This is supposed to test your understanding of Java streams and lambda expressions. Specifically how to create a nonparallel and parallel stream. Intermediate operations, such as map, flatMap, and filter. The terminal operation collect. How to work with java lambda functions with these operations. And efficient programming. This assignment is not meant to be exhausting, in the ideal situation, you will only have to write 25 lines of code to complete this entire assignment. You are to fill in the code in the StreamsHomeWork.java file. You may find a description of what is to be performed for each function below or in that java file provided. Please keep in mind that all the functions have a timelimit in the milliseconds, the exact time for each function is documented below. Please fill in the functional code in the StreamsHomeWork.java file and submit the StreamsHomeWork.java file to Grader Than. Note: The last two functions of the assignment can be used to help you select winning words in the Ghost Project. The last function named "filterLosers" is not required. I added it as extra credit. If you can solve it with an algorithm that does not work in less than O(n^2) time then your Ghost application will be nearly unstoppable. But if your application takes O(n^2) time or longer your application will timeout and lose. Have Fun!
This is supposed to test your understanding of Java streams and lambda expressions. Specifically how to create a nonparallel and parallel stream. Intermediate operations, such as map, flatMap, and filter. The terminal operation collect. How to work with java lambda functions with these operations. And efficient programming. This assignment is not meant to be exhausting, in the ideal situation, you will only have to write 25 lines of code to complete this entire assignment. You are to fill in the code in the StreamsHomeWork.java file. You may find a description of what is to be performed for each function below or in that java file provided. Please keep in mind that all the functions have a timelimit in the milliseconds, the exact time for each function is documented below.
Please fill in the functional code in the StreamsHomeWork.java file and submit the StreamsHomeWork.java file to Grader Than.
Note: The last two functions of the assignment can be used to help you select winning words in the Ghost Project. The last function named "filterLosers" is not required. I added it as extra credit. If you can solve it with an
public static Stream getStream(Collection collection)
This function is given a collection. It will return a stream of the collection. This Function must take no longer than 500 milliseconds to return.
collection - a collection (List or a Set) of objects
returns - A stream of the collection
public static Stream getParallelStream(Collection collection)
This function is given a collection. It will return a parallel stream of the collection. This Function must take no longer than 500 milliseconds to return.
collection - a collection (List or a Set) of objects
return - A stream of the collection that is parallel
public static List<String> convertStreamToStrings(Stream<? extends Object> stream)
This function will convert a stream of objects into a list of strings. Each string in the list is the string representation of the object. Map each object in the stream to a string and collect it into a list. This Function must take no longer than 500 milliseconds to return.
stream - A stream of objects
return - A list of strings
public static Set<Integer> powerDivisibleNumbers(int power, int divisor, Stream<Integer> stream)
This function will filter out all the numbers in the stream that are divisible by the divisor number. Then raise the filtered number to the power number. Example: (n=filtered number) npower. And finally, collect all the numbers in a set. This Function must take no longer than 500 milliseconds to return.
power - A number that the numbers that the divisible numbers will be raised to.
divisor - A number that the numbers in the stream must be divisible by.
stream - A stream of integers.
return - A set of numbers
public static Set<Integer> flatFilteredMap(List<Integer> filterList, Stream<List<Integer>> stream)
This function is given a stream of lists containing integers. You must return a set of all of the numbers that are found in the streams sub-lists that are contained in the filter list. In other words, flatten the stream then filter the numbers based on if they are contained in the filter list. This Function must take no longer than 500 milliseconds to return.
filterList - A list of numbers
stream - A stream of lists that contain numbers
return - A set of numbers found in the stream sub-lists that are equal to at least one number in the Filter List.
public static Map<String, String> toMap(Stream<String> stream)
This function is given a stream of strings and it is your job to convert the strings to a Map. The map's key is the strings in the stream and the values are the strings in reverse order. This Function must take no longer than 500 milliseconds to return.
stream - A stream of strings
return - A map with key equal to the strings in the stream and values equal to strings that are the reverse of the keys
static List<String> findWinners(String pattern, int minLength, boolean even, Stream<String> stream)
This function will receive a stream of strings and it will return a sorted list of strings that meet all of the following criteria:
- They must contain the pattern
- The strings length must be equal to or greater than the min length number
- The strings length must be an even or odd number
This Function must take no longer 10000 milliseconds to return.
pattern - A pattern that all the strings in the list returned must contain
minLength - The length that all the strings in the list returned must be equal to or greater than
even - True if even false if odd. Denotes a condition that all the strings in the list returned must have a length that is even or odd.
stream - A stream of strings to be filtered
return - A list of strings that meets the criteria outlined above sorted by their length from small to large

Trending now
This is a popular solution!
Step by step
Solved in 5 steps

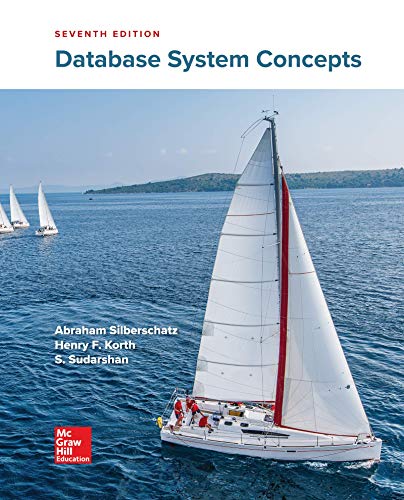
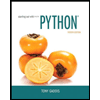
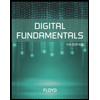
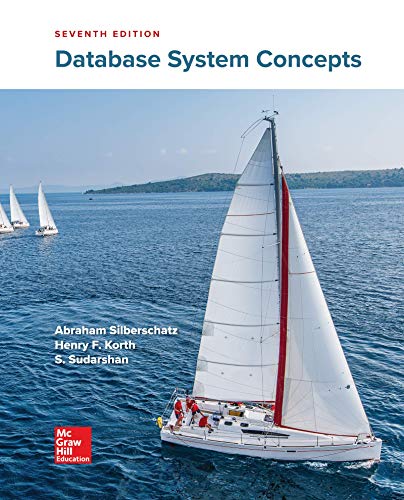
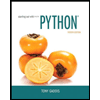
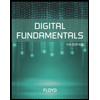
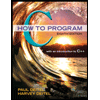
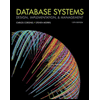
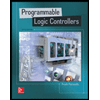