import java.util.Scanner; public class Product{ private Long barcode; private String name; private double cost; public Product(Long bc, String e, double c){ barcode=bc; this.name=e; cost=c; } public Long getBarcode(){ return barcode; } public void setName(String e){ this.name = e; } public String getName(){ return name; } public void setCost(double c){ cost = c; } public double getCost(){ return cost; } public String toString(){ return barcode + " : " + name + " - " + cost; } public boolean equals(Object o ){ if(o instanceof Product){ Product p = (Product) o; if(barcode.compareTo(p.getBarcode())==0) return true; } return false; } } The Market class we have to create has an array Product type and a name (String) so we have to provide the constructor of this class, who accepts the name of the market and the number of products containing so public Market(String name, int arraycapacity) then we also have to Provide the public boolean exists method (Product p) which indicates if the product in question is found in the market or not. • Offer the public void addProduct method (Product p) that adds a product to the market if the product does not exist and has a place in the market. • Provide the public void product method with Expensive () that displays the most expensive product in market. • Offer the public int nrProducts method (double pricing) that returns the number of products that have a lower price (are cheaper) than the price value accepted in the parameter. • Offer the public void method delete Products (double priced) that deletes priced products greater than the accepted price value in the parameter Note: You must deal with the empty spaces left over from the deletion. • Provide the main () method where an instance of the Market class with options can be created storage of 50 products, and add some Products to the market. • Create a Product type object and display (display) on the console, if this product exists in the market and if it does not exist add to the market. • Print (display) the data of the most expensive product • Print (display) the number of products that are cheaper than 15.30 Euro.
import java.util.Scanner;
public class Product{
private Long barcode;
private String name;
private double cost;
public Product(Long bc, String e, double c){
barcode=bc;
this.name=e;
cost=c;
}
public Long getBarcode(){
return barcode;
}
public void setName(String e){
this.name = e;
}
public String getName(){
return name;
}
public void setCost(double c){
cost = c;
}
public double getCost(){
return cost;
}
public String toString(){
return barcode + " : " + name
+ " - " + cost;
}
public boolean equals(Object o ){
if(o instanceof Product){
Product p = (Product) o;
if(barcode.compareTo(p.getBarcode())==0)
return true;
}
return false;
}
}
The Market class we have to create has an array Product type and a name (String) so we have to provide the constructor of this class, who accepts the name of the market and the number of products
containing so public Market(String name, int arraycapacity) then we also have to Provide the public boolean exists method (Product p) which indicates if the product in question is found
in the market or not.
• Offer the public void addProduct method (Product p) that adds a product to the market
if the product does not exist and has a place in the market.
• Provide the public void product method with Expensive () that displays the most expensive product in
market.
• Offer the public int nrProducts method (double pricing) that returns the number of products that
have a lower price (are cheaper) than the price value accepted in the parameter.
• Offer the public void method delete Products (double priced) that deletes priced products
greater than the accepted price value in the parameter
Note: You must deal with the empty spaces left over from the deletion.
• Provide the main () method where an instance of the Market class with options can be created
storage of 50 products, and add some Products to the market.
• Create a Product type object and display (display) on the console, if this product
exists in the market and if it does not exist add to the market.
• Print (display) the data of the most expensive product
• Print (display) the number of products that are cheaper than 15.30 Euro.

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

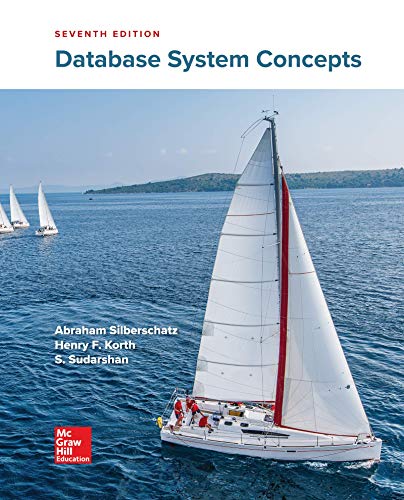
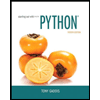
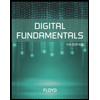
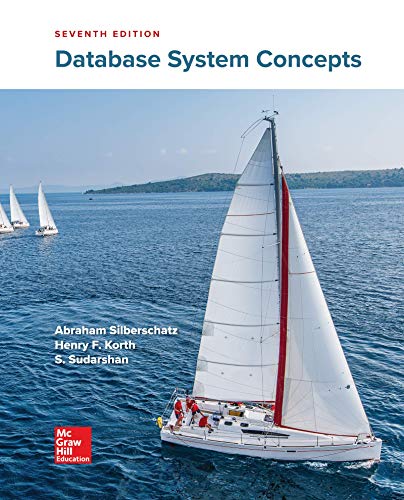
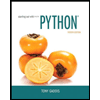
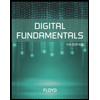
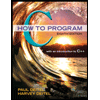
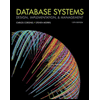
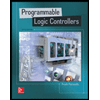