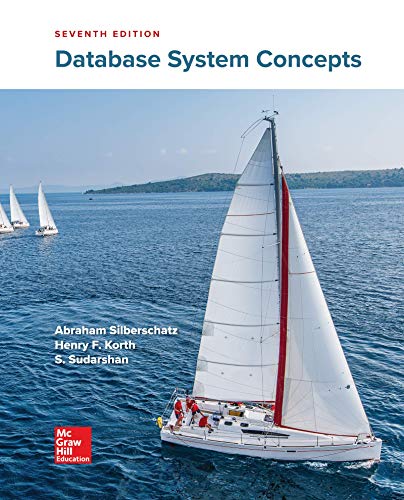
Complete the code and make it run sucessful by fixing errors
//MainValidatorA3
public class MainA3 {
public static void main(String[] args) {
System.out.println("Welcome to the Validation Tester application");
// Int Test
System.out.println("Int Test");
ValidatorNumeric intValidator = new ValidatorNumeric("Enter an integer between -100 and 100: ", -100, 100);
int num = intValidator.getIntWithinRange();
System.out.println("You entered: " + num + "\n");
// Double Test
System.out.println("Double Test");
ValidatorNumeric doubleValidator = new ValidatorNumeric("Enter a double value: ");
double dbl = doubleValidator.getDoubleWithinRange();
System.out.println("You entered: " + dbl + "\n");
// Required String Test
System.out.println("Required String Test:");
ValidatorString stringValidator = new ValidatorString("Enter a required string: ");
String requiredString = stringValidator.getRequiredString();
System.out.println("\nYou entered: " + requiredString + "\n");
// String Choice Test
System.out.println("String Choice Test");
ValidatorString choiceValidator = new ValidatorString("Select one (x/y): ", "x", "y");
String choice = choiceValidator.getChoiceString();
System.out.println("You entered: " + choice);
System.out.println("\nAll Done!");
}
}
//ValidatorNumeric
import java.util.Scanner;
class ValidatorNumeric {
private String prompt;
private int minInt, maxInt;
private double minDouble, maxDouble;
public ValidatorNumeric() {}
public ValidatorNumeric(String prompt, int min, int max) {
this.prompt = prompt;
this.minInt = min;
this.maxInt = max;
}
public ValidatorNumeric(String prompt, double min, double max) {
this.prompt = prompt;
this.minDouble = min;
this.maxDouble = max;
}
public int getInt() {
Scanner scanner = new Scanner(System.in);
System.out.print(prompt);
while (!scanner.hasNextInt()) {
System.out.println("Error! Invalid integer value. Try again.");
System.out.print(prompt);
scanner.next();
}
return scanner.nextInt();
}
public int getIntWithinRange() {
int num;
do {
num = getInt();
if (num < minInt) {
System.out.println("Error! Number must be greater than " + (minInt - 1));
} else if (num > maxInt) {
System.out.println("Error! Number must be less than " + (maxInt + 1));
}
} while (num < minInt || num > maxInt);
return num;
}
public double getDouble() {
Scanner scanner = new Scanner(System.in);
System.out.print(prompt);
while (!scanner.hasNextDouble()) {
System.out.println("Error! Invalid decimal value. Try again.");
System.out.print(prompt);
scanner.next();
}
return scanner.nextDouble();
}
public double getDoubleWithinRange() {
double num;
do {
num = getDouble();
if (num < minDouble) {
System.out.println("Error! Number must be greater than " + minDouble);
} else if (num > maxDouble) {
System.out.println("Error! Number must be less than " + maxDouble);
}
} while (num < minDouble || num > maxDouble);
return num;
}
}
Validator.Java
// Validator.java
import java.util.Scanner;
public interface Validator {
String getRequiredString();
String getChoiceString();
}
![ValidatorString.java X Validator.java X
Source
1
2
3
4
History
ValidatorNumeric.java X
mainValidatorA3.java x
88580
// ValidatorString.java
import java.util.Scanner;
public class ValidatorString implements Validator {
8
9
10
11
12
13
-
14
15
16
17
}
19
20
21
25
}
26
18
30
31
35
36
9=22±2927282232223223322-239327744
private Scanner scanner;
private String prompt;
private String[] choices;
public ValidatorString(String prompt) {
this.scanner = new Scanner (source: System.in);
this.prompt = prompt;
public ValidatorString(String prompt, String... choices) {
