You will implement a comparable interface within Frult objects. Pretend that all fruit costs a toonie, no matter the size. To get more for your money, you will want to buy the bigger fruit. Details Input Input for this problem will be a fruit name, followed by a colour, and then the weight. Input has been handled for you in POD.java. Processing & Output Compare the weight of the object with the weight of the Frult object taken as input. The value you return from the compareTo() method will be as follows: • If the weight of the fruits is equal, you will return 0. If the current fruit object is greater, return 1. • Otherwise (i.e. the other fruit object is greater), you will return -1. This will allow us to sort the fruit objects by weight. This sort is handled in the main method of POD.java'. All output from that method is handled for you.
Java. Refer to attachment.
import java.util.*;
public class PoD
{
public static void main( String [] args ) {
Scanner in = new Scanner( System.in );
FruitBasket fruitBasket = new FruitBasket();
while(in.hasNextLine())
{
String line = in.nextLine();
String[] fruitDetails = line.split(" ");
Fruit nextFruit = new Fruit(fruitDetails[0],fruitDetails[1], Double.parseDouble(fruitDetails[2]));
fruitBasket.addFruit(nextFruit);
}
System.out.println("--- BEFORE SORT ---");
System.out.println(fruitBasket);
Collections.sort(fruitBasket.basket);
System.out.println("--- AFTER SORT ---");
System.out.println(fruitBasket);
in.close();
System.out.print("END OF OUTPUT");
}
}
import java.util.*;
public class FruitBasket
{
//attributes
protected ArrayList<Fruit> basket = new ArrayList<Fruit>();
//constructor
public FruitBasket(){}
//Setters
public void addFruit(Fruit fruitToAdd)
{
this.basket.add(fruitToAdd);
}
public String toString()
{
String basketContents = "FRUIT BASKET:\n";
for (Fruit fruit: basket)
{
basketContents += fruit.toString()+"\n";
}
return basketContents;
}
}
import java.util.*;
public class Fruit implements Comparable<Fruit>
{
//attributes
protected String name = null;
protected String colour = null;
protected double weight = 0;
//constructor
public Fruit(String name, String colour, double weight)
{
this.name = name;
this.colour = colour;
this.weight = weight;
}
//Getters
public String getName(){return name;}
public String getColour(){return colour;}
public double getWeight(){return weight;}
//Setters
public void setName(String name){this.name = name;}
public void setColour(String colour){this.colour = colour;}
public void setWeight(double weight){this.weight = weight;}
public String toString()
{
String fruitDetails = name+" (colour: "+colour+", weight: "+weight+")";
return fruitDetails;
}
// Write the compareTo method here!
}


Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

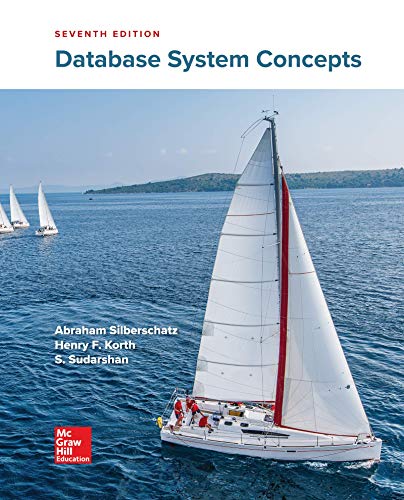
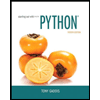
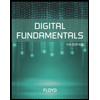
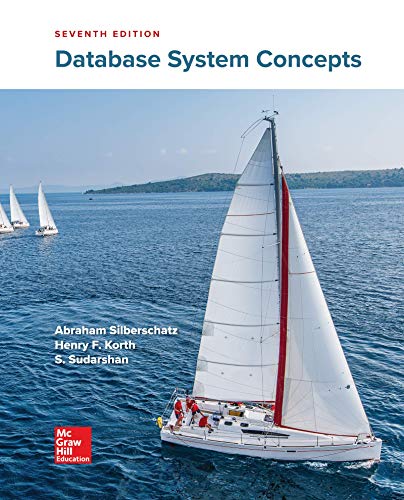
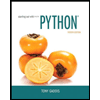
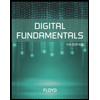
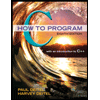
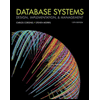
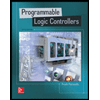