How to fix my error? assignment question This program will have three functions, the main() create() and retrieve(). main() will call create() and then call retrieve() create() will prompt the user to enter any number of course name / grade pairs and write them to a file named grades.txt. Pressing Enter with no input value on the course name will exit the loop, close the file, print "File was created and closed" and return the bool value True to the main() retrieve() will open and read grades.txt. The function then will print the course names and scores as shown below. The average score should be calculated as well as a simple GPA and displayed to two decimal places and then return variable used for the GPA to the main() . See sample run below in which 4 course names were entered, but note that more or fewer courses could have been entered. Tip: Simple GPA, In its easiest form, an A=4, B=3, C=2, D=1, F=0. For each class you have, you assign the correct number to the letter grade, add all of your grades together and divide by the number of classes you have taken. Score Letter GPA >= 90 A 4.0 >= 80 B 3.0 >= 70 C 2.0 >= 60 D 1.0 <60 F 0.0 here is what I coded so far def create(): myGrades_file = open('grades.txt','w') while True: my_courses = input("Enter course name or Enter to quit: ") if my_courses == '': print("File was created and closed") break my_grades = int(input("Enter grade (integer) achieved: ")) string = my_courses+','+" "+str(my_grades)+'\n' myGrades_file.write(string) myGrades_file.close() return True def retrieve(): print("\nHere are your grades:") grades_total = 0 count = 0 gpa=0 myGrades_file = open('grades.txt','r') for line in myGrades_file: mycourses, grades = line.strip().split(',') grades_total+=int(grades) if(int(grades)>=90): gpa+=4.0 elif(int(grades)>=80): gpa+=3.0 elif(int(grades)>=70): gpa+=2.0 elif(int(grades)>=60): gpa+=1.0 else: gpa+=0.0 count+=1 gpa_total = gpa/count print("{} score is{}".format(mycourses,grades)) average = grades_total/count print("Average grade among your courses is {:.2f}".format(average),"Your GPA is {:.2f}".format(gpa_total)) return average def main(): create() average = retrieve() main() This is the error that I am receiving Test 2 Expected data: ['CGS1070', '98'] Data in file: ['CGS1070, 98']Test 2 FailedE ====================================================================== ERROR: test_2_io_case_2 (__main__.programTestCase) ---------------------------------------------------------------------- Traceback (most recent call last): AssertionError: Lists differ: ['CGS1070, 98'] != ['CGS1070', '98'] First differing element 0: 'CGS1070, 98' 'CGS1070' Second list contains 1 additional elements. First extra element 1: '98' - ['CGS1070, 98'] + ['CGS1070', '98'] ? + + During handling of the above exception, another exception occurred: Traceback (most recent call last): ValueError: Contents of file != Expected
How to fix my error?
assignment question
This program will have three functions, the main() create() and retrieve().
main() will call create() and then call retrieve()
create() will prompt the user to enter any number of course name / grade pairs and write them to a file named grades.txt. Pressing Enter with no input value on the course name will exit the loop, close the file, print "File was created and closed" and return the bool value True to the main()
retrieve() will open and read grades.txt. The function then will print the course names and scores as shown below. The average score should be calculated as well as a simple GPA and displayed to two decimal places and then return variable used for the GPA to the main() . See sample run below in which 4 course names were entered, but note that more or fewer courses could have been entered.
Tip: Simple GPA, In its easiest form, an A=4, B=3, C=2, D=1, F=0. For each class you have, you assign the correct number to the letter grade, add all of your grades together and divide by the number of classes you have taken.
Score | Letter | GPA |
>= 90 | A | 4.0 |
>= 80 | B | 3.0 |
>= 70 | C | 2.0 |
>= 60 | D | 1.0 |
<60 | F | 0.0 |
here is what I coded so far
def create():
myGrades_file = open('grades.txt','w')
while True:
my_courses = input("Enter course name or Enter to quit: ")
if my_courses == '':
print("File was created and closed")
break
my_grades = int(input("Enter grade (integer) achieved: "))
string = my_courses+','+" "+str(my_grades)+'\n'
myGrades_file.write(string)
myGrades_file.close()
return True
def retrieve():
print("\nHere are your grades:")
grades_total = 0
count = 0
gpa=0
myGrades_file = open('grades.txt','r')
for line in myGrades_file:
mycourses, grades = line.strip().split(',')
grades_total+=int(grades)
if(int(grades)>=90):
gpa+=4.0
elif(int(grades)>=80):
gpa+=3.0
elif(int(grades)>=70):
gpa+=2.0
elif(int(grades)>=60):
gpa+=1.0
else:
gpa+=0.0
count+=1
gpa_total = gpa/count
print("{} score is{}".format(mycourses,grades))
average = grades_total/count
print("Average grade among your courses is {:.2f}".format(average),"Your GPA is {:.2f}".format(gpa_total))
return average
def main():
create()
average = retrieve()
main()
This is the error that I am receiving
Test 2 Expected data: ['CGS1070', '98'] Data in file: ['CGS1070, 98']Test 2 FailedE
======================================================================
ERROR: test_2_io_case_2 (__main__.programTestCase)
----------------------------------------------------------------------
Traceback (most recent call last):
AssertionError: Lists differ: ['CGS1070, 98'] != ['CGS1070', '98']
First differing element 0:
'CGS1070, 98'
'CGS1070'
Second list contains 1 additional elements.
First extra element 1:
'98'
- ['CGS1070, 98']
+ ['CGS1070', '98']
? + +
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
ValueError: Contents of file != Expected

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

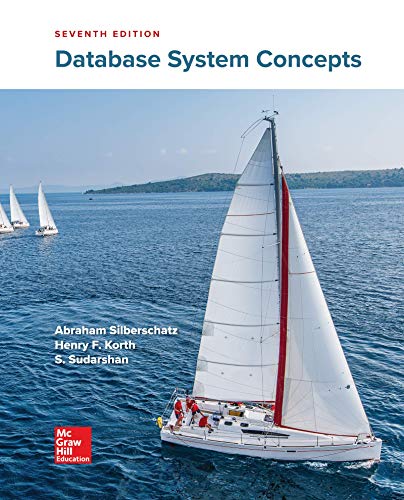
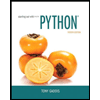
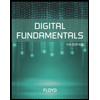
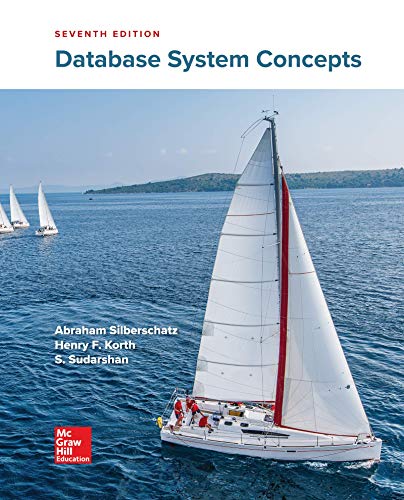
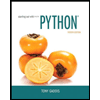
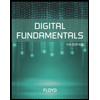
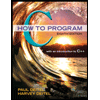
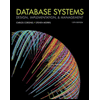
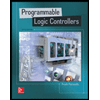