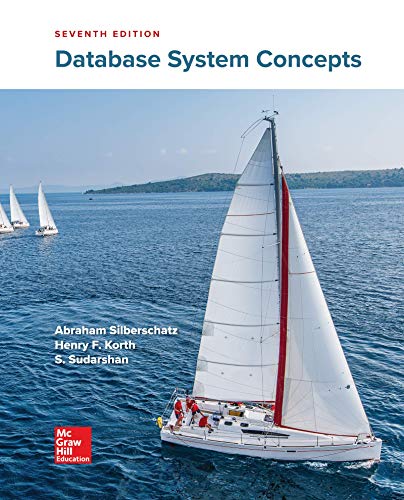
Concept explainers
JAVA
Modify this program below so it is a Filesorting.java program so it passes all the test cases when I upload it to Hypergrade. I have provided the failed test cases a screenshot.
import java.io.BufferedReader;
import java.io.FileReader;
import java.io.IOException;
import java.util.ArrayList;
import java.util.Arrays;
public class Main {
public static void main(String[] args) {
try {
BufferedReader reader = new BufferedReader(new FileReader(getFileName()));
String line;
while ((line = reader.readLine()) != null) {
processAndPrintSortedLine(line);
}
reader.close();
} catch (IOException e) {
System.out.println("Error reading the file: " + e.getMessage());
}
}
private static String getFileName() {
String fileName;
java.util.Scanner scanner = new java.util.Scanner(System.in);
do {
System.out.println("Please enter the file name or type QUIT to exit:");
fileName = scanner.nextLine();
if (fileName.equalsIgnoreCase("QUIT")) {
System.exit(0);
}
if (!fileExists(fileName)) {
System.out.println("File " + fileName + " is not found. Please re-enter the file name.");
}
} while (!fileExists(fileName));
return fileName;
}
private static boolean fileExists(String fileName) {
try (BufferedReader reader = new BufferedReader(new FileReader(fileName))) {
return true;
} catch (IOException e) {
return false;
}
}
private static void processAndPrintSortedLine(String line) {
String[] tokens = line.split(",");
ArrayList<Integer> numbers = new ArrayList<>();
for (String token : tokens) {
numbers.add(Integer.parseInt(token));
}
// Use any sorting method from Sort.java
// For example: Sort.insertionSort(numbers.toArray(new Integer[0]));
// Arrays.sort(numbers.toArray(new Integer[0]));
Arrays.sort(numbers.toArray(new Integer[0])); // Using insertion sort for demonstration
// Convert ArrayList<Integer> to CharSequence[]
CharSequence[] charSequences = new CharSequence[numbers.size()];
for (int i = 0; i < numbers.size(); i++) {
charSequences[i] = numbers.get(i).toString();
}
// Print the sorted numbers on the console
System.out.println(String.join(",", charSequences));
}
}
input2x2.csv
-67,-11
-27,-70
input1.csv
10
input10x10.csv
56,-19,-21,-51,45,96,-46
-27,29,-58,85,8,71,34
50,51,40,50,100,-82,-87
-47,-24,-27,-32,-25,46,88
-47,95,-41,-75,85,-16,43
-78,0,94,-77,-69,78,-25
-80,-31,-95,82,-86,-32,-22
68,-52,-4,-68,10,-14,-89
26,33,-59,-51,-48,-34,-52
-47,-24,80,16,80,-66,-42
input0.csv
Test Case 1
input1.csvENTER
10\n
Test Case 2
input2x2.csvENTER
-67,-11\n
-70,-27\n
Test Case 3
input10x10.csvENTER
-51,-46,-21,-19,45,56,96\n
-58,-27,8,29,34,71,85\n
-87,-82,40,50,50,51,100\n
-47,-32,-27,-25,-24,46,88\n
-75,-47,-41,-16,43,85,95\n
-78,-77,-69,-25,0,78,94\n
-95,-86,-80,-32,-31,-22,82\n
-89,-68,-52,-14,-4,10,68\n
-59,-52,-51,-48,-34,26,33\n
-66,-47,-42,-24,16,80,80\n
Test Case 4
input0.csvENTER
File input0.csv is empty.\n
Test Case 5
input2.csvENTER
File input2.csv is not found.\n
Please re-enter the file name or type QUIT to exit:\n
input1.csvENTER
10\n
Test Case 6
quitENTER
Test Case 7
input2.csvENTER
File input2.csv is not found.\n
Please re-enter the file name or type QUIT to exit:\n
QUITENTER
![Test Case 5 Failed Show what's missing
Error: Main method not found in class Sort, please define the main method as: \n
public static void main(String[] args)\n
or a JavaFX application class must extend javafx.ap.….. OUTPUT TOO LONG
Test Case 6 Failed Show what's missing
Error: Main method not found in class Sort, please define t... OUTPUT TOO LONG
Test Case 7 Failed Show what's missing
Error: Main method not found in class Sort, please define the main method as: \n
public static void main(String[] args)\n
