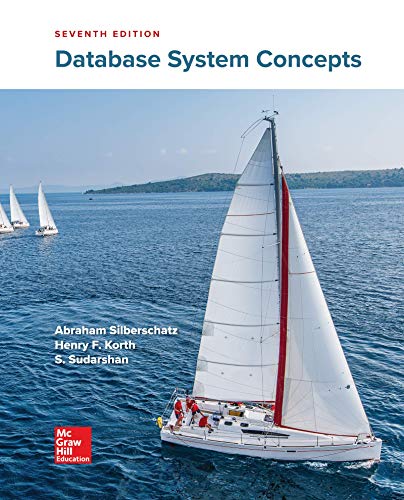
Concept explainers
This program is based in Java, so the response has to be java.
Movie Collection Program
Create a program that keeps track of your movie collection.
Your driver file is movieCollection
You will use it to create 4 movie objects.
Your Movie class should have a default constructor that does not receive any values and sets all information to default values.
You should also have an overload constructor method that has all of the data passed into it.
The following information is what each movie object should have:
Title
Genre
Year of release
Director.
You should have mutator methods that let you set all pieces of information individually.
You should have accessor methods that allow you to get each piece of information individually.
You should have a toString method that prints out all of the information about a movie at one time. Each piece of information should be on its own line.
In the movieCollection file
- Movie one should be created using the default constructor.
- Movies 2-4 should be created using the overloaded method, passing in all of the parameters.
- Print out just the title of movie 2
- Print out just the director for movie 3
- Print out just the genre for movie 4
- Use the mutator methods to add all of the information to movie 1.
- Lastly use the toString method to print out the information for all 4 movies.

Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 1 images

- QUESTION 7 Final data/code constructs are only inheritable once. O True O False QUESTION 8 You can include code in an interface by using the keywordarrow_forwardScenario: A company likes to have a simple system to keep track of all the employees. You must create an object-oriented solution. It must be a menu-driven program for the user to use the system through the console. The following classes are needed for this object-oriented database. 1) Create a class called Employee that has the following stored properties: Employee empID: Integer (Auto Generated) empFirstName: String empLastName: String empAnnualSalary : Integer empResidence: Residence ** Class Employee should have set/get properties, constructor and have following methods: toString() method that returns the above information as a String 2) Create a class called Residence which can be aggregated into the class Employee Residence streetName: String cityName: String zipCode: String province: String country: String ** Class Residence should have set/get properties, constructor and following method: toString() method that returns the above information as a String. System Menu: Summary of…arrow_forwardStockReader Class • This class will contain the method necessary to read and parse the .csv file of stock information. • This class shall contain a default constructor with no parameters. The constructor should be made private. . This is to prevent the class from being instantiated since the class will only contain static methods. • This class shall contain no data fields. • This class shall have a method called readStockData: . This method shall be a public, static, method. . This method shall return a StockList object once all data has been processed. . This method will take a File object as a parameter. This File object should link to the stock market data in your files folder created previously. . This method must validate that the given File object is a .csv file. If it is not, then this method shall throw an IllegalArgumentException. . This method shall read the File object and process all of the stock data into TeslaStock objects and store each object in a StockList. NOTE: The…arrow_forward
- A class object can encapsulate more than one [answer].arrow_forwardWhen a class uses dynamically allocated objects for its members and it does not have a copy assignment operator what of the following could happen (mark all that apply) There could be runtime errors There could be a double frees (double delete errors) There could be memory leaks Nothing happens the compiler provides the correct constructor There could be compile time errorsarrow_forwardWrite a program that will contain an array of person class objects. The program should include two classes: 1) One class will be the person class. 2) The second class will be the team class, which contain the main method and the array or array list. The person class should include the following data fields; Name Phone number Birth Date Jersey Number Be sure to include get and set methods for each data field.The team class should contain the data fields for the team, such as: Team name Coach name Conference name The program should include the following functionality. Add person objects to the array; Find a specific person object in the array; (find a person using any data field you choose such as name or jersey number) Output the contents of the array, including all data fields of each person object. (display roster)arrow_forward
- A field has access that is somewhere between public and private. static final Opackage Oprotectedarrow_forwardclass Student: def __init__(self, id, fn, ln, dob, m='undefined'): self.id = id self.firstName = fn self.lastName = ln self.dateOfBirth = dob self.Major = m def set_id(self, newid): #This is known as setter self.id = newid def get_id(self): #This is known as a getter return self.id def set_fn(self, newfirstName): self.fn = newfirstName def get_fn(self): return self.fn def set_ln(self, newlastName): self.ln = newlastName def get_ln(self): return self.ln def set_dob(self, newdob): self.dob = newdob def get_dob(self): return self.dob def set_m(self, newMajor): self.m = newMajor def get_m(self): return self.m def print_student_info(self): print(f'{self.id} {self.firstName} {self.lastName} {self.dateOfBirth} {self.Major}')all_students = []id=100user_input = int(input("How many students: "))for x in range(user_input): firstName = input('Enter…arrow_forwardCar Class Project The car classwill havethe following attributes: •year: an integer that holds the car's model year •model: a string that holds the make of the car •make: a string that holds the model of the car •speed: an integer that holds the car's current speed Your class should contain thefollowing: A docstring that briefly describes the class and lists the attributes. The docstring will serve as the documentation for your class. •A constructor (__init__ method) that takes the car's year model and make as optional arguments. The constructor will set the value of the speed attribute to 0.•An __str__ method that returns the car's year model and makein a string. •To test your class, create acar object and use the print function to verify that the constructor and __str__ methodsare working correctly. •An accessor method which returns the value stored in the speed instance variable. Call this method getSpeed(). •A modifier method called accelerate() which adds 5 to the speed variable…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
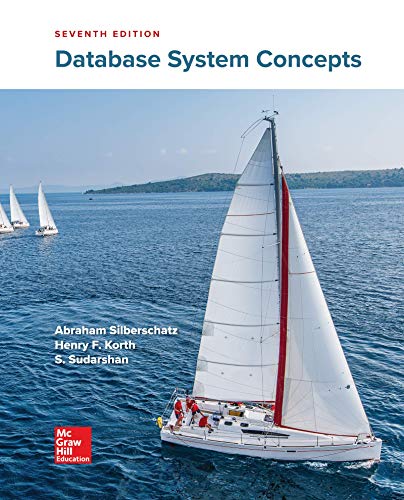
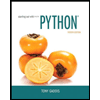
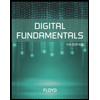
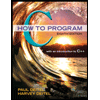
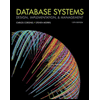
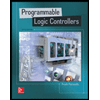