Change the program from the previous example to take command line arguments instead of using the Scanner.
import java.util.Scanner;
public class main
{
public static void main(String[] args)
{
Scanner sc = new Scanner(System.in):
int year, day, weekday;
String month;
String outday = "";
System.out.printf("Enter the month%n");
month = sc.nextLine();
System.out.printf("Enter the day%n");
month = sc.nextInt();
weekay = find_day(month, day);
outday = out_weekday(weekday);
System.out.printf("The day is: %s%n", outday);
}
}
Change the program from the previous example to take command line arguments instead of using the Scanner. For example, running the program like this:
> java OutDays March 14
will output the weekday string (which is Thursday) on the console for March 14, 2019).
public static void main(String[] args)
{
int year, day, weekday;
String month;
String outday = "";
// #### your code here for accessing the command line arguments
weekday = find_day(month, day);
outday = out_weekday(weekday);
System.out.printf("The day is: %s%n", outday);
}

Step by step
Solved in 2 steps

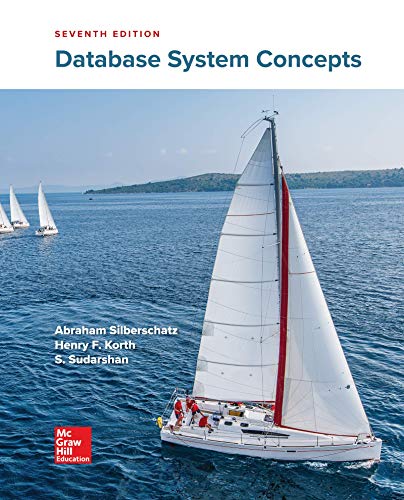
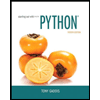
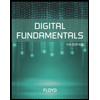
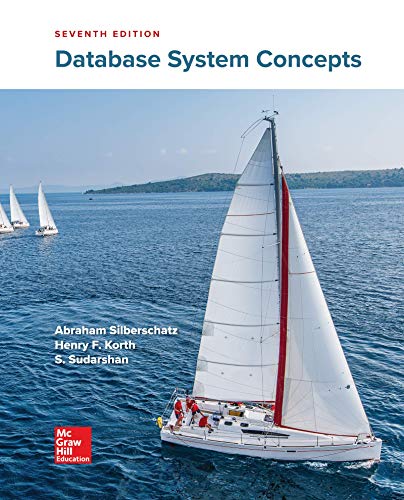
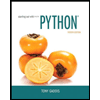
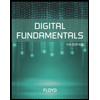
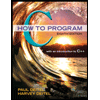
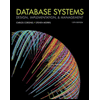
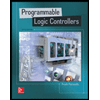