or a JavaFX application class must extend... OUTPUT TOO LONG](https://content.bartleby.com/qna-images/question/c3fe77f3-9d48-40aa-bb9c-abf8aedf62a2/95f74152-72c7-481b-a396-c00dfc0ef698/vi9yimt_thumbnail.png)
![Test Case 1 Failed Show what's missing
Error: Main method not found in class Sort, please define the main me... OUTPUT TOO LONG
Test Case 2 Failed Show what's missing
Error: Main method not found in class Sort, please define the main method as:\n
publ... OUTPUT TOO LONG
Test Case 3 Failed Show what's missing
Error: Main method not found in class Sort, please define the main method as: \n
public static void main(String[] args) \n
or a JavaFX application class must extend javafx.application. Application\n
Test Case 4 Failed Show what's missing D
Error: Main method not found in class Sort, please define the main method as: \n
public static... OUTPUT TOO LONG](https://content.bartleby.com/qna-images/question/c3fe77f3-9d48-40aa-bb9c-abf8aedf62a2/95f74152-72c7-481b-a396-c00dfc0ef698/o7ji0k2_thumbnail.png)

Step by stepSolved in 4 steps with 12 images

- I need this in JAVAarrow_forwardIm trying ro read a csv file and store it into a 2d array but im getting an error when I run my java code. my csv file contains 69 lines of data Below is my code: import java.util.Scanner; import java.util.Arrays; import java.util.Random; import java.io.File; import java.io.FileNotFoundException; import java.io.FilenameFilter; public class CompLab2 { public static String [][] getEarthquakeDatabase (String Filename) { //will read the csv file and convert it to a string 2-d array String [][] Fileinfo = new String [69][22]; int counter = 0; File file = new File(Filename); try { Scanner scnr = new Scanner(file); scnr.nextLine(); //skips the label in the first row of the file while (scnr.hasNextLine()) { // this while loop will count the number of values in the usgs file counter += 1; // increases by one each time a line is read scnr.nextLine(); } while…arrow_forwardI need help with a Java program described below: import java.util.Scanner;import java.io.FileInputStream;import java.io.IOException; public class LabProgram { public static void main(String[] args) throws IOException { Scanner scnr = new Scanner(System.in); /* Type your code here. */ }}arrow_forward
- Please help how do I make the code show on the black screen instead of a txt.file. By the way I have already created a txt.file in this java compiler called Data.txtarrow_forwardin java Write code that prints: firstNumber … 2 1Print a newline after each number. Ex: If the input is: 3 the output is: 3 2 1 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 import java.util.Scanner; public class ForLoops { publicstaticvoidmain (String [] args) { intfirstNumber; inti; Scannerinput=newScanner(System.in); firstNumber=input.nextInt(); for () { System.out.println(i); } } }arrow_forwardJava Netbeansarrow_forward
- JAVA PROGRAM PLEASE FIX AND MODIFY THIS JAVA SO WHEN I UPLOAD IT TO HYPERGRADE IT PASSES ALL TEST CASSES PLEASES. RIGHT NOW IT SAYS 0 OUT 3 PASSED. THE PICTURES THAT I PROVIDED PROOF THAT WHEN I UPLOAD IT TO HYPERGRADES IT FAILS TEST CASSES. THANK YOU. import java.io.BufferedReader;import java.io.FileReader;import java.io.IOException;import java.util.InputMismatchException;import java.util.Locale;import java.util.Scanner;public class FileTotalAndAverage { public static void main(String[] args) { Scanner scanner = new Scanner(System.in); String fileName; do { System.out.print("Please enter the file name: "); fileName = scanner.nextLine(); try { // Read numbers from the file double[] numbers = readNumbersFromFile(fileName); if (numbers != null) { // Calculate total and average double