this.scanner = new Scanner (source: System.in);
this.prompt = prompt;
this.choices = choices;
public String getRequiredString() {
String input;
do {
System.out.print (s: prompt);
input = scanner.nextLine().trim();
while (input.isEmpty());
return input;
public String getChoiceString() {
String input;
boolean validChoice;
do {
System.out.print (s: prompt);
input = scanner.nextLine().trim();
validChoice = false;
for (String choice: choices) {
if (input.equalsIgnoreCase (anotherString: choice)) {
validChoice = true;
break;
}
}
40
41
45
}
46
}
if (!validChoice) {
}
System.out.println(x: "Invalid choice. Please try again.");
while (!validChoice);
return input;](https://content.bartleby.com/qna-images/question/a136d107-3f41-4dc0-bcbb-0f1a73f02f84/d6362fab-d7cc-4fad-91da-fc8676e1609d/pxsrfue_thumbnail.png)
to generate a solution
a solution
- Q1. amount the same, y, n, why? public class CheckingAct { private String actNum; private String nameOnAct; private int balance; . . . . public void processDeposit( int amount ) { balance = balance + amount ; } // modified toString() method public String toString() { return "Account: " + actNum + "\tName: " + nameOnAct + "\tBalance: " + amount ; } } Q2. amount the same, y, n, why? public class CheckingAct { private String actNum; private String nameOnAct; private int balance; . . . . public void processDeposit( int amount ) { // scope of amount starts here balance = balance + amount ; // scope of amount ends here } public void processCheck( int amount ) { // scope of amount starts here int charge; incrementUse(); if ( balance < 100000 ) charge = 15; else charge = 0; balance = balance - amount - charge ; // scope of amount ends here }…arrow_forwardDiscussion: chapter 4 Pick a method of debugging/error catching and explain how it works and give a real world example of how it works.arrow_forwardpublic static void printIt(int value) { if(value 0) { } System.out.println("Play a paladin"); System.out.println("Play a white mage"); }else { } }else if (value < 15) { System.out.println("Play a black mage"); System.out.println("Play a monk"); } else { public static void main(String[] args) { printit (5); printit (10); printit (15); Play a black mage Play a white mage (nothing/they are all printed) Play a paladin Play a monkarrow_forward
- Fix all logic and syntax errors. Results Circle statistics area is 498.75924968391547 diameter is 25.2 // Some circle statistics public class DebugFour2 { public static void main(String args[]) { double radius = 12.6; System.out.println("Circle statistics"); double area = java.Math.PI * radius * radius; System.out.println("area is " + area); double diameter = 2 - radius; System.out.println("diameter is + diameter); } }arrow_forwardTASK 5. Methods Overloading. Review Methods, Implement the following code, test it with different input, make sure it runs without errors.arrow_forwardusing System; class Program{ // Define PrintBoard method public static void PrintBoard() { Console.WriteLine(" X | | "); Console.WriteLine(" | | "); Console.WriteLine(" | O | "); Console.WriteLine(); } public static void Main(string[] args) { PrintBoard(); // Call PrintBoard method PrintBoard(); // Call it again PrintBoard(); // And again! }} This program is really simple and basic to print a tic tac toe board for the user to eventually play the computer in C#. How can it be modified a little bit to print the board and have each tile labeled with a number to identify the space? I'm a beginner and would like to take this step by step in modifying the code. Thank you!arrow_forward
