Tractors Modification of the Tractor project. Make a copy of your testTractor Package name it testLoader and make the following modifications and additions. Let us create a class hierarchy for tractors to use in a fleet management system. All of your classes should be in separate files within your project package. Name your package testLoader. Modify the base class named Tractor. The Tractor class will have a static attribute called Company. All tractors are owned by the same company. Create a class named Loader that inherits from Tractor. Loaders had a Bucket Size (1-5) the Bucket Size effects the RentalProfit by an additional $100-$500. You will need to Override the RentalProfit method to perform this calculation correctly. Create a fully parameterized constructor that uses a reference to super(). Override the toString to contain the base tractor information plus the Bucket Size option. Create a class called Harvester. Harvesters are must be rented for at least 20 days. Override the setter for. Rental days to reflect this. Harvesters may have a tow behind trailer option (1-3) that adds $1000-$3000 to the RentalProfit() calculation. Create a fully parameterized constructor that uses a reference to super(). Override the toString to contain the base tractor information plus the trailer option. In your test drivers main() method create a Tractor, Loader and a Harvester. Using the zero parameter contructor. Then use the set methods to set all attributes. Also create a Tractor, Loader and Harvester using the parameterized contructor. Set the company name to your name. Use the toString() methods to display the information for each tractor. Be sure to display the rentalProfit( calculation in your test driver. Create a zip file for your package and submit it for grading.
Tractors Modification of the Tractor project. Make a copy of your testTractor Package name it testLoader and make the following modifications and additions. Let us create a class hierarchy for tractors to use in a fleet management system. All of your classes should be in separate files within your project package. Name your package testLoader. Modify the base class named Tractor. The Tractor class will have a static attribute called Company. All tractors are owned by the same company. Create a class named Loader that inherits from Tractor. Loaders had a Bucket Size (1-5) the Bucket Size effects the RentalProfit by an additional $100-$500. You will need to Override the RentalProfit method to perform this calculation correctly. Create a fully parameterized constructor that uses a reference to super(). Override the toString to contain the base tractor information plus the Bucket Size option. Create a class called Harvester. Harvesters are must be rented for at least 20 days. Override the setter for. Rental days to reflect this. Harvesters may have a tow behind trailer option (1-3) that adds $1000-$3000 to the RentalProfit() calculation. Create a fully parameterized constructor that uses a reference to super(). Override the toString to contain the base tractor information plus the trailer option. In your test drivers main() method create a Tractor, Loader and a Harvester. Using the zero parameter contructor. Then use the set methods to set all attributes. Also create a Tractor, Loader and Harvester using the parameterized contructor. Set the company name to your name. Use the toString() methods to display the information for each tractor. Be sure to display the rentalProfit( calculation in your test driver. Create a zip file for your package and submit it for grading.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
The first question is a prerequisite to the second. Please answer the second question.
