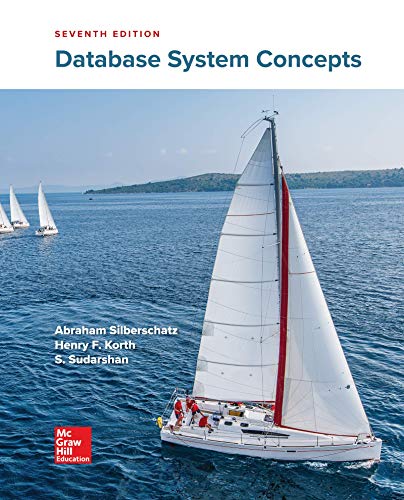
This
main() will call create() and then call retrieve()
create() will prompt the user to enter any number of course name / grade pairs and write them to a file named grades.txt. Pressing Enter with no input value on the course name will exit the loop, close the file, print "File was created and closed" and return the bool value True to the main()
retrieve() will open and read grades.txt. The function then will print the course names and scores as shown below. The average score should be calculated as well as a simple GPA and displayed to two decimal places and then return variable used for the GPA to the main() . See sample run below in which 4 course names were entered, but note that more or fewer courses could have been entered.
I need my grades.txt file to output course and grade in seperate lines (which I think I fixed) and work for the final output (not fixed). I am getting errors. I am not sure how to adjust my code. I attached a good example... Mine is not working, (attached example)
The expected output for the program is also attached.
Thank you for helping.
Here is my current code in Python
#Defining the function create()
def create():
#open the file
grades_file = open('grades.txt','w')
while True:
course_name = input("Enter course name or Enter to quit: ")
#Storing the values
if course_name == '':
print("File was created and closed")
break
grade = int(input("Enter grade (integer) achieved: "))
line = course_name+'\n'+str(grade)+'\n'
grades_file.write(line)
#close the file
grades_file.close()
return True
#Defining the retrieve function
def retrieve():
#Printing statement
print("\nHere are your grades:")
#Initializing the variables
sum_of_grades = 0
count = 0
gpa=0
#open file
grades_file = open('grades.txt','r')
for line in grades_file:
course,grade = line.strip()
#finding sum
sum_of_grades+=int(grade)
#finding the grade
if(int(grade)>=90):
gpa+=4.0
elif(int(grade)>=80):
gpa+=3.0
elif(int(grade)>=70):
gpa+=2.0
elif(int(grade)>=60):
gpa+=1.0
else:
gpa+=0.0
#count how many grades are in total
count+=1
gpa_cal = gpa/count
print("{} score is {}".format(course,grade))
#find the average grade
avg = sum_of_grades/count
print("Average grade among your courses is {:.2f}".format(avg),"Your GPA is {:.2f}".format(gpa_cal))
return avg
#Defining the main()
def main():
#Calling the methods
create()
GPA = retrieve()
main()
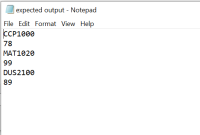
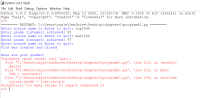

Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 3 images

- Create a brief code segment that moves the file pointer 50 bytes from the file's start. Assume the file is already open and BX holds the file handle.arrow_forwardRange for loop should be used even the block code needs to access index. Group of answer choices True Falsearrow_forwardMenu option A -- Determine Hours to StudyThe program will READ in data from a text file named StudyHours.txt. The user corrects any bad data. The program updates the information in StudyHours.txt file. For example if the file contains a letter grade of K which is not a possible letter grade. You will create and submit a text file with a minimum of 5 additional records from example below. The file is named StudyHours.txt and contains the following format: first line full namesecond line number of creditsthird line grade desired for each class Example format StudyHours.txt fileAaron RODgers12ATom brady9Kphilip RiversapplecJoe Theismann15B The program determines the total weekly study hours (for all classes)All data must be displayed in proper case such as Wendy Payne, i.e. no names should be in all lower case or all upper case or a mix such as wendy or PaYNe. Use a function to convert to proper case.The program displays the student’s name, number of credits, expected total number of…arrow_forward