- int stop = 6; int num =6; int count=0; for(int i = stop; i >0; i-=2) { num += i; count++; } System.out.println("num = "+ num); System.out.println("count = "+ count); } }arrow_forwardComplete the convert() method that casts the parameter from a double to an integer and returns the result.Note that the main() method prints out the returned value of the convert() method. Ex: If the double value is 19.9, then the output is: 19 Ex: If the double value is 3.1, then the output is: 3 code: public class LabProgram { public static int convert(double d){ /* Type your code here */ } public static void main(String[] args) { System.out.println(convert(19.9)); System.out.println(convert(3.1)); }}arrow_forwardCharge Account Validation Using Java programming Create a class with a method that accepts a charge account number as its argument. The method should determine whether the number is valid by comparing it to the following list of valid charge account numbers:5658845 4520125 7895122 8777541 8451277 13028508080152 4562555 5552012 5050552 7825877 12502551005231 6545231 3852085 7576651 7881200 4581002These numbers should be stored in an array. Use a sequential search to locate the number passed as an argument. If the number is in the array, the method should return true, indicating the number is valid. If the number is not in the array, the method should return false, indicating the number is invalid.Write a program that tests the class by asking the user to enter a charge account number. The program should display a message indicating whether the number is valid or invalid.arrow_forward
- Complete the convert() method that casts the parameter from a double to an integer and returns the res Note that the main() method prints out the returned value of the convert() method. Ex: If the double value is 19.9, then the output is: 19 Ex: If the double value is 3.1, then the output is: 3 512334.3517088.qx3zqy7 LAB ACTIVITY 1 public class LabProgram { INM + ∞ 2 25.20.1: LAB: Write convert() method to cast double to int 3 public static int convert (double d) { /* Type your code here */ 4 5 6 7 } 8 public static void main(String[] args) { 9 System.out.println (convert (19.9)); 10 11 12} } System.out.println(convert(3.1)); LabProgram.javaarrow_forwardusing System;public static class Lab3_2{public static void Main(){// declare the variables and constantsconst double FLAT = 1.25;double weight;double cost, pricePerKg = 0;// Input the weightConsole.Write("Enter a positive weight of the package => ");weight = Convert.ToDouble(Console.ReadLine());// Determine the cost per kilogramif (weight <= 0)Console.WriteLine("*** Invalid weight");else if (weight < 1)pricePerKg = 0.25;else if (weight <= 3.5)pricePerKg = 0.5;else (weight > 3.5)pricePerKg = 1.0;// Compute the cost to send the packageif (weight > 0)cost = weight * pricePerKg + FLAT;elsecost = 0;// Output the resultsConsole.WriteLine("The cost to send the package is {0:C}",cost);Console.ReadLine();}} Remove the error from the given program and run it for input weight 2.5.arrow_forwardclass Lab4Q1P3 { public static void main(String args[]) { int max = 10;while (max < 10) {System.out.println("count down: " + max);max--;}}} Do you know what is wrong with the program? I know that there is error on the 7th line but I don't know what to fix.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
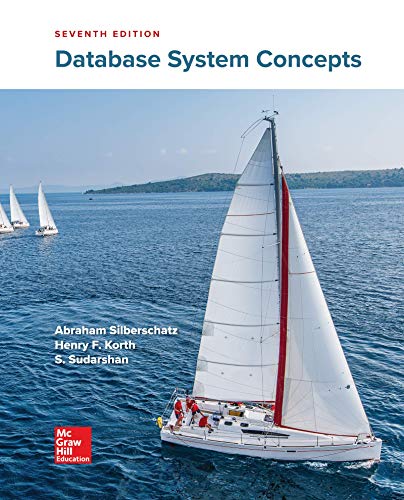
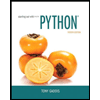
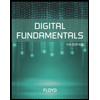
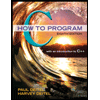
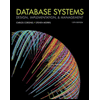
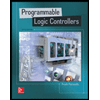