You are working in a doctors' office and have been tasked with creating an application to maintain Patient records for each doctor in the office. Create a class to maintain a Doctor. A doctor has a name that must be stored in the Doctor class. A doctor can only treat 3 patients but could treat fewer than 3. The information for each patient should be encapsulated in a Patient class and should include the patient's last name and up to 5 cholesterol readings for the patient. Note that less than 5 cholesterol readings may sometimes be stored. Your Doctor class should support operations to add a patient record to the end of his/her list of assigned patients (i.e., use a vector to store Patient objects in the Doctor class), and to list all patient records (name and associated cholesterol readings). Your Patient class should include operations to allow entry of the patient's last name and up to 5 cholesterol readings, and to return the name and cholesterol readings for that patient. You should write a main program that creates a single Doctor and presents a menu to users that allows them to select either Add (A), or List (L), or Quit (Q). Add should allow the user to enter a patient record (name and cholesterol readings) and add it to the end of the doctor's list of patients. List should list all patient records currently in the doctor's list. Remember that a patient record includes the patient's name as well as his/her cholesterol readings. You should be able to add and list repeatedly, until you select Q to quit. Use good coding style and principles for all code and input/output formatting. All data in a class must be private. Put each class declaration in its own header file and its implementation in a separate .cpp file. How to approach this lab: 1. In main(): a. Prompt the user to enter a name for a doctor. b. Create an object of the Doctor class, passing in the name of the doctor as an argument to the constructor of the Doctor class. c. Create a menu with “cout" statements. d. Create a switch statement that contains the cases that the user could enter. e. For the Add patients case, prompt the user to enter a name for the patient if the number of patients already existing is less than 3. 1. Using the Doctor object, call the function in the Doctor class to add a patient, passing in the name of the patient that the user entered. f. For the List Patients case, call the function that prints patient records, again by using the Doctor object to call the function in the Doctor class.
Can you please help me write this in C++ with this implemented with in it
Excutes without crashing |
Appropriate Internal Documentation |
Doctor class created |
Doctor.h |
Doctor.cpp |
Contains data for no more than 3 patients |
Patient class created |
Patient.h |
Patient.cpp |
Contains data for up to 5 cholesterol readings |
Menu |
Add: Successfully adds a patient record |
Successfully adds cholesterol readings |
List: Lists all the data |
Quit: Quits |
Loops until the user selects quit |
Style: |
Data members are correctly declared |
Member functions exist in the correct classes |
Overall style is appropriate (no globals unless constants, modularity, uses a |
Appropriate formatting of output |
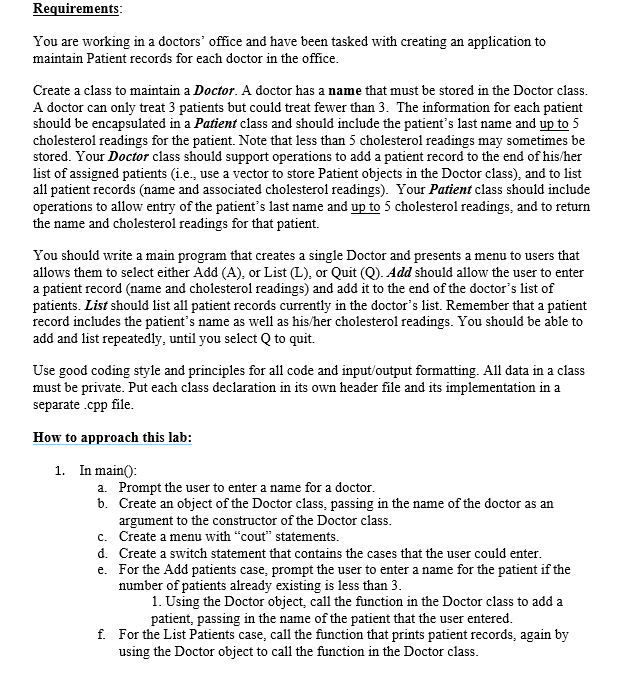
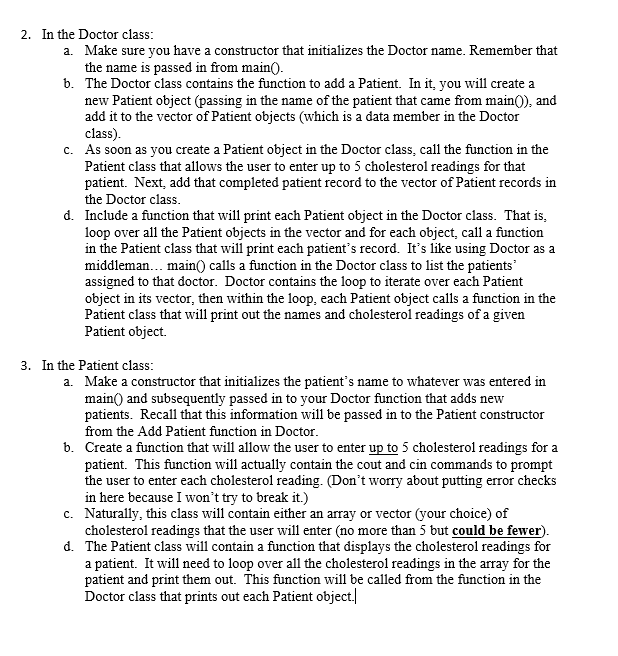

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

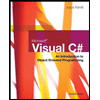
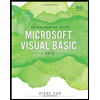
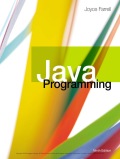
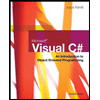
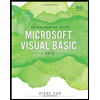
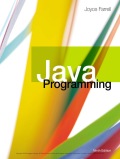
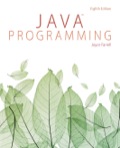