- im usually using python Write a program named filemaker.py that will be used to store the first name and age of some friends in a text file named friends.txt. The program must use a while loop that prompts the user to enter the first name and age of each friend. Each of these entries should be written to its own line in the text file (2 lines of data per friend). The while loop should repeat until the user presses Enter (Return on a Mac) for the name. Then, the file should be closed and a message should be displayed. See Sample Output.SAMPLE OUTPUT Enter first name of friend or Enter to quit DennyEnter age (integer) of this friend 24Enter first name of friend or Enter to quit PennyEnter age (integer) of this friend 28Enter first name of friend or Enter to quit LennyEnter age (integer) of this friend 20Enter first name of friend or Enter to quit JennyEnter age (integer) of this friend 24Enter first name of friend or Enter to quit File was createdarrow_forwardWhat does the following python code do? f = open("sample.txt", "a") Choose all that apply. Select 3 correct answer(s) Question 15 options: opens a file called sample.txt for reading reads the file called sample.txt starting from the top writes "a" to the file called sample.txt opens a file called sample.txt for appending If the file called sample.txt exists, it writes at the bottom of the file closes a file called sample.txt if the file sample.txt exists, deletes everything in it if the file sample.txt does not exist, it creates the file and opens it for writingarrow_forwardC programarrow_forward
- Sample Run 2: Enter the symbol to draw the shapes: # Enter the width of the shape: 4 Enter the height of the shape: 6 #### ## ### ## Save the file as cla14.py by clicking on File on the menu bar, then click on "Save as .". Type in cla14.py as the file name in the save as dialog box. • Run your program: Hit function key to execute the program. Alternatively you can click on Run on the menu bar and select "Run Module". • If you have any errors, fix them, save the changes in the file window, and re-run the program ()arrow_forwardWhat does the following python code do? f = open("sample.txt", "w") Choose all that apply. Select 2 correct answer(s) Question 14 options: If the file called sample.txt exists, it writes at the bottom of the file writes "w" to the file called sample.txt closes a file called sample.txt opens a file called sample.txt for appending opens a file called sample.txt for reading reads the file called sample.txt starting from the top if the file sample.txt exists, deletes everything in it if the file sample.txt does not exist, it creates the file and opens it for writingarrow_forwardTwo of the menu options (#2 and #3) are unfinished, and you need to complete the code necessary to write to and read from a binary file. You will need to read through the code to find the functions that are called by these options, and then supply the missing code. Please help me get menu 2 and 3 to display properly.the .cpp and .h have the information:.cpp file: // Corporate Sales Data Outputusing namespace std; #include <iostream>#include <fstream>#include <stdlib.h>#include "HeaderClassActivity1.h" int main() { Sales qtrSales[NUM_QTRS]; while (true) { cout << "Select an option: " << endl; cout << "1. Input data" << endl; cout << "2. Write data to file" << endl; cout << "3. Read data from file" << endl; cout << "4. Display sales" << endl; cout << "99. End program" << endl; int menuItem; cin >> menuItem; if (menuItem ==…arrow_forward
- This is to be done using Visual Studio and the information input needs to be stored in an array of records.arrow_forwardSummary In this lab, you write a while loop that uses a sentinel value to control a loop in a Python program. You also write the statements that make up the body of the loop. Each theater patron enters a value from 0 to 4 indicating the number of stars that the patron awards to the Guide’s featured movie of the week. The program executes continuously until the theater manager enters a negative number to quit. Instructions Make sure the file MovieGuide.py is selected and open. Write thewhile loop using a sentinel value to control the loop, and also write the statements that make up the body of the loop. Execute the program by clicking the Run button at the bottom of the screen. Input the following as star ratings: 0, 3, 4, 4, 1, 1, 2, -1 must be written with this code: """ MovieGuide.py This program allows each theater patron to enter a value from 0 to 4 indicating the number of stars that the patron awards to the Guide's featured movie of the week. The program executes…arrow_forwardTurn the following code into a procedure. Pass the input file and the amount of PM 10 to the procedure. It will print the city name and the pollution values for all cities that have that much PM 10 pollution or more.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
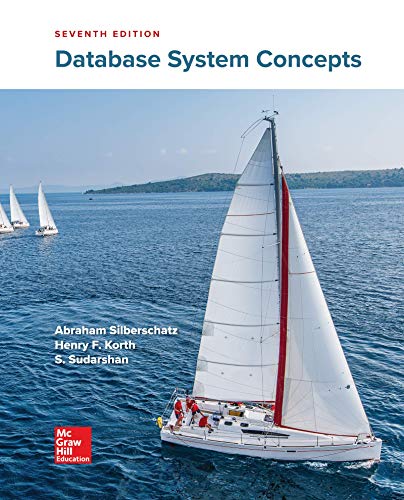
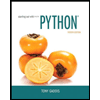
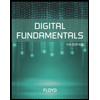
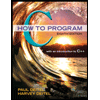
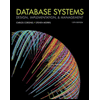
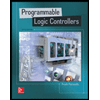