You will create a new version of the restaurant ordering program you created below in which the menu and pricing information is stored in a text file. Assume the name of the text file is menu.txt. You will create this file with the menu information using the following format for each line in the file: menu-item-name,price
You will create a new version of the restaurant ordering program you created below in which the menu and pricing information is stored in a text file.
Assume the name of the text file is menu.txt. You will create this file with the menu information using the following format for each line in the file:
menu-item-name,price
Also, we are going to allow any number of menu items, not just 10. (Note: after reading the menu information into your program, use the len() function to determine the size of the lists, and use this value for validations and for loops, when needed).
Place the following instructions at the beginning of the main() function.
menu_file = open('menu.txt', 'r')
menu_data = menu.readlines()
menu_file.close()
These instructions do the following:
- menu_file = open('menu.txt', 'r') : opens the file menu.txt for reading (the second parameter, r), and stores a reference in the variable menu_file
- menu_data = menu.readlines() : reads the contents of the file as a list of strings. Each item in the list menu_data is a line from the file menu.txt
- menu_file.close() : closes the file.
After these instructions, use the list menu_data (with a loop, and the help of the split() function) to initialize the lists menu_items and menu_prices in your program.
Since the data in menu.txt may contain more or less than 10 items, use len(menu_items) to determine the number of items in the menu for input validation, and for loops, if needed. The program should not require any further modifications.
def Menu(menu_items,menu_prices):
print("Menu Card")
count = 1
for i,j in zip(menu_items,menu_prices):
print("{}. {} price is ${:.2f}".format(count,i,j))
count+=1
def printReceipt(ordered_items,ordered_quantity,menu_prices,menu_items):
subtotal = 0
print("{:<15} {:<10} {:<7} {:<7}".format("Items","Quantity","Rate","Total"))
for i,j in zip(ordered_items,ordered_quantity):
subtotal += j*(menu_prices[i])
print("{:<15} {:<10} {:5.2f} {:5.2f}".format(menu_items[i],j,menu_prices[i],j*menu_prices[i]))
tax = 0.085*subtotal
tip = 0.15*subtotal
grand = subtotal+tax+tip
print("\n------Reciept------")
print("\nSubtotal: ", "$",subtotal)
print("Sales tax : ${:.2f}".format(tax))
print("Grand total : ","$", grand,)
print("Suggested tip : ${:.2f}".format(tip))
def userInputCalc(menu_items,menu_prics,ordered_items,ordered_quantity):
continueLoop = 'y'
while continueLoop=='y':
Menu(menu_items,menu_prices)
DishNumber = int(input("\nEnter numbers between 1 and 10:"))
if DishNumber<1 or DishNumber>10:
print("Invalid Input")
continue
print("{} is {}$".format(menu_items[DishNumber-1],menu_prices[DishNumber-1]))
quantity = int(input("Enter Quantity: "))
if quantity < 1:
print("Invalid Input")
continue
ordered_items.append(DishNumber-1)
ordered_quantity.append(quantity)
continueLoop = input("\nEnter y to Continue or n to quit:")
printReceipt(ordered_items,ordered_quantity,menu_prices,menu_items)
menu_items = ["Cajun Calamari","Shrimp Cocktail","Spicy Garlic Mussels","Crispy Ahi","Spinach Artichoke","Steamed Clams","Chips and Dip","Beer Battered Ribs",
"St.Louis Ribs","Mozzarella Sticks"]
menu_prices = [9,9,9,10.5,8,10,6.5,6,8,7]
ordered_items = []
ordered_quantity = []
userInputCalc(menu_items,menu_prices,ordered_items,ordered_quantity)

Step by step
Solved in 3 steps with 1 images

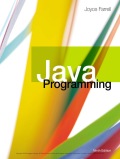
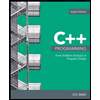
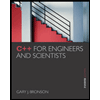
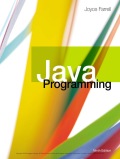
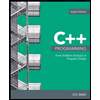
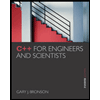
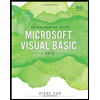