total = calculateTotal(numbers);…arrow_forwardimport java.util.Scanner; public class CircleAndSphereWhileLoop{ public static final double MAX_RADIUS = 500.0; public static void main(String[] args) { Scanner in = new Scanner(System.in); // Step 2: Read a double value as radius using prompt // "Enter the radius (between 0.0 and 500.0, exclusive): " // Step 3: While the input radius is not in the ragne (0.0, 500.0) // Display a message on one line (ssuming input value -1) // "The input number -1.00 is out of range." // Read a double value as radius using the same promt double circumference = 2 * Math.PI * radius; double area = Math.PI * radius * radius; double surfaceArea = 4 * Math.PI * Math.pow(radius, 2); double volume = (4 / 3.0) * Math.PI * Math.pow(radius, 3); // Step 4: Display the radius, circle circumference, circle area, // sphere surface area, and…arrow_forwardJava Proram ASAP Please improve and adjust the program which is down below with the futher moddifications because it does not pass the test cases in Hypergrade. Please remove /n from the program and for test case 4 after this line: Please re-enter the file name or type QUIT to exit:\n quitENTER there needs to be nothing. import java.io.*;import java.util.Scanner;public class ConvertText { public static void main(String[] args) throws Exception { Scanner sc = new Scanner(System.in); System.out.println("Please enter the file name or type QUIT to exit:"); while (true) { String input = sc.next(); if (input.compareTo("QUIT") == 0) { break; } else { String filePath = new File("").getAbsolutePath(); filePath = filePath.concat("/"); filePath = filePath.concat(input); File file = new File(filePath); if (file.exists() && !file.isDirectory()) {…arrow_forward
- StringFun.java import java.util.Scanner; // Needed for the Scanner class 2 3 /** Add a class comment and @tags 4 5 */ 6 7 public class StringFun { /** * @param args not used 8 9 10 11 12 public static void main(String[] args) { Scanner in = new Scanner(System.in); System.out.print("Please enter your first name: "); 13 14 15 16 17 18 System.out.print("Please enter your last name: "); 19 20 21 //Output the welcome message with name 22 23 24 //Output the length of the name 25 26 27 //Output the username 28 29 30 //Output the initials 31 32 33 //Find and output the first name with switched characters 34 //All Done! } } 35 36 37arrow_forwardimport java.io.BufferedReader;import java.io.File;import java.io.FileReader;import java.util.Scanner;public class romeoJuliet {public static void main(String[]args) throws Exception{Scanner keyboard=new Scanner(System.in);System.out.println("Enter a filename");String fN=keyboard.nextLine();int wLC=0, lC=0;String sW= keyboard.nextLine();File file=new File(fN);String [] wordSent=null;FileReader fO=new FileReader(fN);BufferedReader buff= new BufferedReader(fO);String sent;while((sent=buff.readLine())!=null){if(sent.contains(sW)){wLC++;}lC++;}System.out.println(fN+" has "+lC+" lines");System.out.println("Enter some text");System.out.println(wLC+" line(s) contain \""+sW+"\"");fO.close();}} Need to adjust code so that it reads all of the words that it was tasked to find. for some reason it isn't finding all of the word locations in a text. for example the first question is to find "the" in romeo and juliet which should have 1137, but it's only picking up 1032?arrow_forwardI need this in JAVAarrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
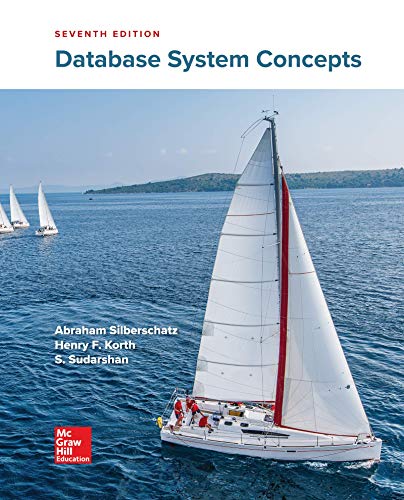
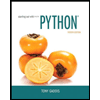
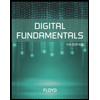
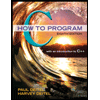
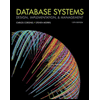
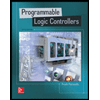