turn this c code into assembly language in optimized and unoptimized mode. /* Project 2 */ /* GCC */ /* gcc -Wa,-adhln -g -masm=intel -m32 "Project 2.c" > "Project 2-g.asm" */ /* gcc -Wa,-adhln -O -masm=intel -m32 "Project 2.c" > "Project 2-o.asm" */ #include #define NOINLINE __attribute__ ((noinline)) static NOINLINE int function1(int x, int y) { int i; int sum; int values[10]; sum = 0; for (i = 0; i < 10; i++) { values[i] = 10 * i + x * y; sum += values[i]; } return (sum); } static int NOINLINE function2(int *values, int valuesLen) { int i; int sum; sum = 0; for (i = 0; i < valuesLen; i++) { sum += values[i]; } return (sum); } static NOINLINE int function3(int x) { int y; y = x / 10; return (y); } static NOINLINE int function4(int a, int b, int c, int d) { int r; if (a > b) r = a; else if (a > c) r = 2 * a; else if (a > d) r = 3 * a; else r = -1; return (r); } int main(int argc, char **argv) { int i; int j; int k; int values[10]; i = 1; j = 2; k = function1(i, j); printf("function1: i = %d, j = %d, k = %d\n", i, j, k); for (i = 0; i < 10; i++) { values[i] = i; } k = function2(values, 10); printf("function2: k = %d\n", k); k = function3(100); printf("function3: k = %d\n", k); k = function4(1, 2, 3, 4); printf("function4: k = %d\n", k); return (0); }
turn this c code into assembly language in optimized and unoptimized mode.
/* Project 2 */
/* GCC */
/* gcc -Wa,-adhln -g -masm=intel -m32 "Project 2.c" > "Project 2-g.asm" */
/* gcc -Wa,-adhln -O -masm=intel -m32 "Project 2.c" > "Project 2-o.asm" */
#include <stdio.h>
#define NOINLINE __attribute__ ((noinline))
static NOINLINE int function1(int x, int y)
{
int i;
int sum;
int values[10];
sum = 0;
for (i = 0; i < 10; i++) {
values[i] = 10 * i + x * y;
sum += values[i];
}
return (sum);
}
static int NOINLINE function2(int *values, int valuesLen)
{
int i;
int sum;
sum = 0;
for (i = 0; i < valuesLen; i++) {
sum += values[i];
}
return (sum);
}
static NOINLINE int function3(int x)
{
int y;
y = x / 10;
return (y);
}
static NOINLINE int function4(int a, int b, int c, int d)
{
int r;
if (a > b)
r = a;
else if (a > c)
r = 2 * a;
else if (a > d)
r = 3 * a;
else
r = -1;
return (r);
}
int main(int argc, char **argv)
{
int i;
int j;
int k;
int values[10];
i = 1;
j = 2;
k = function1(i, j);
printf("function1: i = %d, j = %d, k = %d\n", i, j, k);
for (i = 0; i < 10; i++) {
values[i] = i;
}
k = function2(values, 10);
printf("function2: k = %d\n", k);
k = function3(100);
printf("function3: k = %d\n", k);
k = function4(1, 2, 3, 4);
printf("function4: k = %d\n", k);
return (0);
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

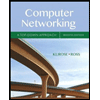
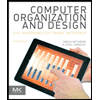
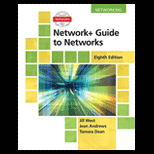
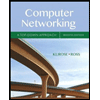
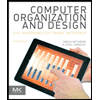
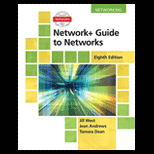
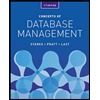
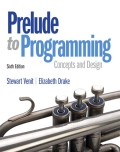
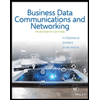