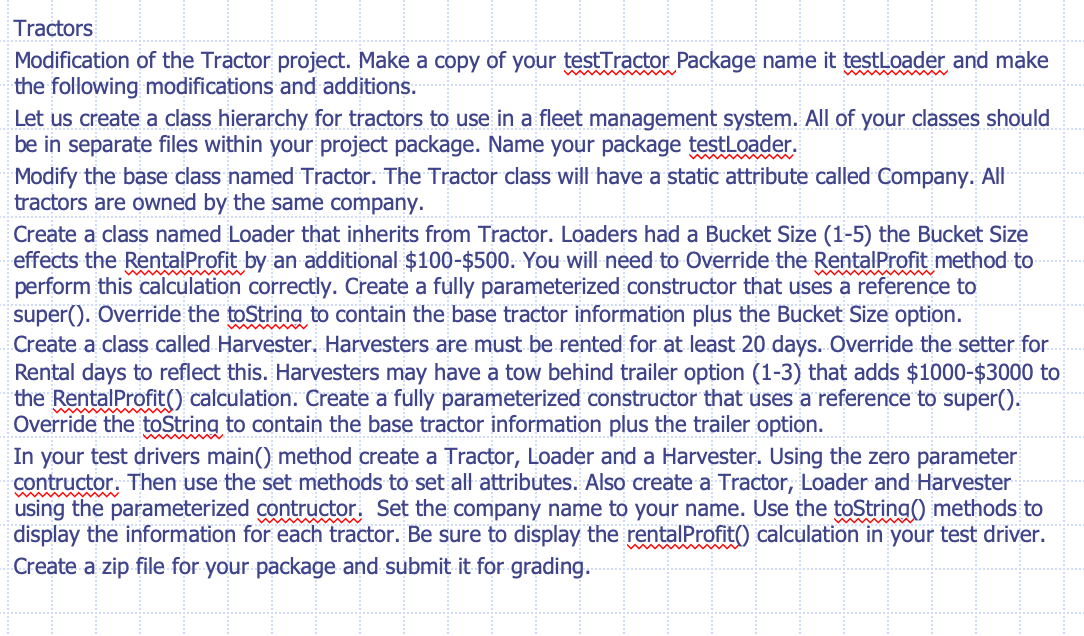
Transcribed Image Text:Tractors
Modification of the Tractor project. Maké a copy of your testTractor Package name it testloader and make
the following modifications and additions.
Let us create a class hierarchy for tractors to use in a fleet management system. All of your classes should
be in separate files within your project package. Name your package testLoader.
Modify the base class named Tractor. The Tractor class will have a static attribute called Company. All
tractors are owned by the same company.
Create a class named Loader that inherits from Tractor. Loaders had a Bucket Size (1-5) the Bucket Size
effects the RentalProfit by an additional $100-$500. You will need to Override the RentalProfit method to
perform this calculation correctly. Create a fully parameterized constructor that uses a reference to
super(). Override the toString to contain the base tractor information plus the Bucket Size option.
Create a class called Harvester. Harvesters are must be rented for at least 20 days. Override the setter for.
Rental days to reflect this. Harvesters may have a tow behind trailer option (1-3) that adds $1000-$3000 to
the RentalProfit() calculation. Create a fully parameterized constructor that uses a reference to super().
Override the toString to contain the base tractor information plus the trailer option.
In your test drivers main() method create a Tractor, Loader and a Harvester. Using the zero parameter
contructor. Then use the set methods to set all attributes. Also create a Tractor, Loader and Harvester
using the parameterized contructor, Set the company name to your name. Use the toString() methods to
display the information for each tractor. Be sure to display the rentalProfit() calculation in your test driver.
Create a zip file for your package and submit it for grading.
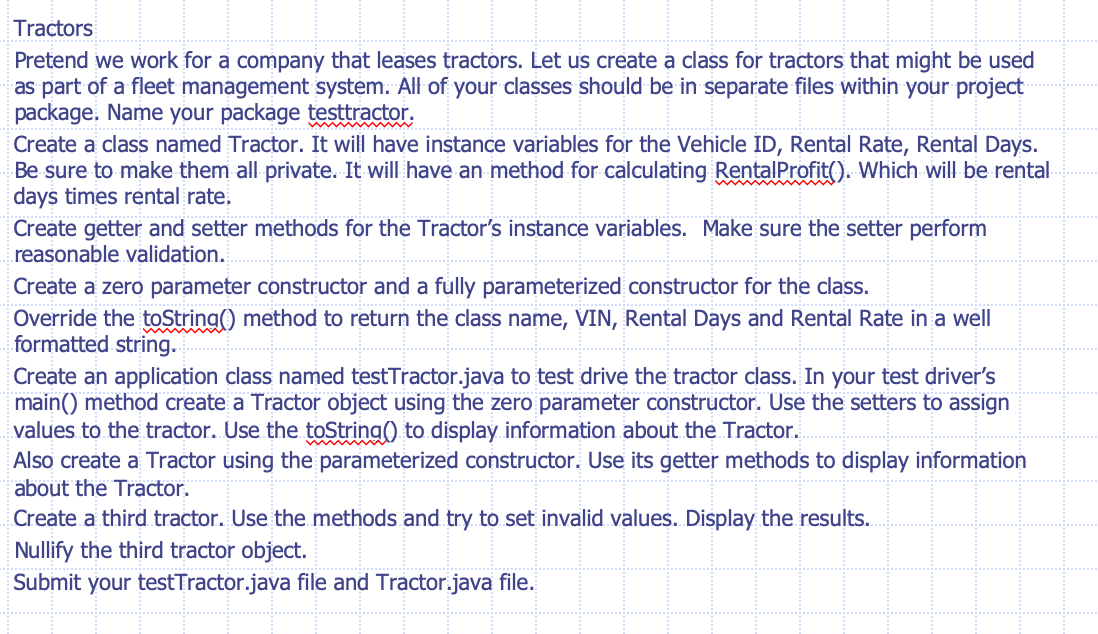
Transcribed Image Text:Tractors
Pretend we work for a company that leases tractors. Let us create a class for tractors that might be used
as part of a fleet management system. All of your classes should be in separate files within your project
