When you borrow money to buy a house, a car, or for some other purpose, you repay the loan by making periodic payments over a certain period of time. Of course, the lending company will charge interest on the loan. Every periodic payment consists of the interest on the loan and the payment toward the principal amount. To be specific, suppose that you borrow $1,000 at an interest rate of 7.2% per year and the payments are monthly. Suppose that your monthly payment is $25. Now, the interest is 7.2% per year and the payments are monthly, so the interest rate per month is 7.2/12 = 0.6%. The first month’s interest on $1,000 is 1000 X 0.006 = 6. Because the payment is $25 and the interest for the first month is $6, the payment toward the principal amount is 25 - 6 = 19. This means after making the first payment, the loan amount is 1,000 - 19 = 981. For the second payment, the interest is calculated on $981. So the interest for the second month is 981 X 0.006 = 5.886, that is, approximately $5.89. This implies that the payment toward the principal is 25 - 5.89 = 19.11 and the remaining balance after the second payment is 981 - 19.11 = 961.89. This process is repeated until the loan is paid. Instructions Write a program that accepts as input: The loan amount The interest rate per year The monthly payment. (Enter the interest rate as a percentage. For example, if the interest rate is 7.2% per year, then enter 7.2.) The program then outputs the number of months it would take to repay the loan. (Note that if the monthly payment is less than the first month’s interest, then after each payment, the loan amount will increase.) In this case, the program must warn the borrower that the Monthly payment is too low. The loan cannot be repaid.
Control structures
Control structures are block of statements that analyze the value of variables and determine the flow of execution based on those values. When a program is running, the CPU executes the code line by line. After sometime, the program reaches the point where it has to make a decision on whether it has to go to another part of the code or repeat execution of certain part of the code. These results affect the flow of the program's code and these are called control structures.
Switch Statement
The switch statement is a key feature that is used by the programmers a lot in the world of programming and coding, as well as in information technology in general. The switch statement is a selection control mechanism that allows the variable value to change the order of the individual statements in the software execution via search.

// iostream in C++ is the standard library which implement stream based input/output capabilities
#include <iostream>
using namespace std;
//main function
int main(){
//declare the required variables
double loanborrow;
double monthlyamount;
double rate,rpm;
double interest=0;
double principal;
//prompt the user to enter the respective info as asked
cout<<"Enter the loan borrowed: $";
cin>>loanborrow;
cout<<"Enter the rate of interest per year: ";
cin>>rate;
cout<<"Enter monthly pay: $";
cin>>monthlyamount;
//calculate the rate per month
rpm=(rate/12.0)/100;
//declare the count variable
//that inimates the number of years
int count=0;
//execute a loop until the loan if ful filled
while(loanborrow>=0){
//condition to check whether the monthly
//pay is less than interest
if(monthlyamount>interest){
//if the condition is true,
//then execute the following statements
interest=loanborrow*rpm;
principal=monthlyamount-interest;
loanborrow=loanborrow-principal;
count++;
}
//if the condition is false
//display the below information
else{
cout<<"Monthly pay is too low than the interest.The loan cannot be recovered."<<endl;
}
}
cout<<"In "<<count<<" months the loan will be repaid."<<endl;
return 0;
}
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

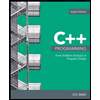
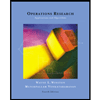
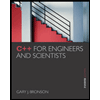
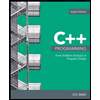
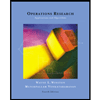
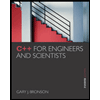