This question is related from my previous question, when I compile this code it gave me several errors. Here's the code with the instructions: import java.text.ParseException; import java.text.SimpleDateFormat; import java.time.LocalDate; import java.time.format.DateTimeFormatter; public class TestWedding{ private static SimpleDateFormat simpleDateFormat = new SimpleDateFormat("yyyy-MM-dd"); //Method to display Wedding details - Couple, Location and Wedding date public static void displayWedding(Wedding w){ System.out.println(""); System.out.println("=== Wedding Information ==="); Person p1 = w.getCouple().getFirstPerson(); Person p2 = w.getCouple().getSecondPerson(); System.out.println(p1.getFirstName() + "" + p2.getLastName() + "(born " + p1.getBirthDate().format(DateTimeFormatter.ISO_DATE)+")" + " weds " + p2.getFirstName()+ "" + p2.getLastName() + "(born " + p2.getBirthDate().format(DateTimeFormatter.ISO_DATE)+")"); System.out.println("ON"); System.out.println(simpleDateFormat.format(w.getWeddingDate())); System.out.println("AT"); System.out.println(w.getLocation()); } //Test method - to create Perspn, Couple and Wedding objects public static void main(String[]args)throws ParseException{ //Create Person objects Person p10 = new Person("John", "Howard", LocalDate.of(2000, 20, 1)); Person p11 = new Person("Mary", "Eliza", LocalDate.of(2002, 1, 2)); //Create Couple object with above data Couple c1 = new Couple(p10,p11); //Create Wedding Object Wedding w1 = new Wedding(simpleDateFormat.parse("2019-05-06"),c1,"CountrySide"); //Display Wedding details displayWedding(w1); Person p20 = new Person("James", "Howie", LocalDate.of(1999, 5, 1)); Person p21 = new Person("Jenny", "Hertz", LocalDate.of(2005, 4, 25)); Couple c2 = new Couple(p20,p21); Wedding w2 = new Wedding(simpleDateFormat.parse("2019-01-02"),c2,"Mary Islands"); displayWedding(w2); } } Create a class named Person that holds the following fields: two String objects for the person’s first and last name and a LocalDate object for the person’s birthdate. Create a class named Couple that contains two Person objects. Create a class named Wedding for a wedding planner that includes the date of the wedding, the names of the Couple being married, and a String for the location. Provide constructors for each class that accept parameters for each field, and provide get methods for each field. Then write a program that creates two Wedding objects and in turn passes each to a method that displays all the details. Save the files as Person.java, Couple.java, Wedding.java, and TestWedding.java. Please help, I have until Friday to submit this assignment.
This question is related from my previous question, when I compile this code it gave me several errors. Here's the code with the instructions:
import java.text.ParseException;
import java.text.SimpleDateFormat;
import java.time.LocalDate;
import java.time.format.DateTimeFormatter;
public class TestWedding{
private static SimpleDateFormat simpleDateFormat = new SimpleDateFormat("yyyy-MM-dd");
//Method to display Wedding details - Couple, Location and Wedding date
public static void displayWedding(Wedding w){
System.out.println("");
System.out.println("=== Wedding Information ===");
Person p1 = w.getCouple().getFirstPerson();
Person p2 = w.getCouple().getSecondPerson();
System.out.println(p1.getFirstName() + "" + p2.getLastName() + "(born " + p1.getBirthDate().format(DateTimeFormatter.ISO_DATE)+")"
+ " weds " + p2.getFirstName()+ "" + p2.getLastName() + "(born " + p2.getBirthDate().format(DateTimeFormatter.ISO_DATE)+")");
System.out.println("ON");
System.out.println(simpleDateFormat.format(w.getWeddingDate()));
System.out.println("AT");
System.out.println(w.getLocation());
}
//Test method - to create Perspn, Couple and Wedding objects
public static void main(String[]args)throws ParseException{
//Create Person objects
Person p10 = new Person("John", "Howard", LocalDate.of(2000, 20, 1));
Person p11 = new Person("Mary", "Eliza", LocalDate.of(2002, 1, 2));
//Create Couple object with above data
Couple c1 = new Couple(p10,p11);
//Create Wedding Object
Wedding w1 = new Wedding(simpleDateFormat.parse("2019-05-06"),c1,"CountrySide");
//Display Wedding details
displayWedding(w1);
Person p20 = new Person("James", "Howie", LocalDate.of(1999, 5, 1));
Person p21 = new Person("Jenny", "Hertz", LocalDate.of(2005, 4, 25));
Couple c2 = new Couple(p20,p21);
Wedding w2 = new Wedding(simpleDateFormat.parse("2019-01-02"),c2,"Mary Islands");
displayWedding(w2);
}
}
Create a class named Person that holds the following fields: two String objects
for the person’s first and last name and a LocalDate object for the person’s
birthdate.
Create a class named Couple that contains two Person objects.
Create a class named Wedding for a wedding planner that includes the date of the wedding,
the names of the Couple being married, and a String for the location.
Provide constructors for each class that accept parameters for each field, and provide
get methods for each field.
Then write a program that creates two Wedding objects and in turn passes each to a method that displays all the details.
Save the files as Person.java, Couple.java, Wedding.java, and TestWedding.java.
Please help, I have until Friday to submit this assignment.

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

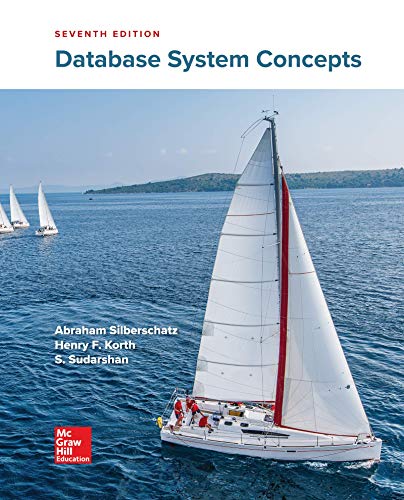
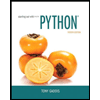
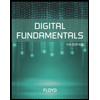
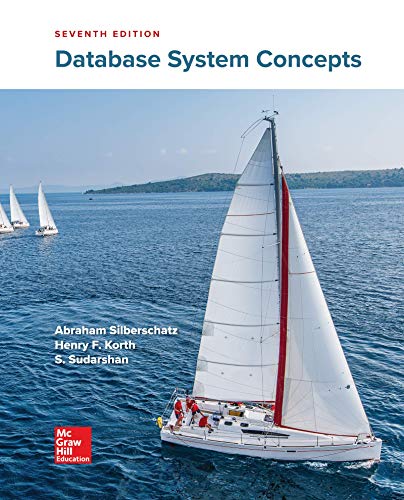
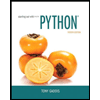
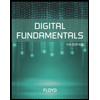
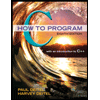
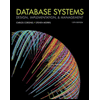
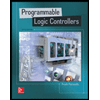