calculate_sum, (void *)&d[i]); } for (int i = 0; i < NUMTHREADS; i++) { pthread_join(tids[i], NULL); sum += d[i].sum; } printf("Sum is: %d\n", sum); } void *calculate_sum(void *param) { struct thread_data *localdata = (struct thread_data *)param; int *iter = localdata->valuelist; /** Hint: The localdata pointer also has fields num_values and sum. */ pthread_exit(NULL); }
![#include <pthread.h>
#include <stdio.h>
#include <stdlib.h>
#include <sys/types.h>
#define NUMTHREADS 2
struct thread_data {
int "valuelist;
int num_values;
int sum;
};
void *calculate_sum(void *);
int main(void) {
int values[] = {1, 3, 5, 7, 9, 2, 6, 7,
в, 10,
11, 12, 13, 14, 15, 40, 50, 68, 61, 62};
%3D
int sum - 0;
int *iter - values;
pthread_t tids[NUMTHREADS];
/* Calculate how much data each thread will work on */
size_t num_values;
num_values = sizeof (values) / sizeof (*values);
int num_values_per_thread = num_values / NUMTHREADS;
/** Standard C doesn't have variable length arrays. To allocate a dynamic
* array, calloc can be used. This is different than dynamically creating a
* list with multiple malloc calls.
*/
struct thread_data *d = calloc(NUMTHREADS, sizeof(struct thread_data));
for (int i - 0; i < NUMTHREADS; i++) {
/* Using array notation changes -> into . /
d[i].sum = 0;
d[1].num_values - num_values_per_thread;
d[i].valuelist - calloc(num_values_per_thread, sizeof(int));
for (int j = 0; j< num values_per_thread; j++) {
fprintf(stderr, "assigning Xd to thread %d \n", *iter, 1);
d[i].valuelist[j] - *iter;
iter++;
%3D
fprintf(stderr, "In");
}
for (int i - 0; i < NUMTHREADS; i++) {
pthread_create(&tids[i], NULL, calculate_sum, (void *)&d[i]);
}
for (int i - 0; i < NUMTHREADSs; i++) {
pthread_join(tids[i], NULL);
sum += d[i].sum;
}
printf("Sum is: %d\n", sum);
void *calculate sum(void *param) {
struct thread_data *localdata = (struct thread_data *)param;
int *iter - localdata->valuelist;
/** Hint: The localdata pointer also has fields num_values and sum. /
pthread_exit(NULL);](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fdca3868f-d639-48af-8a6a-e81b235ca722%2F62849401-7317-478f-b8c6-160e3289e6f0%2Fd4k6t0j_processed.jpeg&w=3840&q=75)

Step by step
Solved in 2 steps

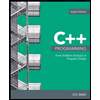
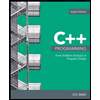