package. Name your package testtractor.
Create a class named Tractor. It will have instance variables for the Vehicle ID, Rental Rate, Rental Days.
Be sure to make them all private. It will have an method for calculating RentalProfit(). Which will be rental
days times rental rate.
Create getter and setter methods for the Tractor's instance variables. Make sure the setter perform
reasonable validation.
Create a zero parameter constructor and a fully parameterized constructor for the class.
Override the toString() method to return the class name, VIN, Rental Days and Rental Rate in a well
formatted string.
Create an application class named testTractor.java to test drive the tractor class. In your test driver's
main() method create a Tractor object using the zero parameter constructor. Use the setters to assign
values to the tractor. Use the toString() to display. information about the Tractor.
Also create a Tractor using the parameterized constructor. Use its getter methods to display information
about the Tractor.
Create a third.tractor. Use the.methods and try to set invalid values. Display the results.
Nullify the third tractor object.
Submit your testTractor.java file and Tractor.java file.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 4 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
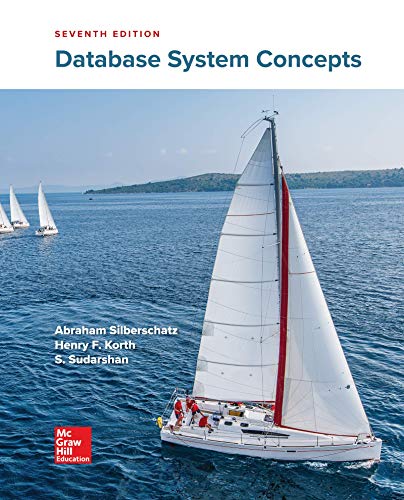
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
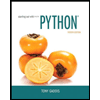
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
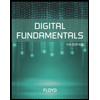
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
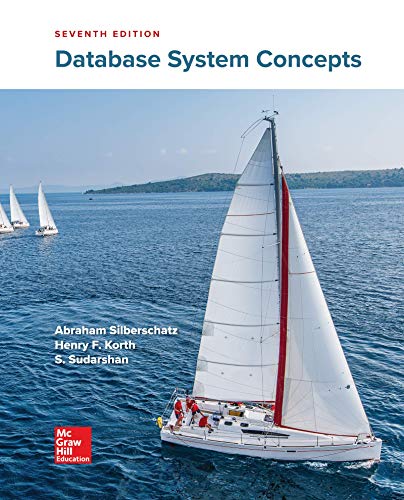
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
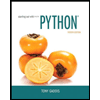
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
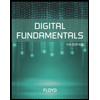
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
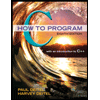
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
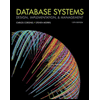
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
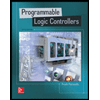
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education